mirror of
https://github.com/drewcassidy/KSP-Conformal-Decals.git
synced 2024-09-01 18:23:54 +00:00
Merge branch 'develop'
This commit is contained in:
commit
bb0829c0a7
8
.gitignore
vendored
8
.gitignore
vendored
@ -2,15 +2,19 @@
|
||||
Assets/*
|
||||
!Assets/Shaders/
|
||||
!Assets/Textures/
|
||||
!Assets/Scripts/
|
||||
!Assets/UI/
|
||||
!Assets/ConformalDecals/
|
||||
KSP/
|
||||
Library/
|
||||
Logs/
|
||||
Packages/
|
||||
ProjectSettings/
|
||||
Temp/
|
||||
|
||||
# Unity Assetbundle Manifest Files
|
||||
GameData/ConformalDecals/Resources/Resources
|
||||
GameData/ConformalDecals/Resources/Resources.manifest
|
||||
GameData/ConformalDecals/Resources/conformaldecals.shab.manifest
|
||||
GameData/ConformalDecals/Resources/*.manifest
|
||||
|
||||
# Unity Project Files
|
||||
PartTools.cfg
|
||||
|
36
Assets/ConformalDecals/BoxSlider.cs
Normal file
36
Assets/ConformalDecals/BoxSlider.cs
Normal file
@ -0,0 +1,36 @@
|
||||
using System;
|
||||
using UnityEngine;
|
||||
using UnityEngine.Events;
|
||||
using UnityEngine.EventSystems;
|
||||
using UnityEngine.UI;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
[AddComponentMenu("UI/BoxSlider", 35)]
|
||||
[RequireComponent(typeof(RectTransform))]
|
||||
public class BoxSlider : Selectable, IDragHandler, IInitializePotentialDragHandler, ICanvasElement {
|
||||
[Serializable]
|
||||
public class BoxSliderEvent : UnityEvent<Vector2> { }
|
||||
|
||||
[SerializeField] private RectTransform _handleRect;
|
||||
[SerializeField] private Vector2 _value = Vector2.zero;
|
||||
|
||||
// Allow for delegate-based subscriptions for faster events than 'eventReceiver', and allowing for multiple receivers.
|
||||
[SerializeField] private BoxSliderEvent _onValueChanged = new BoxSliderEvent();
|
||||
|
||||
public BoxSliderEvent OnValueChanged {
|
||||
get => _onValueChanged;
|
||||
set => _onValueChanged = value;
|
||||
}
|
||||
|
||||
// Private fields
|
||||
public void OnDrag(PointerEventData eventData) { }
|
||||
|
||||
public void OnInitializePotentialDrag(PointerEventData eventData) { }
|
||||
|
||||
public void Rebuild(CanvasUpdate executing) { }
|
||||
|
||||
public void LayoutComplete() { }
|
||||
|
||||
public void GraphicUpdateComplete() { }
|
||||
}
|
||||
}
|
13
Assets/ConformalDecals/ColorBoxSlider.cs
Normal file
13
Assets/ConformalDecals/ColorBoxSlider.cs
Normal file
@ -0,0 +1,13 @@
|
||||
using UnityEngine;
|
||||
using UnityEngine.UI;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
public class ColorBoxSlider : MonoBehaviour{
|
||||
[SerializeField] private Vector2 _value;
|
||||
|
||||
[SerializeField] private BoxSlider _slider;
|
||||
[SerializeField] private Image _image;
|
||||
|
||||
public void OnSliderUpdate(Vector2 value) { }
|
||||
}
|
||||
}
|
18
Assets/ConformalDecals/ColorChannelSlider.cs
Normal file
18
Assets/ConformalDecals/ColorChannelSlider.cs
Normal file
@ -0,0 +1,18 @@
|
||||
using UnityEngine;
|
||||
using UnityEngine.UI;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
public class ColorChannelSlider : MonoBehaviour {
|
||||
[SerializeField] private float _value;
|
||||
[SerializeField] private int _channel;
|
||||
[SerializeField] private bool _hsv;
|
||||
|
||||
[SerializeField] private Selectable _textBox;
|
||||
[SerializeField] private Slider _slider;
|
||||
[SerializeField] private Image _image;
|
||||
|
||||
public void OnTextBoxUpdate(string text) { }
|
||||
|
||||
public void OnSliderUpdate(float value) { }
|
||||
}
|
||||
}
|
17
Assets/ConformalDecals/ColorPickerController.cs
Normal file
17
Assets/ConformalDecals/ColorPickerController.cs
Normal file
@ -0,0 +1,17 @@
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using UnityEngine;
|
||||
using UnityEngine.Events;
|
||||
using UnityEngine.UI;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
public class ColorPickerController : MonoBehaviour {
|
||||
[SerializeField] private Color _value;
|
||||
[SerializeField] private Image _previewImage;
|
||||
[SerializeField] private Selectable _hexTextBox;
|
||||
|
||||
public void Close() { }
|
||||
|
||||
public void OnHexColorUpdate(string text) { }
|
||||
}
|
||||
}
|
3
Assets/ConformalDecals/ConformalDecals.asmdef
Normal file
3
Assets/ConformalDecals/ConformalDecals.asmdef
Normal file
@ -0,0 +1,3 @@
|
||||
{
|
||||
"name": "ConformalDecals"
|
||||
}
|
10
Assets/ConformalDecals/FontMenuController.cs
Normal file
10
Assets/ConformalDecals/FontMenuController.cs
Normal file
@ -0,0 +1,10 @@
|
||||
using UnityEngine;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
public class FontMenuController : MonoBehaviour {
|
||||
[SerializeField] private GameObject _menuItem;
|
||||
[SerializeField] private GameObject _menuList;
|
||||
|
||||
public void Close() { }
|
||||
}
|
||||
}
|
37
Assets/ConformalDecals/TextEntryController.cs
Normal file
37
Assets/ConformalDecals/TextEntryController.cs
Normal file
@ -0,0 +1,37 @@
|
||||
using UnityEngine;
|
||||
using UnityEngine.UI;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
public class TextEntryController : MonoBehaviour {
|
||||
[SerializeField] private Selectable _textBox;
|
||||
[SerializeField] private Button _fontButton;
|
||||
|
||||
[SerializeField] private Slider _lineSpacingSlider;
|
||||
[SerializeField] private Selectable _lineSpacingTextBox;
|
||||
|
||||
[SerializeField] private Slider _charSpacingSlider;
|
||||
[SerializeField] private Selectable _charSpacingTextBox;
|
||||
|
||||
[SerializeField] private Toggle _boldButton;
|
||||
[SerializeField] private Toggle _italicButton;
|
||||
[SerializeField] private Toggle _underlineButton;
|
||||
[SerializeField] private Toggle _smallCapsButton;
|
||||
[SerializeField] private Toggle _verticalButton;
|
||||
|
||||
public void Close() { }
|
||||
|
||||
public void OnTextUpdate(string text) { }
|
||||
|
||||
public void OnFontMenu() { }
|
||||
|
||||
public void OnLineSpacingUpdate(float value) { }
|
||||
public void OnLineSpacingUpdate(string text) { }
|
||||
public void OnCharSpacingUpdate(float value) { }
|
||||
public void OnCharSpacingUpdate(string text) { }
|
||||
public void OnBoldUpdate(bool state) { }
|
||||
public void OnItalicUpdate(bool state) { }
|
||||
public void OnUnderlineUpdate(bool state) { }
|
||||
public void OnSmallCapsUpdate(bool state) { }
|
||||
public void OnVerticalUpdate(bool state) { }
|
||||
}
|
||||
}
|
1
Assets/ConformalDecals/UITag.cs
Symbolic link
1
Assets/ConformalDecals/UITag.cs
Symbolic link
@ -0,0 +1 @@
|
||||
/Users/drewcassidy/Projects/KSP-Conformal-Decals/Source/ConformalDecals/UI/UITag.cs
|
@ -1,4 +1,5 @@
|
||||
using UnityEngine;
|
||||
using System;
|
||||
using UnityEngine;
|
||||
|
||||
[ExecuteInEditMode]
|
||||
public class DecalProjectorTest : MonoBehaviour
|
||||
@ -23,9 +24,6 @@ public class DecalProjectorTest : MonoBehaviour
|
||||
void Awake()
|
||||
{
|
||||
_projectionMatrix = Matrix4x4.identity;
|
||||
_matrixID = Shader.PropertyToID("_ProjectionMatrix");
|
||||
_normalID = Shader.PropertyToID("_DecalNormal");
|
||||
_tangentID= Shader.PropertyToID("_DecalTangent");
|
||||
targetRenderer = target.GetComponent<MeshRenderer>();
|
||||
}
|
||||
|
||||
@ -40,8 +38,9 @@ public class DecalProjectorTest : MonoBehaviour
|
||||
var projectorToTarget = targetRenderer.worldToLocalMatrix * transform.localToWorldMatrix;
|
||||
_projectionMatrix = _OrthoMatrix * targetToProjector;
|
||||
|
||||
targetMaterial.SetMatrix(_matrixID, _projectionMatrix);
|
||||
targetMaterial.SetVector(_normalID, projectorToTarget.MultiplyVector(Vector3.back).normalized);
|
||||
targetMaterial.SetVector(_tangentID, projectorToTarget.MultiplyVector(Vector3.right).normalized);
|
||||
targetMaterial.SetMatrix("_ProjectionMatrix", _projectionMatrix);
|
||||
targetMaterial.SetVector("_DecalNormal", projectorToTarget.MultiplyVector(Vector3.back).normalized);
|
||||
targetMaterial.SetVector("_DecalTangent", projectorToTarget.MultiplyVector(Vector3.right).normalized);
|
||||
}
|
||||
|
||||
}
|
117
Assets/Scripts/TextRenderTest.cs
Normal file
117
Assets/Scripts/TextRenderTest.cs
Normal file
@ -0,0 +1,117 @@
|
||||
using System.Collections;
|
||||
using System.Collections.Generic;
|
||||
using TMPro;
|
||||
using UnityEngine;
|
||||
using UnityEngine.Rendering;
|
||||
|
||||
public class TextRenderTest : MonoBehaviour {
|
||||
//[InspectorButton("go")] public bool button;
|
||||
|
||||
public Camera _camera;
|
||||
|
||||
public GameObject _cameraObject;
|
||||
|
||||
public TextMeshPro _tmp;
|
||||
|
||||
public Material _blitMaterial;
|
||||
|
||||
public Material _targetMaterial;
|
||||
|
||||
public RenderTexture renderTex;
|
||||
private float pixelDensity = 8;
|
||||
private int MaxTextureSize = 4096;
|
||||
private static readonly int Decal = Shader.PropertyToID("_Decal");
|
||||
|
||||
public const TextureFormat TextTextureFormat = TextureFormat.RG16;
|
||||
public const RenderTextureFormat TextRenderTextureFormat = RenderTextureFormat.R8;
|
||||
|
||||
// Start is called before the first frame update
|
||||
void Start() {
|
||||
Debug.Log("starting...");
|
||||
|
||||
StartCoroutine(OnRender());
|
||||
}
|
||||
|
||||
// Update is called once per frame
|
||||
void Update() { }
|
||||
|
||||
private IEnumerator OnRender() {
|
||||
Debug.Log("starting...2");
|
||||
|
||||
// calculate camera and texture size
|
||||
_tmp.ForceMeshUpdate();
|
||||
var mesh = _tmp.mesh;
|
||||
mesh.RecalculateBounds();
|
||||
var bounds = mesh.bounds;
|
||||
Debug.Log(bounds.size);
|
||||
|
||||
var width = bounds.size.x * pixelDensity;
|
||||
var height = bounds.size.y * pixelDensity;
|
||||
|
||||
var widthPoT = Mathf.NextPowerOfTwo((int) width);
|
||||
var heightPoT = Mathf.NextPowerOfTwo((int) height);
|
||||
|
||||
if (widthPoT > MaxTextureSize) {
|
||||
widthPoT /= widthPoT / MaxTextureSize;
|
||||
heightPoT /= widthPoT / MaxTextureSize;
|
||||
}
|
||||
|
||||
if (heightPoT > MaxTextureSize) {
|
||||
widthPoT /= heightPoT / MaxTextureSize;
|
||||
heightPoT /= heightPoT / MaxTextureSize;
|
||||
}
|
||||
|
||||
widthPoT = Mathf.Min(widthPoT, MaxTextureSize);
|
||||
heightPoT = Mathf.Min(heightPoT, MaxTextureSize);
|
||||
|
||||
var widthRatio = widthPoT / width;
|
||||
var heightRatio = heightPoT / height;
|
||||
|
||||
var sizeRatio = Mathf.Min(widthRatio, heightRatio);
|
||||
|
||||
Debug.Log(sizeRatio);
|
||||
|
||||
int scaledHeight = (int) (sizeRatio * height);
|
||||
int scaledWidth = (int) (sizeRatio * width);
|
||||
|
||||
Debug.Log($"width = {scaledWidth}");
|
||||
Debug.Log($"height = {scaledHeight}");
|
||||
|
||||
_camera.orthographicSize = scaledHeight / pixelDensity / 2;
|
||||
_camera.aspect = (float) widthPoT / heightPoT;
|
||||
|
||||
_cameraObject.transform.localPosition = new Vector3(bounds.center.x, bounds.center.y, -1);
|
||||
|
||||
var halfHeight = heightPoT / pixelDensity / 2 / sizeRatio;
|
||||
var halfWidth = widthPoT / pixelDensity / 2 / sizeRatio;
|
||||
var matrix = Matrix4x4.Ortho(bounds.center.x - halfWidth, bounds.center.x + halfWidth,
|
||||
bounds.center.y - halfHeight, bounds.center.y + halfHeight, -1, 1);
|
||||
|
||||
// setup texture
|
||||
var texture = new Texture2D(widthPoT, heightPoT, TextTextureFormat, true);
|
||||
_targetMaterial.SetTexture(Decal, texture);
|
||||
|
||||
|
||||
// setup render texture
|
||||
renderTex = RenderTexture.GetTemporary(widthPoT, heightPoT, 0, TextRenderTextureFormat, RenderTextureReadWrite.Linear, 1);
|
||||
renderTex.autoGenerateMips = false;
|
||||
|
||||
RenderTexture.active = renderTex;
|
||||
GL.PushMatrix();
|
||||
GL.LoadProjectionMatrix(matrix);
|
||||
_blitMaterial.SetPass(0);
|
||||
Graphics.DrawMeshNow(mesh, Matrix4x4.identity);
|
||||
GL.PopMatrix();
|
||||
|
||||
// setup material
|
||||
_blitMaterial.mainTexture = _tmp.font.atlas;
|
||||
|
||||
yield return null;
|
||||
|
||||
RenderTexture.active = renderTex;
|
||||
texture.ReadPixels(new Rect(0, 0, widthPoT, heightPoT), 0, 0, true);
|
||||
texture.Apply(false, true);
|
||||
|
||||
RenderTexture.ReleaseTemporary(renderTex);
|
||||
}
|
||||
}
|
143
Assets/Shaders/ColorSlider.shader
Normal file
143
Assets/Shaders/ColorSlider.shader
Normal file
@ -0,0 +1,143 @@
|
||||
Shader "ConformalDecals/UI/Color Slider"
|
||||
{
|
||||
Properties
|
||||
{
|
||||
_Color("Color", Color) = (0,0,0,0)
|
||||
_Radius("Radius", Float) = 4
|
||||
|
||||
_OutlineGradient("Outline Gradient Step", Range (0, 1)) = 0.6
|
||||
_OutlineOpacity("Outline Opacity", Range (0, 0.5)) = 0.1
|
||||
_OutlineWidth("Outline Width", Float) = 3
|
||||
|
||||
_StencilComp ("Stencil Comparison", Float) = 8
|
||||
_Stencil ("Stencil ID", Float) = 0
|
||||
_StencilOp ("Stencil Operation", Float) = 0
|
||||
_StencilWriteMask ("Stencil Write Mask", Float) = 255
|
||||
_StencilReadMask ("Stencil Read Mask", Float) = 255
|
||||
|
||||
_ColorMask ("Color Mask", Float) = 15
|
||||
|
||||
[Toggle(UNITY_UI_ALPHACLIP)] _UseUIAlphaClip ("Use Alpha Clip", Float) = 0
|
||||
|
||||
[Toggle(HUE)] _Hue ("Hue", int) = 0
|
||||
[Toggle(RED)] _Red ("Red", int) = 0
|
||||
[Toggle(GREEN)] _Green ("Green", int) = 0
|
||||
[Toggle(BLUE)] _Blue ("Blue", int) = 0
|
||||
|
||||
|
||||
}
|
||||
SubShader
|
||||
{
|
||||
Tags
|
||||
{
|
||||
"Queue"="Transparent"
|
||||
"IgnoreProjector"="True"
|
||||
"RenderType"="Transparent"
|
||||
"PreviewType"="Plane"
|
||||
"CanUseSpriteAtlas"="True"
|
||||
}
|
||||
Stencil
|
||||
{
|
||||
Ref [_Stencil]
|
||||
Comp [_StencilComp]
|
||||
Pass [_StencilOp]
|
||||
ReadMask [_StencilReadMask]
|
||||
WriteMask [_StencilWriteMask]
|
||||
}
|
||||
|
||||
Cull Off
|
||||
Lighting Off
|
||||
ZWrite Off
|
||||
ZTest [unity_GUIZTestMode]
|
||||
Blend SrcAlpha OneMinusSrcAlpha
|
||||
ColorMask [_ColorMask]
|
||||
|
||||
Pass
|
||||
{
|
||||
CGPROGRAM
|
||||
#pragma vertex vert
|
||||
#pragma fragment frag
|
||||
|
||||
#pragma require integers
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "UnityUI.cginc"
|
||||
#include "HSL.cginc"
|
||||
#include "SDF.cginc"
|
||||
|
||||
#pragma multi_compile_local _ UNITY_UI_CLIP_RECT
|
||||
#pragma multi_compile_local _ UNITY_UI_ALPHACLIP
|
||||
#pragma multi_compile_local HUE RED GREEN BLUE
|
||||
|
||||
float4 _ClipRect;
|
||||
float _Radius;
|
||||
float4 _Color;
|
||||
|
||||
float _OutlineGradient;
|
||||
float _OutlineOpacity;
|
||||
float _OutlineWidth;
|
||||
|
||||
struct appdata
|
||||
{
|
||||
float4 vertex : POSITION;
|
||||
float2 uv : TEXCOORD0;
|
||||
};
|
||||
|
||||
struct v2f
|
||||
{
|
||||
float4 vertex : SV_POSITION;
|
||||
float2 uv : TEXCOORD0;
|
||||
float4 worldPosition : TEXCOORD1;
|
||||
};
|
||||
|
||||
v2f vert (appdata v)
|
||||
{
|
||||
v2f o;
|
||||
o.vertex = UnityObjectToClipPos(v.vertex);
|
||||
o.worldPosition = v.vertex;
|
||||
o.uv = v.uv;
|
||||
return o;
|
||||
}
|
||||
|
||||
fixed4 frag (v2f i) : SV_Target
|
||||
{
|
||||
float4 color = 1;
|
||||
|
||||
#ifdef HUE
|
||||
color.rgb = HSV2RGB(float3(i.uv.y, _Color.y, _Color.z));
|
||||
#endif //HUE
|
||||
|
||||
#ifdef RED
|
||||
color.rgb = float3(i.uv.x, _Color.g, _Color.b);
|
||||
#endif //RED
|
||||
|
||||
#ifdef GREEN
|
||||
color.rgb = float3(_Color.r, i.uv.x, _Color.b);
|
||||
#endif //GREEN
|
||||
|
||||
#ifdef BLUE
|
||||
color.rgb = float3(_Color.r, _Color.g, i.uv.x);
|
||||
#endif //BLUE
|
||||
|
||||
float rrect = sdRoundedUVBox(i.uv, _Radius);
|
||||
float gradient = smoothstep(_OutlineGradient, 1 - _OutlineGradient, i.uv.y);
|
||||
float outlineOpacity = _OutlineOpacity * smoothstep(-1*_OutlineWidth, 0, rrect);
|
||||
|
||||
color.rgb = lerp(color.rgb, gradient, outlineOpacity);
|
||||
|
||||
color.a = saturate(0.5 - rrect);
|
||||
|
||||
#ifdef UNITY_UI_CLIP_RECT
|
||||
color.a *= UnityGet2DClipping(i.worldPosition.xy, _ClipRect);
|
||||
#endif
|
||||
|
||||
#ifdef UNITY_UI_ALPHACLIP
|
||||
clip (color.a - 0.001);
|
||||
#endif
|
||||
|
||||
return color;
|
||||
}
|
||||
ENDCG
|
||||
}
|
||||
}
|
||||
}
|
122
Assets/Shaders/ColorSquare.shader
Normal file
122
Assets/Shaders/ColorSquare.shader
Normal file
@ -0,0 +1,122 @@
|
||||
Shader "ConformalDecals/UI/HSV Square"
|
||||
{
|
||||
Properties
|
||||
{
|
||||
_Color("Color", Color) = (0,0,0,0)
|
||||
_Radius("Radius", Float) = 4
|
||||
|
||||
_OutlineGradient("Outline Gradient Step", Range (0, 1)) = 0.6
|
||||
_OutlineOpacity("Outline Opacity", Range (0, 0.5)) = 0.1
|
||||
_OutlineWidth("Outline Width", Float) = 3
|
||||
|
||||
_StencilComp ("Stencil Comparison", Float) = 8
|
||||
_Stencil ("Stencil ID", Float) = 0
|
||||
_StencilOp ("Stencil Operation", Float) = 0
|
||||
_StencilWriteMask ("Stencil Write Mask", Float) = 255
|
||||
_StencilReadMask ("Stencil Read Mask", Float) = 255
|
||||
|
||||
_ColorMask ("Color Mask", Float) = 15
|
||||
|
||||
[Toggle(UNITY_UI_ALPHACLIP)] _UseUIAlphaClip ("Use Alpha Clip", Float) = 0
|
||||
}
|
||||
SubShader
|
||||
{
|
||||
Tags
|
||||
{
|
||||
"Queue"="Transparent"
|
||||
"IgnoreProjector"="True"
|
||||
"RenderType"="Transparent"
|
||||
"PreviewType"="Plane"
|
||||
"CanUseSpriteAtlas"="True"
|
||||
}
|
||||
Stencil
|
||||
{
|
||||
Ref [_Stencil]
|
||||
Comp [_StencilComp]
|
||||
Pass [_StencilOp]
|
||||
ReadMask [_StencilReadMask]
|
||||
WriteMask [_StencilWriteMask]
|
||||
}
|
||||
|
||||
Cull Off
|
||||
Lighting Off
|
||||
ZWrite Off
|
||||
ZTest [unity_GUIZTestMode]
|
||||
Blend SrcAlpha OneMinusSrcAlpha
|
||||
ColorMask [_ColorMask]
|
||||
|
||||
Pass
|
||||
{
|
||||
CGPROGRAM
|
||||
#pragma vertex vert
|
||||
#pragma fragment frag
|
||||
|
||||
#pragma require integers
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "UnityUI.cginc"
|
||||
#include "HSL.cginc"
|
||||
#include "SDF.cginc"
|
||||
|
||||
#pragma multi_compile_local _ UNITY_UI_CLIP_RECT
|
||||
#pragma multi_compile_local _ UNITY_UI_ALPHACLIP
|
||||
|
||||
float4 _ClipRect;
|
||||
float _Radius;
|
||||
float4 _Color;
|
||||
|
||||
float _OutlineGradient;
|
||||
float _OutlineOpacity;
|
||||
float _OutlineWidth;
|
||||
|
||||
struct appdata
|
||||
{
|
||||
float4 vertex : POSITION;
|
||||
float2 uv : TEXCOORD0;
|
||||
};
|
||||
|
||||
struct v2f
|
||||
{
|
||||
float4 vertex : SV_POSITION;
|
||||
float2 uv : TEXCOORD0;
|
||||
float4 worldPosition : TEXCOORD1;
|
||||
};
|
||||
|
||||
v2f vert (appdata v)
|
||||
{
|
||||
v2f o;
|
||||
o.vertex = UnityObjectToClipPos(v.vertex);
|
||||
o.worldPosition = v.vertex;
|
||||
o.uv = v.uv;
|
||||
return o;
|
||||
}
|
||||
|
||||
fixed4 frag (v2f i) : SV_Target
|
||||
{
|
||||
// sample the texture
|
||||
fixed4 color = 1;
|
||||
|
||||
color.a = saturate(0.5 - sdRoundedUVBox(i.uv, _Radius));
|
||||
|
||||
color.rgb = HSV2RGB(float3(_Color.x, i.uv.x, i.uv.y));
|
||||
|
||||
float rrect = sdRoundedUVBox(i.uv, _Radius);
|
||||
float gradient = smoothstep(_OutlineGradient, 1 - _OutlineGradient, i.uv.y);
|
||||
float outlineOpacity = _OutlineOpacity * smoothstep(-1*_OutlineWidth, 0, rrect);
|
||||
|
||||
color.rgb = lerp(color.rgb, gradient, outlineOpacity);
|
||||
|
||||
#ifdef UNITY_UI_CLIP_RECT
|
||||
color.a *= UnityGet2DClipping(i.worldPosition.xy, _ClipRect);
|
||||
#endif
|
||||
|
||||
#ifdef UNITY_UI_ALPHACLIP
|
||||
clip (color.a - 0.001);
|
||||
#endif
|
||||
|
||||
return color;
|
||||
}
|
||||
ENDCG
|
||||
}
|
||||
}
|
||||
}
|
120
Assets/Shaders/ColorSwatch.shader
Normal file
120
Assets/Shaders/ColorSwatch.shader
Normal file
@ -0,0 +1,120 @@
|
||||
Shader "ConformalDecals/UI/Color Swatch"
|
||||
{
|
||||
Properties
|
||||
{
|
||||
_Color("Color", Color) = (0,0,0,0)
|
||||
_Radius("Radius", Float) = 4
|
||||
|
||||
_OutlineGradient("Outline Gradient Step", Range (0, 1)) = 0.6
|
||||
_OutlineOpacity("Outline Opacity", Range (0, 0.5)) = 0.1
|
||||
_OutlineWidth("Outline Width", Float) = 3
|
||||
|
||||
_StencilComp ("Stencil Comparison", Float) = 8
|
||||
_Stencil ("Stencil ID", Float) = 0
|
||||
_StencilOp ("Stencil Operation", Float) = 0
|
||||
_StencilWriteMask ("Stencil Write Mask", Float) = 255
|
||||
_StencilReadMask ("Stencil Read Mask", Float) = 255
|
||||
|
||||
_ColorMask ("Color Mask", Float) = 15
|
||||
|
||||
[Toggle(UNITY_UI_ALPHACLIP)] _UseUIAlphaClip ("Use Alpha Clip", Float) = 0
|
||||
}
|
||||
SubShader
|
||||
{
|
||||
Tags
|
||||
{
|
||||
"Queue"="Transparent"
|
||||
"IgnoreProjector"="True"
|
||||
"RenderType"="Transparent"
|
||||
"PreviewType"="Plane"
|
||||
"CanUseSpriteAtlas"="True"
|
||||
}
|
||||
Stencil
|
||||
{
|
||||
Ref [_Stencil]
|
||||
Comp [_StencilComp]
|
||||
Pass [_StencilOp]
|
||||
ReadMask [_StencilReadMask]
|
||||
WriteMask [_StencilWriteMask]
|
||||
}
|
||||
|
||||
Cull Off
|
||||
Lighting Off
|
||||
ZWrite Off
|
||||
ZTest [unity_GUIZTestMode]
|
||||
Blend SrcAlpha OneMinusSrcAlpha
|
||||
ColorMask [_ColorMask]
|
||||
|
||||
Pass
|
||||
{
|
||||
CGPROGRAM
|
||||
#pragma vertex vert
|
||||
#pragma fragment frag
|
||||
|
||||
#pragma require integers
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "UnityUI.cginc"
|
||||
#include "HSL.cginc"
|
||||
#include "SDF.cginc"
|
||||
|
||||
#pragma multi_compile_local _ UNITY_UI_CLIP_RECT
|
||||
#pragma multi_compile_local _ UNITY_UI_ALPHACLIP
|
||||
#pragma multi_compile_local HUE RED GREEN BLUE
|
||||
|
||||
float4 _ClipRect;
|
||||
float _Radius;
|
||||
float4 _Color;
|
||||
|
||||
float _OutlineGradient;
|
||||
float _OutlineOpacity;
|
||||
float _OutlineWidth;
|
||||
|
||||
struct appdata
|
||||
{
|
||||
float4 vertex : POSITION;
|
||||
float2 uv : TEXCOORD0;
|
||||
};
|
||||
|
||||
struct v2f
|
||||
{
|
||||
float4 vertex : SV_POSITION;
|
||||
float2 uv : TEXCOORD0;
|
||||
float4 worldPosition : TEXCOORD1;
|
||||
};
|
||||
|
||||
v2f vert (appdata v)
|
||||
{
|
||||
v2f o;
|
||||
o.vertex = UnityObjectToClipPos(v.vertex);
|
||||
o.worldPosition = v.vertex;
|
||||
o.uv = v.uv;
|
||||
return o;
|
||||
}
|
||||
|
||||
fixed4 frag (v2f i) : SV_Target
|
||||
{
|
||||
fixed4 color = _Color;
|
||||
|
||||
color.a = saturate(0.5 - sdRoundedUVBox(i.uv, _Radius));
|
||||
|
||||
float rrect = sdRoundedUVBox(i.uv, _Radius);
|
||||
float gradient = smoothstep(_OutlineGradient, 1 - _OutlineGradient, i.uv.y);
|
||||
float outlineOpacity = _OutlineOpacity * smoothstep(-1*_OutlineWidth, 0, rrect);
|
||||
|
||||
color.rgb = lerp(color.rgb, gradient, outlineOpacity);
|
||||
|
||||
#ifdef UNITY_UI_CLIP_RECT
|
||||
color.a *= UnityGet2DClipping(i.worldPosition.xy, _ClipRect);
|
||||
#endif
|
||||
|
||||
#ifdef UNITY_UI_ALPHACLIP
|
||||
clip (color.a - 0.001);
|
||||
#endif
|
||||
|
||||
return color;
|
||||
}
|
||||
ENDCG
|
||||
}
|
||||
}
|
||||
}
|
@ -1,130 +0,0 @@
|
||||
Shader "ConformalDecals/Feature/Bumped"
|
||||
{
|
||||
Properties
|
||||
{
|
||||
[Header(Texture Maps)]
|
||||
_Decal("Decal Texture", 2D) = "gray" {}
|
||||
_DecalBumpMap("Decal Bump Map", 2D) = "bump" {}
|
||||
|
||||
_Cutoff ("Alpha cutoff", Range(0,1)) = 0.5
|
||||
_DecalOpacity("Opacity", Range(0,1) ) = 1
|
||||
_Background("Background Color", Color) = (0.9,0.9,0.9,0.7)
|
||||
|
||||
[Enum(UnityEngine.Rendering.CullMode)] _Cull ("Cull", int) = 2
|
||||
[Toggle(DECAL_PREVIEW)] _Preview ("Preview", int) = 0
|
||||
|
||||
[Header(Effects)]
|
||||
[PerRendererData]_Opacity("_Opacity", Range(0,1) ) = 1
|
||||
[PerRendererData]_Color("_Color", Color) = (1,1,1,1)
|
||||
[PerRendererData]_RimFalloff("_RimFalloff", Range(0.01,5) ) = 0.1
|
||||
[PerRendererData]_RimColor("_RimColor", Color) = (0,0,0,0)
|
||||
[PerRendererData]_UnderwaterFogFactor ("Underwater Fog Factor", Range(0,1)) = 0
|
||||
}
|
||||
SubShader
|
||||
{
|
||||
Tags { "Queue" = "Geometry+100" "IgnoreProjector" = "true"}
|
||||
Cull [_Cull]
|
||||
Ztest LEqual
|
||||
|
||||
Pass
|
||||
{
|
||||
Name "FORWARD"
|
||||
Tags { "LightMode" = "ForwardBase" }
|
||||
Blend SrcAlpha OneMinusSrcAlpha
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert_forward
|
||||
#pragma fragment frag_forward
|
||||
|
||||
#pragma multi_compile_fwdbase nolightmap nodirlightmap nodynlightmap
|
||||
#pragma multi_compile DECAL_PROJECT DECAL_PREVIEW
|
||||
|
||||
sampler2D _Decal;
|
||||
sampler2D _DecalBumpMap;
|
||||
|
||||
float4 _Decal_ST;
|
||||
float4 _DecalBumpMap_ST;
|
||||
|
||||
float _RimFalloff;
|
||||
float4 _RimColor;
|
||||
|
||||
#define DECAL_NORMAL
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "Lighting.cginc"
|
||||
#include "AutoLight.cginc"
|
||||
#include "LightingKSP.cginc"
|
||||
#include "DecalsCommon.cginc"
|
||||
|
||||
void surf (DecalSurfaceInput IN, inout SurfaceOutput o)
|
||||
{
|
||||
float4 color = tex2D(_Decal, IN.uv_decal);
|
||||
float3 normal = UnpackNormalDXT5nm(tex2D(_DecalBumpMap, IN.uv_bump));
|
||||
|
||||
decalClipAlpha(color.a - _Cutoff);
|
||||
|
||||
half rim = 1.0 - saturate(dot (normalize(IN.viewDir), normal));
|
||||
float3 emission = (_RimColor.rgb * pow(rim, _RimFalloff)) * _RimColor.a;
|
||||
|
||||
o.Albedo = UnderwaterFog(IN.worldPosition, color).rgb;
|
||||
o.Alpha = color.a * _DecalOpacity;
|
||||
o.Emission = emission;
|
||||
o.Normal = normal;
|
||||
}
|
||||
|
||||
ENDCG
|
||||
}
|
||||
|
||||
Pass
|
||||
{
|
||||
Name "FORWARD"
|
||||
Tags { "LightMode" = "ForwardAdd" }
|
||||
Blend One One
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert_forward
|
||||
#pragma fragment frag_forward
|
||||
|
||||
#pragma multi_compile_fwdadd nolightmap nodirlightmap nodynlightmap
|
||||
#pragma multi_compile DECAL_PROJECT DECAL_PREVIEW
|
||||
|
||||
sampler2D _Decal;
|
||||
sampler2D _DecalBumpMap;
|
||||
|
||||
float4 _Decal_ST;
|
||||
float4 _DecalBumpMap_ST;
|
||||
|
||||
float _RimFalloff;
|
||||
float4 _RimColor;
|
||||
|
||||
#define DECAL_NORMAL
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "Lighting.cginc"
|
||||
#include "AutoLight.cginc"
|
||||
#include "LightingKSP.cginc"
|
||||
#include "DecalsCommon.cginc"
|
||||
|
||||
void surf (DecalSurfaceInput IN, inout SurfaceOutput o)
|
||||
{
|
||||
float4 color = tex2D(_Decal, IN.uv_decal);
|
||||
float3 normal = UnpackNormal(tex2D(_DecalBumpMap, IN.uv_bump));
|
||||
|
||||
decalClipAlpha(color.a - _Cutoff);
|
||||
|
||||
half rim = 1.0 - saturate(dot (normalize(IN.viewDir), normal));
|
||||
float3 emission = (_RimColor.rgb * pow(rim, _RimFalloff)) * _RimColor.a;
|
||||
|
||||
o.Albedo = UnderwaterFog(IN.worldPosition, color).rgb;
|
||||
o.Alpha = color.a * _DecalOpacity;
|
||||
o.Emission = emission;
|
||||
o.Normal = normal;
|
||||
}
|
||||
|
||||
ENDCG
|
||||
}
|
||||
|
||||
// shadow casting support
|
||||
UsePass "Legacy Shaders/VertexLit/SHADOWCASTER"
|
||||
}
|
||||
}
|
@ -1,143 +0,0 @@
|
||||
Shader "ConformalDecals/Paint/Diffuse"
|
||||
{
|
||||
Properties
|
||||
{
|
||||
[Header(Texture Maps)]
|
||||
_Decal("Decal Texture", 2D) = "gray" {}
|
||||
_BumpMap("Bump Map", 2D) = "bump" {}
|
||||
|
||||
_EdgeWearStrength("Edge Wear Strength", Range(0,500)) = 100
|
||||
_EdgeWearOffset("Edge Wear Offset", Range(0,1)) = 0.1
|
||||
|
||||
_Cutoff ("Alpha cutoff", Range(0,1)) = 0.5
|
||||
_DecalOpacity("Opacity", Range(0,1) ) = 1
|
||||
_Background("Background Color", Color) = (0.9,0.9,0.9,0.7)
|
||||
|
||||
[Enum(UnityEngine.Rendering.CullMode)] _Cull ("Cull", int) = 2
|
||||
[Toggle(DECAL_PREVIEW)] _Preview ("Preview", int) = 0
|
||||
|
||||
[Header(Effects)]
|
||||
[PerRendererData]_Opacity("_Opacity", Range(0,1) ) = 1
|
||||
[PerRendererData]_Color("_Color", Color) = (1,1,1,1)
|
||||
[PerRendererData]_RimFalloff("_RimFalloff", Range(0.01,5) ) = 0.1
|
||||
[PerRendererData]_RimColor("_RimColor", Color) = (0,0,0,0)
|
||||
[PerRendererData]_UnderwaterFogFactor ("Underwater Fog Factor", Range(0,1)) = 0
|
||||
}
|
||||
SubShader
|
||||
{
|
||||
Tags { "Queue" = "Geometry+100" "IgnoreProjector" = "true"}
|
||||
Cull [_Cull]
|
||||
Ztest LEqual
|
||||
|
||||
Pass
|
||||
{
|
||||
Name "FORWARD"
|
||||
Tags { "LightMode" = "ForwardBase" }
|
||||
Blend SrcAlpha OneMinusSrcAlpha
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert_forward
|
||||
#pragma fragment frag_forward
|
||||
|
||||
#pragma multi_compile_fwdbase nolightmap nodirlightmap nodynlightmap
|
||||
#pragma multi_compile __ DECAL_PREVIEW
|
||||
|
||||
sampler2D _Decal;
|
||||
|
||||
float4 _Decal_ST;
|
||||
|
||||
float _EdgeWearStrength;
|
||||
float _EdgeWearOffset;
|
||||
|
||||
float _RimFalloff;
|
||||
float4 _RimColor;
|
||||
|
||||
#define DECAL_BASE_NORMAL
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "Lighting.cginc"
|
||||
#include "AutoLight.cginc"
|
||||
#include "LightingKSP.cginc"
|
||||
#include "DecalsCommon.cginc"
|
||||
|
||||
void surf (DecalSurfaceInput IN, inout SurfaceOutput o)
|
||||
{
|
||||
float4 color = tex2D(_Decal, IN.uv_decal);
|
||||
|
||||
decalClipAlpha(color.a - _Cutoff);
|
||||
|
||||
float3 normal = IN.normal;
|
||||
half rim = 1.0 - saturate(dot (normalize(IN.viewDir), normal));
|
||||
float3 emission = (_RimColor.rgb * pow(rim, _RimFalloff)) * _RimColor.a;
|
||||
|
||||
float wearFactor = 1 - normal.z;
|
||||
float wearFactorAlpha = saturate(_EdgeWearStrength * wearFactor);
|
||||
|
||||
color.a *= saturate(1 + _EdgeWearOffset - saturate(_EdgeWearStrength * wearFactor));
|
||||
|
||||
o.Albedo = UnderwaterFog(IN.worldPosition, color).rgb;
|
||||
o.Alpha = color.a * _DecalOpacity;
|
||||
o.Emission = emission;
|
||||
}
|
||||
|
||||
ENDCG
|
||||
}
|
||||
|
||||
Pass
|
||||
{
|
||||
Name "FORWARD"
|
||||
Tags { "LightMode" = "ForwardAdd" }
|
||||
Blend One One
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert_forward
|
||||
#pragma fragment frag_forward
|
||||
|
||||
#pragma multi_compile_fwdadd nolightmap nodirlightmap nodynlightmap
|
||||
#pragma multi_compile __ DECAL_PREVIEW
|
||||
|
||||
sampler2D _Decal;
|
||||
|
||||
float4 _Decal_ST;
|
||||
|
||||
float _EdgeWearStrength;
|
||||
float _EdgeWearOffset;
|
||||
|
||||
float _RimFalloff;
|
||||
float4 _RimColor;
|
||||
|
||||
#define DECAL_BASE_NORMAL
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "Lighting.cginc"
|
||||
#include "AutoLight.cginc"
|
||||
#include "LightingKSP.cginc"
|
||||
#include "DecalsCommon.cginc"
|
||||
|
||||
void surf (DecalSurfaceInput IN, inout SurfaceOutput o)
|
||||
{
|
||||
float4 color = tex2D(_Decal, IN.uv_decal);
|
||||
|
||||
decalClipAlpha(color.a - _Cutoff);
|
||||
|
||||
float3 normal = IN.normal;
|
||||
half rim = 1.0 - saturate(dot (normalize(IN.viewDir), normal));
|
||||
float3 emission = (_RimColor.rgb * pow(rim, _RimFalloff)) * _RimColor.a;
|
||||
|
||||
float wearFactor = 1 - normal.z;
|
||||
float wearFactorAlpha = saturate(_EdgeWearStrength * wearFactor);
|
||||
|
||||
color.a *= saturate(1 + _EdgeWearOffset - saturate(_EdgeWearStrength * wearFactor));
|
||||
|
||||
o.Albedo = UnderwaterFog(IN.worldPosition, color).rgb;
|
||||
o.Alpha = color.a * _DecalOpacity;
|
||||
o.Emission = emission;
|
||||
}
|
||||
|
||||
ENDCG
|
||||
}
|
||||
|
||||
// shadow casting support
|
||||
UsePass "Legacy Shaders/VertexLit/SHADOWCASTER"
|
||||
}
|
||||
}
|
@ -1,159 +0,0 @@
|
||||
Shader "ConformalDecals/Paint/DiffuseSDF"
|
||||
{
|
||||
Properties
|
||||
{
|
||||
[Header(Texture Maps)]
|
||||
_Decal("Decal Texture", 2D) = "gray" {}
|
||||
_BumpMap("Bump Map", 2D) = "bump" {}
|
||||
|
||||
_EdgeWearStrength("Edge Wear Strength", Range(0,500)) = 100
|
||||
_EdgeWearOffset("Edge Wear Offset", Range(0,1)) = 0.1
|
||||
|
||||
_Cutoff ("Alpha cutoff", Range(0,1)) = 0.5
|
||||
_Smoothness ("SDF smoothness", Range(0,1)) = 0.15
|
||||
_SmoothnessMipScale ("Smoothness fadeout", Range(0,1)) = 0.1
|
||||
_DecalOpacity("Opacity", Range(0,1) ) = 1
|
||||
_Background("Background Color", Color) = (0.9,0.9,0.9,0.7)
|
||||
|
||||
[Enum(UnityEngine.Rendering.CullMode)] _Cull ("Cull", int) = 2
|
||||
[Toggle(DECAL_PREVIEW)] _Preview ("Preview", int) = 0
|
||||
|
||||
[Header(Effects)]
|
||||
[PerRendererData]_Opacity("_Opacity", Range(0,1) ) = 1
|
||||
[PerRendererData]_Color("_Color", Color) = (1,1,1,1)
|
||||
[PerRendererData]_RimFalloff("_RimFalloff", Range(0.01,5) ) = 0.1
|
||||
[PerRendererData]_RimColor("_RimColor", Color) = (0,0,0,0)
|
||||
[PerRendererData]_UnderwaterFogFactor ("Underwater Fog Factor", Range(0,1)) = 0
|
||||
}
|
||||
SubShader
|
||||
{
|
||||
Tags { "Queue" = "Geometry+100" "IgnoreProjector" = "true"}
|
||||
Cull [_Cull]
|
||||
Ztest LEqual
|
||||
|
||||
Pass
|
||||
{
|
||||
Name "FORWARD"
|
||||
Tags { "LightMode" = "ForwardBase" }
|
||||
Blend SrcAlpha OneMinusSrcAlpha
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert_forward
|
||||
#pragma fragment frag_forward
|
||||
|
||||
#pragma multi_compile_fwdbase nolightmap nodirlightmap nodynlightmap
|
||||
#pragma multi_compile __ DECAL_PREVIEW
|
||||
|
||||
sampler2D _Decal;
|
||||
|
||||
float4 _Decal_ST;
|
||||
float4 _Decal_TexelSize;
|
||||
|
||||
float _Smoothness;
|
||||
float _SmoothnessMipScale;
|
||||
|
||||
float _EdgeWearStrength;
|
||||
float _EdgeWearOffset;
|
||||
|
||||
float _RimFalloff;
|
||||
float4 _RimColor;
|
||||
|
||||
#define DECAL_BASE_NORMAL
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "Lighting.cginc"
|
||||
#include "AutoLight.cginc"
|
||||
#include "LightingKSP.cginc"
|
||||
#include "DecalsCommon.cginc"
|
||||
|
||||
void surf (DecalSurfaceInput IN, inout SurfaceOutput o)
|
||||
{
|
||||
float4 color = tex2D(_Decal, IN.uv_decal);
|
||||
float3 normal = IN.normal;
|
||||
|
||||
float smoothScale = (1 - saturate(1-(CalcMipLevel(IN.uv_decal * _Decal_TexelSize.zw) * _SmoothnessMipScale))) / 2;
|
||||
color.a = smoothstep(_Cutoff - smoothScale, saturate(_Smoothness + smoothScale + _Cutoff), color.a);
|
||||
|
||||
decalClipAlpha(color.a);
|
||||
|
||||
half rim = 1.0 - saturate(dot (normalize(IN.viewDir), normal));
|
||||
float3 emission = (_RimColor.rgb * pow(rim, _RimFalloff)) * _RimColor.a;
|
||||
|
||||
float wearFactor = 1 - normal.z;
|
||||
float wearFactorAlpha = saturate(_EdgeWearStrength * wearFactor);
|
||||
|
||||
color.a *= saturate(1 + _EdgeWearOffset - saturate(_EdgeWearStrength * wearFactor));
|
||||
|
||||
o.Albedo = UnderwaterFog(IN.worldPosition, color).rgb;
|
||||
o.Alpha = color.a * _DecalOpacity;
|
||||
o.Emission = emission;
|
||||
}
|
||||
|
||||
ENDCG
|
||||
}
|
||||
|
||||
Pass
|
||||
{
|
||||
Name "FORWARD"
|
||||
Tags { "LightMode" = "ForwardAdd" }
|
||||
Blend One One
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert_forward
|
||||
#pragma fragment frag_forward
|
||||
|
||||
#pragma multi_compile_fwdadd nolightmap nodirlightmap nodynlightmap
|
||||
#pragma multi_compile __ DECAL_PREVIEW
|
||||
|
||||
sampler2D _Decal;
|
||||
|
||||
float4 _Decal_ST;
|
||||
float4 _Decal_TexelSize;
|
||||
|
||||
float _Smoothness;
|
||||
float _SmoothnessMipScale;
|
||||
|
||||
float _EdgeWearStrength;
|
||||
float _EdgeWearOffset;
|
||||
|
||||
float _RimFalloff;
|
||||
float4 _RimColor;
|
||||
|
||||
#define DECAL_BASE_NORMAL
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "Lighting.cginc"
|
||||
#include "AutoLight.cginc"
|
||||
#include "LightingKSP.cginc"
|
||||
#include "DecalsCommon.cginc"
|
||||
|
||||
void surf (DecalSurfaceInput IN, inout SurfaceOutput o)
|
||||
{
|
||||
float4 color = tex2D(_Decal, IN.uv_decal);
|
||||
float3 normal = IN.normal;
|
||||
|
||||
float smoothScale = (1 - saturate(1-(CalcMipLevel(IN.uv_decal * _Decal_TexelSize.zw) * _SmoothnessMipScale))) / 2;
|
||||
color.a = smoothstep(_Cutoff - smoothScale, saturate(_Smoothness + smoothScale + _Cutoff), color.a);
|
||||
|
||||
decalClipAlpha(color.a);
|
||||
|
||||
half rim = 1.0 - saturate(dot (normalize(IN.viewDir), normal));
|
||||
float3 emission = (_RimColor.rgb * pow(rim, _RimFalloff)) * _RimColor.a;
|
||||
|
||||
float wearFactor = 1 - normal.z;
|
||||
float wearFactorAlpha = saturate(_EdgeWearStrength * wearFactor);
|
||||
|
||||
color.a *= saturate(1 + _EdgeWearOffset - saturate(_EdgeWearStrength * wearFactor));
|
||||
|
||||
o.Albedo = UnderwaterFog(IN.worldPosition, color).rgb;
|
||||
o.Alpha = color.a * _DecalOpacity;
|
||||
o.Emission = emission;
|
||||
}
|
||||
|
||||
ENDCG
|
||||
}
|
||||
|
||||
// shadow casting support
|
||||
UsePass "Legacy Shaders/VertexLit/SHADOWCASTER"
|
||||
}
|
||||
}
|
@ -1,165 +0,0 @@
|
||||
Shader "ConformalDecals/Paint/Specular"
|
||||
{
|
||||
Properties
|
||||
{
|
||||
[Header(Texture Maps)]
|
||||
_Decal("Decal Texture", 2D) = "gray" {}
|
||||
_BumpMap("Bump Map", 2D) = "bump" {}
|
||||
_SpecMap("Specular Map", 2D) = "black" {}
|
||||
|
||||
_EdgeWearStrength("Edge Wear Strength", Range(0,500)) = 100
|
||||
_EdgeWearOffset("Edge Wear Offset", Range(0,1)) = 0.1
|
||||
|
||||
_Cutoff ("Alpha cutoff", Range(0,1)) = 0.5
|
||||
_DecalOpacity("Opacity", Range(0,1) ) = 1
|
||||
_Background("Background Color", Color) = (0.9,0.9,0.9,0.7)
|
||||
|
||||
[Header(Specularity)]
|
||||
_SpecColor ("_SpecColor", Color) = (0.25, 0.25, 0.25, 1)
|
||||
_Shininess ("Shininess", Range (0.03, 10)) = 0.3
|
||||
|
||||
[Enum(UnityEngine.Rendering.CullMode)] _Cull ("Cull", int) = 2
|
||||
[Toggle(DECAL_PREVIEW)] _Preview ("Preview", int) = 0
|
||||
|
||||
[Header(Effects)]
|
||||
[PerRendererData]_Opacity("_Opacity", Range(0,1) ) = 1
|
||||
[PerRendererData]_Color("_Color", Color) = (1,1,1,1)
|
||||
[PerRendererData]_RimFalloff("_RimFalloff", Range(0.01,5) ) = 0.1
|
||||
[PerRendererData]_RimColor("_RimColor", Color) = (0,0,0,0)
|
||||
[PerRendererData]_UnderwaterFogFactor ("Underwater Fog Factor", Range(0,1)) = 0
|
||||
}
|
||||
SubShader
|
||||
{
|
||||
Tags { "Queue" = "Geometry+100" "IgnoreProjector" = "true"}
|
||||
Cull [_Cull]
|
||||
Ztest LEqual
|
||||
|
||||
Pass
|
||||
{
|
||||
Name "FORWARD"
|
||||
Tags { "LightMode" = "ForwardBase" }
|
||||
Blend SrcAlpha OneMinusSrcAlpha
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert_forward
|
||||
#pragma fragment frag_forward
|
||||
|
||||
#pragma multi_compile_fwdbase nolightmap nodirlightmap nodynlightmap
|
||||
#pragma multi_compile __ DECAL_PREVIEW
|
||||
|
||||
sampler2D _Decal;
|
||||
sampler2D _SpecMap;
|
||||
|
||||
float4 _Decal_ST;
|
||||
float4 _SpecMap_ST;
|
||||
|
||||
float _EdgeWearStrength;
|
||||
float _EdgeWearOffset;
|
||||
|
||||
half _Shininess;
|
||||
|
||||
float _RimFalloff;
|
||||
float4 _RimColor;
|
||||
|
||||
#define DECAL_BASE_NORMAL
|
||||
#define DECAL_SPECULAR
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "Lighting.cginc"
|
||||
#include "AutoLight.cginc"
|
||||
#include "LightingKSP.cginc"
|
||||
#include "DecalsCommon.cginc"
|
||||
|
||||
void surf (DecalSurfaceInput IN, inout SurfaceOutput o)
|
||||
{
|
||||
float4 color = tex2D(_Decal, IN.uv_decal);
|
||||
float3 specular = tex2D(_SpecMap, IN.uv_spec);
|
||||
|
||||
decalClipAlpha(color.a - _Cutoff);
|
||||
|
||||
float3 normal = IN.normal;
|
||||
half rim = 1.0 - saturate(dot (normalize(IN.viewDir), normal));
|
||||
float3 emission = (_RimColor.rgb * pow(rim, _RimFalloff)) * _RimColor.a;
|
||||
|
||||
float wearFactor = 1 - normal.z;
|
||||
float wearFactorAlpha = saturate(_EdgeWearStrength * wearFactor);
|
||||
|
||||
color.a *= saturate(1 + _EdgeWearOffset - saturate(_EdgeWearStrength * wearFactor));
|
||||
color.a *= _DecalOpacity;
|
||||
|
||||
o.Albedo = UnderwaterFog(IN.worldPosition, color).rgb;
|
||||
o.Alpha = color.a;
|
||||
o.Emission = emission;
|
||||
o.Specular = _Shininess;
|
||||
o.Gloss = specular.r * color.a;
|
||||
}
|
||||
|
||||
ENDCG
|
||||
}
|
||||
|
||||
Pass
|
||||
{
|
||||
Name "FORWARD"
|
||||
Tags { "LightMode" = "ForwardAdd" }
|
||||
Blend One One
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert_forward
|
||||
#pragma fragment frag_forward
|
||||
|
||||
#pragma multi_compile_fwdadd nolightmap nodirlightmap nodynlightmap
|
||||
#pragma multi_compile __ DECAL_PREVIEW
|
||||
|
||||
sampler2D _Decal;
|
||||
sampler2D _SpecMap;
|
||||
|
||||
float4 _Decal_ST;
|
||||
float4 _SpecMap_ST;
|
||||
|
||||
float _EdgeWearStrength;
|
||||
float _EdgeWearOffset;
|
||||
|
||||
half _Shininess;
|
||||
|
||||
float _RimFalloff;
|
||||
float4 _RimColor;
|
||||
|
||||
#define DECAL_BASE_NORMAL
|
||||
#define DECAL_SPECULAR
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "Lighting.cginc"
|
||||
#include "AutoLight.cginc"
|
||||
#include "LightingKSP.cginc"
|
||||
#include "DecalsCommon.cginc"
|
||||
|
||||
void surf (DecalSurfaceInput IN, inout SurfaceOutput o)
|
||||
{
|
||||
float4 color = tex2D(_Decal, IN.uv_decal);
|
||||
float3 specular = tex2D(_SpecMap, IN.uv_spec);
|
||||
float3 normal = IN.normal;
|
||||
|
||||
decalClipAlpha(color.a - _Cutoff);
|
||||
|
||||
half rim = 1.0 - saturate(dot (normalize(IN.viewDir), normal));
|
||||
float3 emission = (_RimColor.rgb * pow(rim, _RimFalloff)) * _RimColor.a;
|
||||
|
||||
float wearFactor = 1 - normal.z;
|
||||
float wearFactorAlpha = saturate(_EdgeWearStrength * wearFactor);
|
||||
|
||||
color.a *= saturate(1 + _EdgeWearOffset - saturate(_EdgeWearStrength * wearFactor));
|
||||
|
||||
o.Albedo = UnderwaterFog(IN.worldPosition, color).rgb;
|
||||
o.Alpha = color.a * _DecalOpacity;
|
||||
o.Emission = emission;
|
||||
o.Specular = _Shininess;
|
||||
o.Gloss = specular.r;
|
||||
}
|
||||
|
||||
ENDCG
|
||||
}
|
||||
|
||||
// shadow casting support
|
||||
UsePass "Legacy Shaders/VertexLit/SHADOWCASTER"
|
||||
}
|
||||
}
|
@ -1,180 +0,0 @@
|
||||
Shader "ConformalDecals/Paint/SpecularSDF"
|
||||
{
|
||||
Properties
|
||||
{
|
||||
[Header(Texture Maps)]
|
||||
_Decal("Decal Texture", 2D) = "gray" {}
|
||||
_BumpMap("Bump Map", 2D) = "bump" {}
|
||||
_SpecMap("Specular Map", 2D) = "black" {}
|
||||
|
||||
_EdgeWearStrength("Edge Wear Strength", Range(0,500)) = 100
|
||||
_EdgeWearOffset("Edge Wear Offset", Range(0,1)) = 0.1
|
||||
|
||||
_Cutoff ("Alpha cutoff", Range(0,1)) = 0.5
|
||||
_Smoothness ("SDF smoothness", Range(0,1)) = 0.15
|
||||
_SmoothnessMipScale ("Smoothness fadeout", Range(0,1)) = 0.1
|
||||
_DecalOpacity("Opacity", Range(0,1) ) = 1
|
||||
_Background("Background Color", Color) = (0.9,0.9,0.9,0.7)
|
||||
|
||||
[Header(Specularity)]
|
||||
_SpecColor ("_SpecColor", Color) = (0.25, 0.25, 0.25, 1)
|
||||
_Shininess ("Shininess", Range (0.03, 10)) = 0.3
|
||||
|
||||
[Enum(UnityEngine.Rendering.CullMode)] _Cull ("Cull", int) = 2
|
||||
[Toggle(DECAL_PREVIEW)] _Preview ("Preview", int) = 0
|
||||
|
||||
[Header(Effects)]
|
||||
[PerRendererData]_Opacity("_Opacity", Range(0,1) ) = 1
|
||||
[PerRendererData]_Color("_Color", Color) = (1,1,1,1)
|
||||
[PerRendererData]_RimFalloff("_RimFalloff", Range(0.01,5) ) = 0.1
|
||||
[PerRendererData]_RimColor("_RimColor", Color) = (0,0,0,0)
|
||||
[PerRendererData]_UnderwaterFogFactor ("Underwater Fog Factor", Range(0,1)) = 0
|
||||
}
|
||||
SubShader
|
||||
{
|
||||
Tags { "Queue" = "Geometry+100" "IgnoreProjector" = "true"}
|
||||
Cull [_Cull]
|
||||
Ztest LEqual
|
||||
|
||||
Pass
|
||||
{
|
||||
Name "FORWARD"
|
||||
Tags { "LightMode" = "ForwardBase" }
|
||||
Blend SrcAlpha OneMinusSrcAlpha
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert_forward
|
||||
#pragma fragment frag_forward
|
||||
|
||||
#pragma multi_compile_fwdbase nolightmap nodirlightmap nodynlightmap
|
||||
#pragma multi_compile __ DECAL_PREVIEW
|
||||
|
||||
sampler2D _Decal;
|
||||
sampler2D _SpecMap;
|
||||
|
||||
float4 _Decal_ST;
|
||||
float4 _Decal_TexelSize;
|
||||
float4 _SpecMap_ST;
|
||||
|
||||
float _Smoothness;
|
||||
float _SmoothnessMipScale;
|
||||
|
||||
float _EdgeWearStrength;
|
||||
float _EdgeWearOffset;
|
||||
|
||||
half _Shininess;
|
||||
|
||||
float _RimFalloff;
|
||||
float4 _RimColor;
|
||||
|
||||
#define DECAL_BASE_NORMAL
|
||||
#define DECAL_SPECULAR
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "Lighting.cginc"
|
||||
#include "AutoLight.cginc"
|
||||
#include "LightingKSP.cginc"
|
||||
#include "DecalsCommon.cginc"
|
||||
|
||||
void surf (DecalSurfaceInput IN, inout SurfaceOutput o)
|
||||
{
|
||||
float4 color = tex2D(_Decal, IN.uv_decal);
|
||||
float3 specular = tex2D(_SpecMap, IN.uv_spec);
|
||||
float3 normal = IN.normal;
|
||||
|
||||
float smoothScale = (1 - saturate(1-(CalcMipLevel(IN.uv_decal * _Decal_TexelSize.zw) * _SmoothnessMipScale))) / 2;
|
||||
color.a = smoothstep(_Cutoff - smoothScale, saturate(_Smoothness + smoothScale + _Cutoff), color.a);
|
||||
|
||||
decalClipAlpha(color.a);
|
||||
|
||||
half rim = 1.0 - saturate(dot (normalize(IN.viewDir), normal));
|
||||
float3 emission = (_RimColor.rgb * pow(rim, _RimFalloff)) * _RimColor.a;
|
||||
|
||||
float wearFactor = 1 - normal.z;
|
||||
float wearFactorAlpha = saturate(_EdgeWearStrength * wearFactor);
|
||||
|
||||
color.a *= saturate(1 + _EdgeWearOffset - saturate(_EdgeWearStrength * wearFactor));
|
||||
|
||||
o.Albedo = UnderwaterFog(IN.worldPosition, color).rgb;
|
||||
o.Alpha = color.a * _DecalOpacity;
|
||||
o.Emission = emission;
|
||||
o.Specular = _Shininess;
|
||||
o.Gloss = specular.r;
|
||||
}
|
||||
|
||||
ENDCG
|
||||
}
|
||||
|
||||
Pass
|
||||
{
|
||||
Name "FORWARD"
|
||||
Tags { "LightMode" = "ForwardAdd" }
|
||||
Blend One One
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert_forward
|
||||
#pragma fragment frag_forward
|
||||
|
||||
#pragma multi_compile_fwdadd nolightmap nodirlightmap nodynlightmap
|
||||
#pragma multi_compile __ DECAL_PREVIEW
|
||||
|
||||
sampler2D _Decal;
|
||||
sampler2D _SpecMap;
|
||||
|
||||
float4 _Decal_ST;
|
||||
float4 _Decal_TexelSize;
|
||||
float4 _SpecMap_ST;
|
||||
|
||||
float _Smoothness;
|
||||
float _SmoothnessMipScale;
|
||||
|
||||
float _EdgeWearStrength;
|
||||
float _EdgeWearOffset;
|
||||
|
||||
half _Shininess;
|
||||
|
||||
float _RimFalloff;
|
||||
float4 _RimColor;
|
||||
|
||||
#define DECAL_BASE_NORMAL
|
||||
#define DECAL_SPECULAR
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "Lighting.cginc"
|
||||
#include "AutoLight.cginc"
|
||||
#include "LightingKSP.cginc"
|
||||
#include "DecalsCommon.cginc"
|
||||
|
||||
void surf (DecalSurfaceInput IN, inout SurfaceOutput o)
|
||||
{
|
||||
float4 color = tex2D(_Decal, IN.uv_decal);
|
||||
float3 specular = tex2D(_SpecMap, IN.uv_spec);
|
||||
float3 normal = IN.normal;
|
||||
|
||||
float smoothScale = (1 - saturate(1-(CalcMipLevel(IN.uv_decal * _Decal_TexelSize.zw) * _SmoothnessMipScale))) / 2;
|
||||
color.a = smoothstep(_Cutoff - smoothScale, saturate(_Smoothness + smoothScale + _Cutoff), color.a);
|
||||
|
||||
decalClipAlpha(color.a);
|
||||
|
||||
half rim = 1.0 - saturate(dot (normalize(IN.viewDir), normal));
|
||||
float3 emission = (_RimColor.rgb * pow(rim, _RimFalloff)) * _RimColor.a;
|
||||
|
||||
float wearFactor = 1 - normal.z;
|
||||
float wearFactorAlpha = saturate(_EdgeWearStrength * wearFactor);
|
||||
|
||||
color.a *= saturate(1 + _EdgeWearOffset - saturate(_EdgeWearStrength * wearFactor));
|
||||
|
||||
o.Albedo = UnderwaterFog(IN.worldPosition, color).rgb;
|
||||
o.Alpha = color.a * _DecalOpacity;
|
||||
o.Emission = emission;
|
||||
o.Specular = _Shininess;
|
||||
o.Gloss = specular.r;
|
||||
}
|
||||
|
||||
ENDCG
|
||||
}
|
||||
|
||||
// shadow casting support
|
||||
UsePass "Legacy Shaders/VertexLit/SHADOWCASTER"
|
||||
}
|
||||
}
|
@ -1,26 +1,93 @@
|
||||
#ifndef DECALS_COMMON_INCLUDED
|
||||
#define DECALS_COMMON_INCLUDED
|
||||
|
||||
#include "AutoLight.cginc"
|
||||
#include "Lighting.cginc"
|
||||
|
||||
#define CLIP_MARGIN 0.05
|
||||
#define EDGE_MARGIN 0.01
|
||||
|
||||
// UNIFORM VARIABLES
|
||||
// Projection matrix, normal, and tangent vectors
|
||||
float4x4 _ProjectionMatrix;
|
||||
float3 _DecalNormal;
|
||||
float3 _DecalTangent;
|
||||
|
||||
// Common Shading Paramaters
|
||||
float _Cutoff;
|
||||
float _DecalOpacity;
|
||||
float4 _Background;
|
||||
|
||||
sampler2D _Decal;
|
||||
float4 _Decal_ST;
|
||||
|
||||
// Variant Shading Parameters
|
||||
#ifdef DECAL_BASE_NORMAL
|
||||
sampler2D _BumpMap;
|
||||
float4 _BumpMap_ST;
|
||||
float _EdgeWearStrength;
|
||||
float _EdgeWearOffset;
|
||||
#endif //DECAL_BASE_NORMAL
|
||||
|
||||
#ifdef DECAL_BUMPMAP
|
||||
sampler2D _BumpMap;
|
||||
float4 _BumpMap_ST;
|
||||
#endif //DECAL_BUMPMAP
|
||||
|
||||
#ifdef DECAL_SPECMAP
|
||||
sampler2D _SpecMap;
|
||||
float4 _SpecMap_ST;
|
||||
// specular color is declared in a unity CGINC for some reason??
|
||||
fixed _Shininess;
|
||||
#endif //DECAL_SPECMAP
|
||||
|
||||
#ifdef DECAL_EMISSIVE
|
||||
sampler2D _Emissive;
|
||||
float4 _Emissive_ST;
|
||||
fixed4 _Emissive_Color;
|
||||
#endif //DECAL_EMISSIVE
|
||||
|
||||
// KSP EFFECTS
|
||||
// opacity and color
|
||||
float _Opacity;
|
||||
float4 _Color;
|
||||
float _RimFalloff;
|
||||
float4 _RimColor;
|
||||
|
||||
// fog
|
||||
float4 _LocalCameraPos;
|
||||
float4 _LocalCameraDir;
|
||||
float4 _UnderwaterFogColor;
|
||||
float _UnderwaterMinAlphaFogDistance;
|
||||
float _UnderwaterMaxAlbedoFog;
|
||||
float _UnderwaterMaxAlphaFog;
|
||||
float _UnderwaterAlbedoDistanceScalar;
|
||||
float _UnderwaterAlphaDistanceScalar;
|
||||
float _UnderwaterFogFactor;
|
||||
|
||||
// SURFACE INPUT STRUCT
|
||||
struct DecalSurfaceInput
|
||||
{
|
||||
float3 uv;
|
||||
float2 uv_decal;
|
||||
|
||||
#ifdef DECAL_NORMAL
|
||||
float2 uv_bump;
|
||||
#endif //DECAL_NORMAL
|
||||
#ifdef DECAL_BUMPMAP
|
||||
float2 uv_bumpmap;
|
||||
#endif //DECAL_BUMPMAP
|
||||
|
||||
#ifdef DECAL_SPECULAR
|
||||
float2 uv_spec;
|
||||
#endif //DECAL_SPECULAR
|
||||
#ifdef DECAL_SPECMAP
|
||||
float2 uv_specmap;
|
||||
#endif //DECAL_SPECMAP
|
||||
|
||||
#ifdef DECAL_EMISSIVE
|
||||
float2 uv_glow;
|
||||
float2 uv_emissive;
|
||||
#endif //DECAL_EMISSIVE
|
||||
|
||||
#ifdef DECAL_BASE_NORMAL
|
||||
float3 normal;
|
||||
#endif
|
||||
|
||||
#endif //DECAL_BASE_NORMAL
|
||||
|
||||
float3 vertex_normal;
|
||||
float3 viewDir;
|
||||
float3 worldPosition;
|
||||
};
|
||||
@ -59,20 +126,6 @@ struct v2f
|
||||
#endif //UNITY_PASS_FORWARDADD
|
||||
};
|
||||
|
||||
// Projection matrix, normal, and tangent vectors
|
||||
float4x4 _ProjectionMatrix;
|
||||
float3 _DecalNormal;
|
||||
float3 _DecalTangent;
|
||||
|
||||
#ifdef DECAL_BASE_NORMAL
|
||||
sampler2D _BumpMap;
|
||||
float4 _BumpMap_ST;
|
||||
#endif //DECAL_BASE_NORMAL
|
||||
|
||||
float _Cutoff;
|
||||
float _DecalOpacity;
|
||||
float _Opacity;
|
||||
float4 _Background;
|
||||
|
||||
inline void decalClipAlpha(float alpha) {
|
||||
#ifndef DECAL_PREVIEW
|
||||
@ -88,200 +141,16 @@ inline float CalcMipLevel(float2 texture_coord) {
|
||||
return 0.5 * log2(delta_max_sqr);
|
||||
}
|
||||
|
||||
// modifed version of the KSP BlinnPhong because it does some weird things
|
||||
inline fixed4 LightingBlinnPhongDecal(SurfaceOutput s, fixed3 lightDir, half3 viewDir, fixed atten)
|
||||
{
|
||||
s.Normal = normalize(s.Normal);
|
||||
half3 h = normalize(lightDir + viewDir);
|
||||
|
||||
fixed diff = max(0, dot(s.Normal, lightDir));
|
||||
|
||||
float nh = max(0, dot(s.Normal, h));
|
||||
float spec = pow(nh, s.Specular*128.0) * s.Gloss;
|
||||
|
||||
fixed4 c = 0;
|
||||
c.rgb = (s.Albedo * _LightColor0.rgb * diff + _LightColor0.rgb * _SpecColor.rgb * spec) * (atten);
|
||||
return c;
|
||||
}
|
||||
|
||||
// declare surf function,
|
||||
// this must be defined in any shader using this cginc
|
||||
void surf (DecalSurfaceInput IN, inout SurfaceOutput o);
|
||||
|
||||
v2f vert_forward(appdata_decal v)
|
||||
{
|
||||
v2f o;
|
||||
UNITY_INITIALIZE_OUTPUT(v2f,o);
|
||||
|
||||
o.pos = UnityObjectToClipPos(v.vertex);
|
||||
o.normal = v.normal;
|
||||
|
||||
inline float BoundsDist(float3 p, float3 normal, float3 projNormal) {
|
||||
float3 q = abs(p - 0.5) - 0.5; // 1x1 square/cube centered at (0.5,0.5)
|
||||
//float dist = length(max(q,0)) + min(max(q.x,max(q.y,q.z)),0.0); // true SDF
|
||||
#ifdef DECAL_PREVIEW
|
||||
o.uv_decal = v.texcoord;
|
||||
return 10 * max(q.x, q.y); // 2D pseudo SDF
|
||||
#else
|
||||
o.uv_decal = mul (_ProjectionMatrix, v.vertex);
|
||||
float dist = max(max(q.x, q.y), q.z); // pseudo SDF
|
||||
float ndist = EDGE_MARGIN - dot(normal, projNormal); // SDF to normal
|
||||
return 10 * max(dist, ndist); // return intersection
|
||||
#endif //DECAL_PREVIEW
|
||||
|
||||
#ifdef DECAL_BASE_NORMAL
|
||||
o.uv_base = TRANSFORM_TEX(v.texcoord, _BumpMap);
|
||||
#endif //DECAL_BASE_NORMAL
|
||||
|
||||
float3 worldPosition = mul(unity_ObjectToWorld, v.vertex).xyz;
|
||||
float3 worldNormal = UnityObjectToWorldNormal(v.normal);
|
||||
|
||||
#if defined(DECAL_BASE_NORMAL) || defined(DECAL_PREVIEW)
|
||||
// use tangent of base geometry
|
||||
fixed3 worldTangent = UnityObjectToWorldDir(v.tangent.xyz);
|
||||
fixed tangentSign = v.tangent.w * unity_WorldTransformParams.w;
|
||||
fixed3 worldBinormal = cross(worldNormal, worldTangent) * tangentSign;
|
||||
#else
|
||||
// use tangent of projector
|
||||
fixed3 decalTangent = UnityObjectToWorldDir(_DecalTangent);
|
||||
fixed3 worldBinormal = cross(decalTangent, worldNormal);
|
||||
fixed3 worldTangent = cross(worldNormal, worldBinormal);
|
||||
#endif //defined(DECAL_BASE_NORMAL) || defined(DECAL_PREVIEW)
|
||||
|
||||
o.tSpace0 = float4(worldTangent.x, worldBinormal.x, worldNormal.x, worldPosition.x);
|
||||
o.tSpace1 = float4(worldTangent.y, worldBinormal.y, worldNormal.y, worldPosition.y);
|
||||
o.tSpace2 = float4(worldTangent.z, worldBinormal.z, worldNormal.z, worldPosition.z);
|
||||
|
||||
// forward base pass specific lighting code
|
||||
#ifdef UNITY_PASS_FORWARDBASE
|
||||
// SH/ambient light
|
||||
#if UNITY_SHOULD_SAMPLE_SH
|
||||
float3 shlight = ShadeSH9 (float4(worldNormal,1.0));
|
||||
o.vlight = shlight;
|
||||
#else
|
||||
o.vlight = 0.0;
|
||||
#endif // UNITY_SHOULD_SAMPLE_SH
|
||||
|
||||
// vertex light
|
||||
#ifdef VERTEXLIGHT_ON
|
||||
o.vlight += Shade4PointLights (
|
||||
unity_4LightPosX0, unity_4LightPosY0, unity_4LightPosZ0,
|
||||
unity_LightColor[0].rgb, unity_LightColor[1].rgb, unity_LightColor[2].rgb, unity_LightColor[3].rgb,
|
||||
unity_4LightAtten0, worldPosition, worldNormal );
|
||||
#endif // VERTEXLIGHT_ON
|
||||
#endif // UNITY_PASS_FORWARDBASE
|
||||
|
||||
// pass shadow and, possibly, light cookie coordinates to pixel shader
|
||||
UNITY_TRANSFER_LIGHTING(o, 0.0);
|
||||
|
||||
return o;
|
||||
}
|
||||
|
||||
fixed4 frag_forward(v2f IN) : SV_Target
|
||||
{
|
||||
// declare data
|
||||
DecalSurfaceInput i;
|
||||
SurfaceOutput o;
|
||||
fixed4 c = 0;
|
||||
|
||||
// setup world-space TBN vectors
|
||||
UNITY_EXTRACT_TBN(IN);
|
||||
|
||||
float3 worldPosition = float3(IN.tSpace0.w, IN.tSpace1.w, IN.tSpace2.w);
|
||||
float3 worldTangent = float3(IN.tSpace0.x, IN.tSpace1.x, IN.tSpace2.x);
|
||||
|
||||
// setup world-space light and view direction vectors
|
||||
#ifndef USING_DIRECTIONAL_LIGHT
|
||||
fixed3 lightDir = normalize(UnityWorldSpaceLightDir(worldPosition));
|
||||
#else
|
||||
fixed3 lightDir = _WorldSpaceLightPos0.xyz;
|
||||
#endif
|
||||
float3 worldViewDir = normalize(UnityWorldSpaceViewDir(worldPosition));
|
||||
float3 viewDir = _unity_tbn_0 * worldViewDir.x + _unity_tbn_1 * worldViewDir.y + _unity_tbn_2 * worldViewDir.z;
|
||||
|
||||
#ifdef DECAL_PREVIEW
|
||||
fixed4 uv_projected = IN.uv_decal;
|
||||
#else
|
||||
// perform decal projection
|
||||
fixed4 uv_projected = UNITY_PROJ_COORD(IN.uv_decal);
|
||||
|
||||
// clip texture outside of xyz bounds
|
||||
clip(uv_projected.xyz);
|
||||
clip(1-uv_projected.xyz);
|
||||
|
||||
// clip backsides
|
||||
clip(dot(_DecalNormal, IN.normal));
|
||||
#endif //DECAL_PREVIEW
|
||||
|
||||
// initialize surface input
|
||||
UNITY_INITIALIZE_OUTPUT(DecalSurfaceInput, i)
|
||||
i.uv_decal = TRANSFORM_TEX(uv_projected, _Decal);
|
||||
|
||||
#ifdef DECAL_NORMAL
|
||||
i.uv_bump = TRANSFORM_TEX(uv_projected, _DecalBumpMap);
|
||||
#endif //DECAL_NORMAL
|
||||
|
||||
#ifdef DECAL_SPECULAR
|
||||
i.uv_spec = TRANSFORM_TEX(uv_projected, _SpecMap);
|
||||
#endif //DECAL_SPECULAR
|
||||
|
||||
#ifdef DECAL_EMISSIVE
|
||||
i.uv_glow = TRANSFORM_TEX(uv_projected, _GlowMap);
|
||||
#endif //DECAL_EMISSIVE
|
||||
|
||||
#ifdef DECAL_BASE_NORMAL
|
||||
#ifdef DECAL_PREVIEW
|
||||
i.normal = fixed3(0,0,1);
|
||||
#else
|
||||
i.normal = UnpackNormalDXT5nm(tex2D(_BumpMap, IN.uv_base));
|
||||
#endif //DECAL_PREVIEW
|
||||
#endif //DECAL_BASE_NORMAL
|
||||
|
||||
//i.normal = IN.normal;
|
||||
i.viewDir = viewDir;
|
||||
i.worldPosition = worldPosition;
|
||||
|
||||
// initialize surface output
|
||||
o.Albedo = 0.0;
|
||||
o.Emission = 0.0;
|
||||
o.Specular = 0.0;
|
||||
o.Alpha = 0.0;
|
||||
o.Gloss = 0.0;
|
||||
o.Normal = fixed3(0,0,1);
|
||||
|
||||
// call surface function
|
||||
surf(i, o);
|
||||
|
||||
#ifdef DECAL_PREVIEW
|
||||
if (any(IN.uv_decal > 1) || any(IN.uv_decal < 0)) o.Alpha = 0;
|
||||
|
||||
o.Albedo = lerp(_Background.rgb, o.Albedo, o.Alpha) * _Color.rgb;
|
||||
o.Normal = lerp(float3(0,0,1), o.Normal, o.Alpha);
|
||||
o.Gloss = lerp(_Background.a, o.Gloss, o.Alpha);
|
||||
o.Emission = lerp(0, o.Emission, o.Alpha);
|
||||
o.Alpha = _Opacity;
|
||||
#endif //DECAL_PREVIEW
|
||||
|
||||
// compute lighting & shadowing factor
|
||||
UNITY_LIGHT_ATTENUATION(atten, IN, worldPosition)
|
||||
|
||||
// compute world normal
|
||||
float3 WorldNormal;
|
||||
WorldNormal.x = dot(_unity_tbn_0, o.Normal);
|
||||
WorldNormal.y = dot(_unity_tbn_1, o.Normal);
|
||||
WorldNormal.z = dot(_unity_tbn_2, o.Normal);
|
||||
WorldNormal = normalize(WorldNormal);
|
||||
o.Normal = WorldNormal;
|
||||
|
||||
//call modified KSP lighting function
|
||||
c += LightingBlinnPhongDecal(o, lightDir, worldViewDir, atten);
|
||||
|
||||
// Forward base emission and ambient/vertex lighting
|
||||
#ifdef UNITY_PASS_FORWARDBASE
|
||||
c.rgb += o.Emission;
|
||||
c.rgb += o.Albedo * IN.vlight;
|
||||
c.a = o.Alpha;
|
||||
#endif //UNITY_PASS_FORWARDBASE
|
||||
|
||||
// Forward add multiply by alpha
|
||||
#ifdef UNITY_PASS_FORWARDADD
|
||||
c.rgb *= o.Alpha;
|
||||
#endif
|
||||
|
||||
return c;
|
||||
}
|
||||
|
||||
#endif
|
||||
#endif
|
33
Assets/Shaders/DecalsLighting.cginc
Normal file
33
Assets/Shaders/DecalsLighting.cginc
Normal file
@ -0,0 +1,33 @@
|
||||
#ifndef DECALS_LIGHTING_INCLUDED
|
||||
#define DECALS_LIGHTING_INCLUDED
|
||||
|
||||
// modifed version of the KSP BlinnPhong because it does some weird things
|
||||
inline fixed4 LightingBlinnPhongDecal(SurfaceOutput s, fixed3 lightDir, half3 viewDir, fixed atten)
|
||||
{
|
||||
s.Normal = normalize(s.Normal);
|
||||
half3 h = normalize(lightDir + viewDir);
|
||||
|
||||
fixed diff = max(0, dot(s.Normal, lightDir));
|
||||
|
||||
float nh = max(0, dot(s.Normal, h));
|
||||
float spec = pow(nh, s.Specular*128.0) * s.Gloss;
|
||||
|
||||
fixed4 c = 0;
|
||||
c.rgb = (s.Albedo * _LightColor0.rgb * diff + _LightColor0.rgb * _SpecColor.rgb * spec) * (atten);
|
||||
return c;
|
||||
}
|
||||
|
||||
// KSP underwater fog function
|
||||
float4 UnderwaterFog(float3 worldPos, float3 color)
|
||||
{
|
||||
float3 toPixel = worldPos - _LocalCameraPos.xyz;
|
||||
float toPixelLength = length(toPixel);
|
||||
|
||||
float underwaterDetection = _UnderwaterFogFactor * _LocalCameraDir.w;
|
||||
float albedoLerpValue = underwaterDetection * (_UnderwaterMaxAlbedoFog * saturate(toPixelLength * _UnderwaterAlbedoDistanceScalar));
|
||||
float alphaFactor = 1 - underwaterDetection * (_UnderwaterMaxAlphaFog * saturate((toPixelLength - _UnderwaterMinAlphaFogDistance) * _UnderwaterAlphaDistanceScalar));
|
||||
|
||||
return float4(lerp(color, _UnderwaterFogColor.rgb, albedoLerpValue), alphaFactor);
|
||||
}
|
||||
|
||||
#endif
|
183
Assets/Shaders/DecalsSurface.cginc
Normal file
183
Assets/Shaders/DecalsSurface.cginc
Normal file
@ -0,0 +1,183 @@
|
||||
#ifndef DECALS_SURFACE_INCLUDED
|
||||
#define DECALS_SURFACE_INCLUDED
|
||||
|
||||
#include "DecalsCommon.cginc"
|
||||
#include "DecalsLighting.cginc"
|
||||
|
||||
// declare surf function,
|
||||
// this must be defined in any shader using this cginc
|
||||
void surf (DecalSurfaceInput IN, inout SurfaceOutput o);
|
||||
|
||||
v2f vert_forward(appdata_decal v)
|
||||
{
|
||||
v2f o;
|
||||
UNITY_INITIALIZE_OUTPUT(v2f,o);
|
||||
|
||||
o.pos = UnityObjectToClipPos(v.vertex);
|
||||
o.normal = v.normal;
|
||||
|
||||
#ifdef DECAL_PREVIEW
|
||||
o.uv_decal = v.texcoord;
|
||||
#else
|
||||
o.uv_decal = mul (_ProjectionMatrix, v.vertex);
|
||||
#endif //DECAL_PREVIEW
|
||||
|
||||
#ifdef DECAL_BASE_NORMAL
|
||||
o.uv_base = TRANSFORM_TEX(v.texcoord, _BumpMap);
|
||||
#endif //DECAL_BASE_NORMAL
|
||||
|
||||
float3 worldPosition = mul(unity_ObjectToWorld, v.vertex).xyz;
|
||||
float3 worldNormal = UnityObjectToWorldNormal(v.normal);
|
||||
|
||||
#if defined(DECAL_BASE_NORMAL) || defined(DECAL_PREVIEW)
|
||||
// use tangent of base geometry
|
||||
fixed3 worldTangent = UnityObjectToWorldDir(v.tangent.xyz);
|
||||
fixed tangentSign = v.tangent.w * unity_WorldTransformParams.w;
|
||||
fixed3 worldBinormal = cross(worldNormal, worldTangent) * tangentSign;
|
||||
#else
|
||||
// use tangent of projector
|
||||
fixed3 decalTangent = UnityObjectToWorldDir(_DecalTangent);
|
||||
fixed3 worldBinormal = cross(decalTangent, worldNormal);
|
||||
fixed3 worldTangent = cross(worldNormal, worldBinormal);
|
||||
#endif //defined(DECAL_BASE_NORMAL) || defined(DECAL_PREVIEW)
|
||||
|
||||
o.tSpace0 = float4(worldTangent.x, worldBinormal.x, worldNormal.x, worldPosition.x);
|
||||
o.tSpace1 = float4(worldTangent.y, worldBinormal.y, worldNormal.y, worldPosition.y);
|
||||
o.tSpace2 = float4(worldTangent.z, worldBinormal.z, worldNormal.z, worldPosition.z);
|
||||
|
||||
// forward base pass specific lighting code
|
||||
#ifdef UNITY_PASS_FORWARDBASE
|
||||
// SH/ambient light
|
||||
#if UNITY_SHOULD_SAMPLE_SH
|
||||
float3 shlight = ShadeSH9 (float4(worldNormal,1.0));
|
||||
o.vlight = shlight;
|
||||
#else
|
||||
o.vlight = 0.0;
|
||||
#endif // UNITY_SHOULD_SAMPLE_SH
|
||||
|
||||
// vertex light
|
||||
#ifdef VERTEXLIGHT_ON
|
||||
o.vlight += Shade4PointLights (
|
||||
unity_4LightPosX0, unity_4LightPosY0, unity_4LightPosZ0,
|
||||
unity_LightColor[0].rgb, unity_LightColor[1].rgb, unity_LightColor[2].rgb, unity_LightColor[3].rgb,
|
||||
unity_4LightAtten0, worldPosition, worldNormal );
|
||||
#endif // VERTEXLIGHT_ON
|
||||
#endif // UNITY_PASS_FORWARDBASE
|
||||
|
||||
// pass shadow and, possibly, light cookie coordinates to pixel shader
|
||||
UNITY_TRANSFER_LIGHTING(o, 0.0);
|
||||
|
||||
return o;
|
||||
}
|
||||
|
||||
fixed4 frag_forward(v2f IN) : SV_Target
|
||||
{
|
||||
#ifdef DECAL_PREVIEW
|
||||
fixed4 uv_projected = IN.uv_decal;
|
||||
#else
|
||||
// perform decal projection
|
||||
fixed4 uv_projected = UNITY_PROJ_COORD(IN.uv_decal);
|
||||
|
||||
clip(uv_projected.xyz + CLIP_MARGIN);
|
||||
clip(CLIP_MARGIN + (1-uv_projected.xyz));
|
||||
#endif //DECAL_PREVIEW
|
||||
|
||||
// declare data
|
||||
DecalSurfaceInput i;
|
||||
SurfaceOutput o;
|
||||
|
||||
// setup world-space TBN vectors
|
||||
UNITY_EXTRACT_TBN(IN);
|
||||
|
||||
float3 worldPosition = float3(IN.tSpace0.w, IN.tSpace1.w, IN.tSpace2.w);
|
||||
float3 worldTangent = float3(IN.tSpace0.x, IN.tSpace1.x, IN.tSpace2.x);
|
||||
|
||||
// setup world-space light and view direction vectors
|
||||
#ifndef USING_DIRECTIONAL_LIGHT
|
||||
fixed3 lightDir = normalize(UnityWorldSpaceLightDir(worldPosition));
|
||||
#else
|
||||
fixed3 lightDir = _WorldSpaceLightPos0.xyz;
|
||||
#endif
|
||||
float3 worldViewDir = normalize(UnityWorldSpaceViewDir(worldPosition));
|
||||
float3 viewDir = _unity_tbn_0 * worldViewDir.x + _unity_tbn_1 * worldViewDir.y + _unity_tbn_2 * worldViewDir.z;
|
||||
|
||||
// initialize surface input
|
||||
UNITY_INITIALIZE_OUTPUT(DecalSurfaceInput, i)
|
||||
i.uv_decal = TRANSFORM_TEX(uv_projected, _Decal);
|
||||
i.uv = uv_projected;
|
||||
|
||||
#ifdef DECAL_BUMPMAP
|
||||
i.uv_bumpmap = TRANSFORM_TEX(uv_projected, _BumpMap);
|
||||
#endif //DECAL_BUMPMAP
|
||||
|
||||
#ifdef DECAL_SPECMAP
|
||||
i.uv_specmap = TRANSFORM_TEX(uv_projected, _SpecMap);
|
||||
#endif //DECAL_SPECMAP
|
||||
|
||||
#ifdef DECAL_EMISSIVE
|
||||
i.uv_emissive = TRANSFORM_TEX(uv_projected, _Emissive);
|
||||
#endif //DECAL_EMISSIVE
|
||||
|
||||
#ifdef DECAL_BASE_NORMAL
|
||||
#ifdef DECAL_PREVIEW
|
||||
i.normal = fixed3(0,0,1);
|
||||
#else
|
||||
i.normal = UnpackNormalDXT5nm(tex2D(_BumpMap, IN.uv_base));
|
||||
#endif //DECAL_PREVIEW
|
||||
#endif //DECAL_BASE_NORMAL
|
||||
|
||||
i.vertex_normal = IN.normal;
|
||||
i.viewDir = viewDir;
|
||||
i.worldPosition = worldPosition;
|
||||
|
||||
// initialize surface output
|
||||
o.Albedo = 0.0;
|
||||
o.Emission = 0.0;
|
||||
o.Specular = 0.0;
|
||||
o.Alpha = 0.0;
|
||||
o.Gloss = 0.0;
|
||||
o.Normal = fixed3(0,0,1);
|
||||
|
||||
// call surface function
|
||||
surf(i, o);
|
||||
|
||||
#ifdef DECAL_PREVIEW
|
||||
if (any(IN.uv_decal > 1) || any(IN.uv_decal < 0)) o.Alpha = 0;
|
||||
|
||||
o.Albedo = lerp(_Background.rgb, o.Albedo, o.Alpha) * _Color.rgb;
|
||||
o.Normal = lerp(float3(0,0,1), o.Normal, o.Alpha);
|
||||
o.Gloss = lerp(_Background.a, o.Gloss, o.Alpha);
|
||||
o.Emission = lerp(0, o.Emission, o.Alpha);
|
||||
o.Alpha = _Opacity;
|
||||
#endif //DECAL_PREVIEW
|
||||
|
||||
// compute lighting & shadowing factor
|
||||
UNITY_LIGHT_ATTENUATION(atten, IN, worldPosition)
|
||||
|
||||
// compute world normal
|
||||
float3 WorldNormal;
|
||||
WorldNormal.x = dot(_unity_tbn_0, o.Normal);
|
||||
WorldNormal.y = dot(_unity_tbn_1, o.Normal);
|
||||
WorldNormal.z = dot(_unity_tbn_2, o.Normal);
|
||||
WorldNormal = normalize(WorldNormal);
|
||||
o.Normal = WorldNormal;
|
||||
|
||||
//call modified KSP lighting function
|
||||
float4 c = LightingBlinnPhongDecal(o, lightDir, worldViewDir, atten);
|
||||
|
||||
// Forward base emission and ambient/vertex lighting
|
||||
#ifdef UNITY_PASS_FORWARDBASE
|
||||
c.rgb += o.Emission;
|
||||
c.rgb += o.Albedo * IN.vlight;
|
||||
c.a = o.Alpha;
|
||||
#endif //UNITY_PASS_FORWARDBASE
|
||||
|
||||
// Forward add multiply by alpha
|
||||
#ifdef UNITY_PASS_FORWARDADD
|
||||
c.rgb *= o.Alpha;
|
||||
#endif
|
||||
|
||||
return c;
|
||||
}
|
||||
|
||||
#endif
|
17
Assets/Shaders/HSL.cginc
Normal file
17
Assets/Shaders/HSL.cginc
Normal file
@ -0,0 +1,17 @@
|
||||
#ifndef HSL_INCLUDED
|
||||
#define HSL_INCLUDED
|
||||
|
||||
inline float3 HSL2RGB(float3 hsl) {
|
||||
int3 n = int3(0, 8, 4);
|
||||
float3 k = (n + hsl.x * 12) % 12;
|
||||
float a = hsl.y * min(hsl.z, 1 - hsl.z);
|
||||
return hsl.z - a * max(-1, min(k - 3, min(9 - k, 1)));
|
||||
}
|
||||
|
||||
inline float3 HSV2RGB(float3 hsv) {
|
||||
int3 n = int3(5, 3, 1);
|
||||
float3 k = (n + hsv.x * 6) % 6;
|
||||
return hsv.z - hsv.z * hsv.y * max(0, min(1, min(k, 4 - k)));
|
||||
}
|
||||
|
||||
#endif
|
45
Assets/Shaders/SDF.cginc
Normal file
45
Assets/Shaders/SDF.cginc
Normal file
@ -0,0 +1,45 @@
|
||||
#ifndef SDF_INCLUDED
|
||||
#define SDF_INCLUDED
|
||||
|
||||
// based on functions by Inigo Quilez
|
||||
// https://iquilezles.org/www/articles/distfunctions2d/distfunctions2d.htm
|
||||
|
||||
// SDF of a box
|
||||
float sdBox( in float2 p, in float2 b ) {
|
||||
float2 d = abs(p)-b;
|
||||
return length(max(d,0.0)) + min(max(d.x,d.y),0.0);
|
||||
}
|
||||
|
||||
// SDF of a box with corner radius r
|
||||
float sdRoundedBox( in float2 p, in float2 b, in float r ) {
|
||||
float2 d = abs(p)-b+r;
|
||||
return min(max(d.x,d.y),0.0) + length(max(d,0.0)) - r;
|
||||
}
|
||||
|
||||
// SDF of a box with corner radius r, based on the current UV position
|
||||
// UV must be ∈ (0,1), with (0,0) on one corner
|
||||
float sdRoundedUVBox( float2 uv, float r ) {
|
||||
float dx = ddx(uv.x);
|
||||
float dy = ddy(uv.y);
|
||||
|
||||
float2 dim = abs(float2(1/dx, 1/dy));
|
||||
float2 halfDim = dim / 2;
|
||||
float2 pos = (dim * uv) - halfDim;
|
||||
|
||||
return sdRoundedBox(pos, halfDim, r);
|
||||
}
|
||||
|
||||
inline float SDFdDist(float dist) {
|
||||
return length(float2(ddx(dist), ddy(dist)));
|
||||
}
|
||||
|
||||
inline float SDFAA(float dist, float ddist) {
|
||||
float pixelDist = dist / ddist;
|
||||
return saturate(0.5-pixelDist);
|
||||
}
|
||||
|
||||
inline float SDFAA(float dist) {
|
||||
return SDFAA(dist, SDFdDist(dist));
|
||||
}
|
||||
|
||||
#endif
|
38
Assets/Shaders/StandardDecal.cginc
Normal file
38
Assets/Shaders/StandardDecal.cginc
Normal file
@ -0,0 +1,38 @@
|
||||
void surf(DecalSurfaceInput IN, inout SurfaceOutput o) {
|
||||
float4 color = tex2D(_Decal, IN.uv_decal);
|
||||
o.Albedo = UnderwaterFog(IN.worldPosition, color).rgb;
|
||||
o.Alpha = _DecalOpacity;
|
||||
|
||||
#ifdef DECAL_BASE_NORMAL
|
||||
float3 normal = IN.normal;
|
||||
float wearFactor = 1 - normal.z;
|
||||
float wearFactorAlpha = saturate(_EdgeWearStrength * wearFactor);
|
||||
o.Alpha *= saturate(1 + _EdgeWearOffset - saturate(_EdgeWearStrength * wearFactor));
|
||||
#endif
|
||||
|
||||
#ifdef DECAL_BUMPMAP
|
||||
o.Normal = tex2D(_BumpMap, IN.uv_bumpmap);
|
||||
#endif
|
||||
|
||||
#ifdef DECAL_SPECMAP
|
||||
float4 specular = tex2D(_SpecMap, IN.uv_specmap);
|
||||
o.Gloss = specular.r;
|
||||
o.Specular = _Shininess;
|
||||
#endif
|
||||
|
||||
half rim = 1.0 - saturate(dot(normalize(IN.viewDir), o.Normal));
|
||||
o.Emission = (_RimColor.rgb * pow(rim, _RimFalloff)) * _RimColor.a;
|
||||
|
||||
#ifdef DECAL_EMISSIVE
|
||||
o.Emission += tex2D(_Emissive, IN.uv_emissive).rgb * _Emissive_Color.rgb * _Emissive_Color.a;
|
||||
#endif
|
||||
|
||||
float dist = BoundsDist(IN.uv, IN.vertex_normal, _DecalNormal);
|
||||
#ifdef DECAL_SDF_ALPHA
|
||||
float decalDist = _Cutoff - color.a;
|
||||
o.Alpha *= SDFAA(max(decalDist, dist));
|
||||
#else
|
||||
o.Alpha *= SDFAA(dist);
|
||||
o.Alpha *= color.a;
|
||||
#endif
|
||||
}
|
111
Assets/Shaders/StandardDecal.shader
Normal file
111
Assets/Shaders/StandardDecal.shader
Normal file
@ -0,0 +1,111 @@
|
||||
Shader "ConformalDecals/Decal/Standard"
|
||||
{
|
||||
Properties
|
||||
{
|
||||
[Header(Decal)]
|
||||
_Decal("Decal Texture", 2D) = "gray" {}
|
||||
[Toggle(DECAL_SDF_ALPHA)] _Decal_SDF_Alpha ("SDF in Alpha", int) = 0
|
||||
|
||||
|
||||
[Header(Normal)]
|
||||
[Toggle(DECAL_BASE_NORMAL)] _BaseNormal ("Use Base Normal", int) = 0
|
||||
[Toggle(DECAL_BUMPMAP)] _Decal_BumpMap ("Has BumpMap", int) = 0
|
||||
_BumpMap("Bump Map", 2D) = "bump" {}
|
||||
_EdgeWearStrength("Edge Wear Strength", Range(0,500)) = 100
|
||||
_EdgeWearOffset("Edge Wear Offset", Range(0,1)) = 0.1
|
||||
|
||||
[Header(Specularity)]
|
||||
[Toggle(DECAL_SPECMAP)] _Decal_SpecMap ("Has SpecMap", int) = 0
|
||||
_SpecMap ("Specular Map)", 2D) = "black" {}
|
||||
_SpecColor ("_SpecColor", Color) = (0.25, 0.25, 0.25, 1)
|
||||
_Shininess ("Shininess", Range (0.03, 10)) = 0.3
|
||||
|
||||
[Header(Emissive)]
|
||||
[Toggle(DECAL_EMISSIVE)] _Decal_Emissive ("Has Emissive", int) = 0
|
||||
_Emissive("_Emissive", 2D) = "black" {}
|
||||
_EmissiveColor("_EmissiveColor", Color) = (0,0,0,1)
|
||||
|
||||
_Cutoff ("Alpha cutoff", Range(0,1)) = 0.5
|
||||
_DecalOpacity("Opacity", Range(0,1) ) = 1
|
||||
_Background("Background Color", Color) = (0.9,0.9,0.9,0.7)
|
||||
|
||||
[Enum(UnityEngine.Rendering.CullMode)] _Cull ("Cull", int) = 2
|
||||
[Toggle] _ZWrite ("ZWrite", Float) = 1.0
|
||||
|
||||
[Toggle(DECAL_PREVIEW)] _Preview ("Preview", int) = 0
|
||||
|
||||
[Header(Effects)]
|
||||
[PerRendererData]_Opacity("_Opacity", Range(0,1) ) = 1
|
||||
[PerRendererData]_Color("_Color", Color) = (1,1,1,1)
|
||||
[PerRendererData]_RimFalloff("_RimFalloff", Range(0.01,5) ) = 0.1
|
||||
[PerRendererData]_RimColor("_RimColor", Color) = (0,0,0,0)
|
||||
[PerRendererData]_UnderwaterFogFactor ("Underwater Fog Factor", Range(0,1)) = 0
|
||||
}
|
||||
SubShader
|
||||
{
|
||||
Tags { "Queue" = "Geometry+100" "IgnoreProjector" = "true" "DisableBatching" = "true"}
|
||||
Cull [_Cull]
|
||||
|
||||
Pass
|
||||
{
|
||||
Name "FORWARD"
|
||||
Tags { "LightMode" = "ForwardBase" }
|
||||
ZWrite [_ZWrite]
|
||||
ZTest LEqual
|
||||
Blend SrcAlpha OneMinusSrcAlpha
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert_forward
|
||||
#pragma fragment frag_forward
|
||||
|
||||
#pragma multi_compile_fwdbase nolightmap nodirlightmap nodynlightmap
|
||||
#pragma skip_variants SHADOWS_DEPTH SHADOWS_CUBE SHADOWS_SHADOWMASK LIGHTMAP_SHADOW_MIXING POINT_COOKIE
|
||||
#pragma multi_compile_local __ DECAL_PREVIEW
|
||||
#pragma multi_compile_local __ DECAL_BASE_NORMAL DECAL_BUMPMAP
|
||||
#pragma multi_compile_local __ DECAL_SPECMAP
|
||||
#pragma multi_compile_local __ DECAL_EMISSIVE
|
||||
#pragma multi_compile_local __ DECAL_SDF_ALPHA
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "DecalsCommon.cginc"
|
||||
#include "DecalsSurface.cginc"
|
||||
#include "SDF.cginc"
|
||||
#include "StandardDecal.cginc"
|
||||
|
||||
ENDCG
|
||||
}
|
||||
|
||||
Pass
|
||||
{
|
||||
Name "FORWARD"
|
||||
Tags { "LightMode" = "ForwardAdd" }
|
||||
ZWrite Off
|
||||
ZTest LEqual
|
||||
Blend One One
|
||||
Offset -1, -1
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert_forward
|
||||
#pragma fragment frag_forward
|
||||
|
||||
#pragma multi_compile_fwdadd nolightmap nodirlightmap nodynlightmap
|
||||
#pragma skip_variants SHADOWS_DEPTH SHADOWS_CUBE SHADOWS_SHADOWMASK LIGHTMAP_SHADOW_MIXING POINT_COOKIE
|
||||
#pragma multi_compile_local __ DECAL_PREVIEW
|
||||
#pragma multi_compile_local __ DECAL_BASE_NORMAL DECAL_BUMPMAP
|
||||
#pragma multi_compile_local __ DECAL_SPECMAP
|
||||
#pragma multi_compile_local __ DECAL_EMISSIVE
|
||||
#pragma multi_compile_local __ DECAL_SDF_ALPHA
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "DecalsCommon.cginc"
|
||||
#include "DecalsSurface.cginc"
|
||||
#include "SDF.cginc"
|
||||
#include "StandardDecal.cginc"
|
||||
|
||||
ENDCG
|
||||
}
|
||||
|
||||
// shadow casting support
|
||||
UsePass "Legacy Shaders/VertexLit/SHADOWCASTER"
|
||||
}
|
||||
}
|
73
Assets/Shaders/TextBlit.shader
Normal file
73
Assets/Shaders/TextBlit.shader
Normal file
@ -0,0 +1,73 @@
|
||||
Shader "ConformalDecals/Text Blit"
|
||||
{
|
||||
Properties
|
||||
{
|
||||
_MainTex("_MainTex (RGB spec(A))", 2D) = "white" {}
|
||||
|
||||
_WeightNormal("Weight Normal", float) = 0
|
||||
_WeightBold("Weight Bold", float) = 0.5
|
||||
|
||||
_ScaleRatioA("Scale RatioA", float) = 1
|
||||
_ScaleRatioB("Scale RatioB", float) = 1
|
||||
_ScaleRatioC("Scale RatioC", float) = 1
|
||||
}
|
||||
|
||||
SubShader
|
||||
{
|
||||
Tags { "Queue" = "Transparent" }
|
||||
Cull Off
|
||||
ZWrite Off
|
||||
|
||||
Pass
|
||||
{
|
||||
BlendOp Max
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert
|
||||
#pragma fragment frag
|
||||
|
||||
sampler2D _MainTex;
|
||||
|
||||
// font weights to fake bold
|
||||
float _WeightNormal;
|
||||
float _WeightBold;
|
||||
|
||||
// no idea what these do
|
||||
float _ScaleRatioA;
|
||||
float _ScaleRatioB;
|
||||
float _ScaleRatioC;
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "Lighting.cginc"
|
||||
#include "AutoLight.cginc"
|
||||
|
||||
struct v2f {
|
||||
float4 pos : SV_POSITION;
|
||||
float4 uv : TEXCOORD0; // u, v, bias, 0
|
||||
};
|
||||
|
||||
v2f vert(float4 vertex : POSITION, float2 uv0 : TEXCOORD0, float2 uv1 : TEXCOORD1) {
|
||||
float bold = step(uv1.y, 0);
|
||||
float weight = lerp(_WeightNormal, _WeightBold, bold) * _ScaleRatioA / 8.0;
|
||||
float bias = 1 - weight;
|
||||
|
||||
v2f o;
|
||||
o.pos = UnityObjectToClipPos(vertex);
|
||||
o.uv = float4(uv0.x, uv0.y, bias, weight);
|
||||
return o;
|
||||
}
|
||||
|
||||
fixed4 frag (v2f i) : SV_Target {
|
||||
float2 uv = i.uv.xy;
|
||||
float bias = i.uv.z;
|
||||
float weight = i.uv.w;
|
||||
|
||||
fixed4 c = 0;
|
||||
c.r = saturate(tex2D(_MainTex,(uv)).a + weight);
|
||||
return c;
|
||||
}
|
||||
|
||||
ENDCG
|
||||
}
|
||||
}
|
||||
}
|
49
Assets/Shaders/TextDecal.cginc
Normal file
49
Assets/Shaders/TextDecal.cginc
Normal file
@ -0,0 +1,49 @@
|
||||
float4 _DecalColor;
|
||||
|
||||
float4 _OutlineColor;
|
||||
float _OutlineWidth;
|
||||
|
||||
void surf(DecalSurfaceInput IN, inout SurfaceOutput o) {
|
||||
float4 color = _DecalColor;
|
||||
float dist = _Cutoff - tex2D(_Decal, IN.uv_decal).r; // text distance
|
||||
|
||||
#ifdef DECAL_OUTLINE
|
||||
// Outline
|
||||
float outlineOffset = _OutlineWidth * 0.25;
|
||||
float outlineRadius = _OutlineWidth * 0.5;
|
||||
|
||||
#ifdef DECAL_FILL
|
||||
// Outline and Fill
|
||||
float outlineDist = -dist - outlineOffset;
|
||||
float outlineFactor = SDFAA(outlineDist);
|
||||
dist -= outlineOffset;
|
||||
color = lerp(_DecalColor, _OutlineColor, outlineFactor);
|
||||
#else
|
||||
// Outline Only
|
||||
float outlineDist = abs(dist) - outlineOffset;
|
||||
dist = outlineDist;
|
||||
color = _OutlineColor;
|
||||
#endif
|
||||
#endif
|
||||
|
||||
dist = max(dist, BoundsDist(IN.uv, IN.vertex_normal, _DecalNormal));
|
||||
float ddist = SDFdDist(dist); // distance gradient magnitude
|
||||
o.Alpha = _DecalOpacity * SDFAA(dist, ddist);
|
||||
o.Albedo = UnderwaterFog(IN.worldPosition, color).rgb;
|
||||
|
||||
#ifdef DECAL_BASE_NORMAL
|
||||
float3 normal = IN.normal;
|
||||
float wearFactor = 1 - normal.z;
|
||||
float wearFactorAlpha = saturate(_EdgeWearStrength * wearFactor);
|
||||
o.Alpha *= saturate(1 + _EdgeWearOffset - saturate(_EdgeWearStrength * wearFactor));
|
||||
#endif
|
||||
|
||||
#ifdef DECAL_SPECMAP
|
||||
float4 specular = tex2D(_SpecMap, IN.uv_specmap);
|
||||
o.Gloss = specular.r;
|
||||
o.Specular = _Shininess;
|
||||
#endif
|
||||
|
||||
half rim = 1.0 - saturate(dot(normalize(IN.viewDir), o.Normal));
|
||||
o.Emission = (_RimColor.rgb * pow(rim, _RimFalloff)) * _RimColor.a;
|
||||
}
|
112
Assets/Shaders/TextDecal.shader
Normal file
112
Assets/Shaders/TextDecal.shader
Normal file
@ -0,0 +1,112 @@
|
||||
Shader "ConformalDecals/Decal/Text"
|
||||
{
|
||||
Properties
|
||||
{
|
||||
[Header(Decal)]
|
||||
[Toggle(DECAL_FILL)] _Fill ("Fill", int) = 0
|
||||
_Decal("Decal Texture", 2D) = "gray" {}
|
||||
_DecalColor("Decal Color", Color) = (1,1,1,1)
|
||||
|
||||
_Weight("Text Weight", Range(0,1)) = 0
|
||||
|
||||
[Header(Outline)]
|
||||
[Toggle(DECAL_OUTLINE)] _Outline ("Outline", int) = 0
|
||||
_OutlineColor("Outline Color", Color) = (0,0,0,1)
|
||||
_OutlineWidth("Outline Width", Range(0,1)) = 0.1
|
||||
|
||||
[Header(Normal)]
|
||||
[Toggle(DECAL_BASE_NORMAL)] _BaseNormal ("Use Base Normal", int) = 0
|
||||
_BumpMap("Bump Map", 2D) = "bump" {}
|
||||
_EdgeWearStrength("Edge Wear Strength", Range(0,500)) = 100
|
||||
_EdgeWearOffset("Edge Wear Offset", Range(0,1)) = 0.1
|
||||
|
||||
[Header(Specularity)]
|
||||
[Toggle(DECAL_SPECMAP)] _Decal_SpecMap ("Has SpecMap", int) = 0
|
||||
_SpecMap ("Specular Map)", 2D) = "black" {}
|
||||
_SpecColor ("_SpecColor", Color) = (0.25, 0.25, 0.25, 1)
|
||||
_Shininess ("Shininess", Range (0.03, 10)) = 0.3
|
||||
|
||||
_Cutoff ("Alpha cutoff", Range(0,1)) = 0.5
|
||||
_DecalOpacity("Opacity", Range(0,1) ) = 1
|
||||
_Background("Background Color", Color) = (0.9,0.9,0.9,0.7)
|
||||
|
||||
[Enum(UnityEngine.Rendering.CullMode)] _Cull ("Cull", int) = 2
|
||||
[Toggle] _ZWrite ("ZWrite", Float) = 1.0
|
||||
|
||||
[Toggle(DECAL_PREVIEW)] _Preview ("Preview", int) = 0
|
||||
|
||||
[Header(Effects)]
|
||||
[PerRendererData]_Opacity("_Opacity", Range(0,1) ) = 1
|
||||
_Color("_Color", Color) = (1,1,1,1)
|
||||
[PerRendererData]_RimFalloff("_RimFalloff", Range(0.01,5) ) = 0.1
|
||||
[PerRendererData]_RimColor("_RimColor", Color) = (0,0,0,0)
|
||||
[PerRendererData]_UnderwaterFogFactor ("Underwater Fog Factor", Range(0,1)) = 0
|
||||
}
|
||||
SubShader
|
||||
{
|
||||
Tags { "Queue" = "Geometry+100" "IgnoreProjector" = "true" "DisableBatching" = "true"}
|
||||
Cull [_Cull]
|
||||
|
||||
Pass
|
||||
{
|
||||
Name "FORWARD"
|
||||
Tags { "LightMode" = "ForwardBase" }
|
||||
ZWrite [_ZWrite]
|
||||
ZTest LEqual
|
||||
Blend SrcAlpha OneMinusSrcAlpha
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert_forward
|
||||
#pragma fragment frag_forward
|
||||
|
||||
#pragma multi_compile_fwdbase nolightmap nodirlightmap nodynlightmap
|
||||
#pragma skip_variants SHADOWS_DEPTH SHADOWS_CUBE SHADOWS_SHADOWMASK LIGHTMAP_SHADOW_MIXING POINT_COOKIE
|
||||
#pragma multi_compile_local __ DECAL_PREVIEW
|
||||
#pragma multi_compile_local __ DECAL_BASE_NORMAL
|
||||
#pragma multi_compile_local __ DECAL_SPECMAP
|
||||
#pragma multi_compile_local __ DECAL_OUTLINE
|
||||
#pragma multi_compile_local __ DECAL_FILL
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "DecalsCommon.cginc"
|
||||
#include "DecalsSurface.cginc"
|
||||
#include "SDF.cginc"
|
||||
#include "TextDecal.cginc"
|
||||
|
||||
ENDCG
|
||||
}
|
||||
|
||||
Pass
|
||||
{
|
||||
Name "FORWARD"
|
||||
Tags { "LightMode" = "ForwardAdd" }
|
||||
ZWrite Off
|
||||
ZTest LEqual
|
||||
Blend One One
|
||||
Offset -1, -1
|
||||
|
||||
CGPROGRAM
|
||||
#pragma vertex vert_forward
|
||||
#pragma fragment frag_forward
|
||||
|
||||
#pragma multi_compile_fwdadd nolightmap nodirlightmap nodynlightmap
|
||||
#pragma skip_variants SHADOWS_DEPTH SHADOWS_CUBE SHADOWS_SHADOWMASK LIGHTMAP_SHADOW_MIXING POINT_COOKIE
|
||||
#pragma multi_compile_local __ DECAL_PREVIEW
|
||||
#pragma multi_compile_local __ DECAL_BASE_NORMAL
|
||||
#pragma multi_compile_local __ DECAL_SPECMAP
|
||||
#pragma multi_compile_local __ DECAL_OUTLINE
|
||||
#pragma multi_compile_local __ DECAL_FILL
|
||||
|
||||
#include "UnityCG.cginc"
|
||||
#include "DecalsCommon.cginc"
|
||||
#include "DecalsSurface.cginc"
|
||||
#include "SDF.cginc"
|
||||
#include "TextDecal.cginc"
|
||||
|
||||
ENDCG
|
||||
}
|
||||
|
||||
// shadow casting support
|
||||
UsePass "Legacy Shaders/VertexLit/SHADOWCASTER"
|
||||
}
|
||||
}
|
BIN
Assets/Textures/Munar-Atlas.png
Normal file
BIN
Assets/Textures/Munar-Atlas.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 470 KiB |
BIN
Assets/Textures/UI.png
Normal file
BIN
Assets/Textures/UI.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 6.2 KiB |
93
Assets/UI/BSlider.mat
Normal file
93
Assets/UI/BSlider.mat
Normal file
@ -0,0 +1,93 @@
|
||||
%YAML 1.1
|
||||
%TAG !u! tag:unity3d.com,2011:
|
||||
--- !u!21 &2100000
|
||||
Material:
|
||||
serializedVersion: 6
|
||||
m_ObjectHideFlags: 0
|
||||
m_CorrespondingSourceObject: {fileID: 0}
|
||||
m_PrefabInstance: {fileID: 0}
|
||||
m_PrefabAsset: {fileID: 0}
|
||||
m_Name: BSlider
|
||||
m_Shader: {fileID: 4800000, guid: a6b2542ba8ea844e7b0526fab69d88ed, type: 3}
|
||||
m_ShaderKeywords: BLUE
|
||||
m_LightmapFlags: 4
|
||||
m_EnableInstancingVariants: 0
|
||||
m_DoubleSidedGI: 0
|
||||
m_CustomRenderQueue: -1
|
||||
stringTagMap: {}
|
||||
disabledShaderPasses: []
|
||||
m_SavedProperties:
|
||||
serializedVersion: 3
|
||||
m_TexEnvs:
|
||||
- _BumpMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailAlbedoMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailMask:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailNormalMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _EmissionMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _MainTex:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _MetallicGlossMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _OcclusionMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _ParallaxMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
m_Floats:
|
||||
- _Blue: 1
|
||||
- _BumpScale: 1
|
||||
- _ColorMask: 15
|
||||
- _Cutoff: 0.5
|
||||
- _DetailNormalMapScale: 1
|
||||
- _DstBlend: 0
|
||||
- _GlossMapScale: 1
|
||||
- _Glossiness: 0.5
|
||||
- _GlossyReflections: 1
|
||||
- _GradientStep: 0.331
|
||||
- _Green: 0
|
||||
- _Hue: 0
|
||||
- _Metallic: 0
|
||||
- _Mode: 0
|
||||
- _OcclusionStrength: 1
|
||||
- _OutlineGradient: 0.7
|
||||
- _OutlineOpacity: 0.15
|
||||
- _OutlineWidth: 2.5
|
||||
- _Parallax: 0.02
|
||||
- _Radius: 4
|
||||
- _Red: 0
|
||||
- _SmoothnessTextureChannel: 0
|
||||
- _SpecularHighlights: 1
|
||||
- _SrcBlend: 1
|
||||
- _Stencil: 0
|
||||
- _StencilComp: 8
|
||||
- _StencilOp: 0
|
||||
- _StencilReadMask: 255
|
||||
- _StencilWriteMask: 255
|
||||
- _UVSec: 0
|
||||
- _UseUIAlphaClip: 0
|
||||
- _ZWrite: 1
|
||||
m_Colors:
|
||||
- _Color: {r: 0, g: 0, b: 0, a: 1}
|
||||
- _EmissionColor: {r: 0, g: 0, b: 0, a: 1}
|
5492
Assets/UI/ColorPickerPanel.prefab
Normal file
5492
Assets/UI/ColorPickerPanel.prefab
Normal file
File diff suppressed because it is too large
Load Diff
1048
Assets/UI/FontMenuPanel.prefab
Normal file
1048
Assets/UI/FontMenuPanel.prefab
Normal file
File diff suppressed because it is too large
Load Diff
93
Assets/UI/GSlider.mat
Normal file
93
Assets/UI/GSlider.mat
Normal file
@ -0,0 +1,93 @@
|
||||
%YAML 1.1
|
||||
%TAG !u! tag:unity3d.com,2011:
|
||||
--- !u!21 &2100000
|
||||
Material:
|
||||
serializedVersion: 6
|
||||
m_ObjectHideFlags: 0
|
||||
m_CorrespondingSourceObject: {fileID: 0}
|
||||
m_PrefabInstance: {fileID: 0}
|
||||
m_PrefabAsset: {fileID: 0}
|
||||
m_Name: GSlider
|
||||
m_Shader: {fileID: 4800000, guid: a6b2542ba8ea844e7b0526fab69d88ed, type: 3}
|
||||
m_ShaderKeywords: GREEN
|
||||
m_LightmapFlags: 4
|
||||
m_EnableInstancingVariants: 0
|
||||
m_DoubleSidedGI: 0
|
||||
m_CustomRenderQueue: -1
|
||||
stringTagMap: {}
|
||||
disabledShaderPasses: []
|
||||
m_SavedProperties:
|
||||
serializedVersion: 3
|
||||
m_TexEnvs:
|
||||
- _BumpMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailAlbedoMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailMask:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailNormalMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _EmissionMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _MainTex:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _MetallicGlossMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _OcclusionMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _ParallaxMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
m_Floats:
|
||||
- _Blue: 0
|
||||
- _BumpScale: 1
|
||||
- _ColorMask: 15
|
||||
- _Cutoff: 0.5
|
||||
- _DetailNormalMapScale: 1
|
||||
- _DstBlend: 0
|
||||
- _GlossMapScale: 1
|
||||
- _Glossiness: 0.5
|
||||
- _GlossyReflections: 1
|
||||
- _GradientStep: 0.2
|
||||
- _Green: 1
|
||||
- _Hue: 0
|
||||
- _Metallic: 0
|
||||
- _Mode: 0
|
||||
- _OcclusionStrength: 1
|
||||
- _OutlineGradient: 0.7
|
||||
- _OutlineOpacity: 0.15
|
||||
- _OutlineWidth: 2.5
|
||||
- _Parallax: 0.02
|
||||
- _Radius: 4
|
||||
- _Red: 0
|
||||
- _SmoothnessTextureChannel: 0
|
||||
- _SpecularHighlights: 1
|
||||
- _SrcBlend: 1
|
||||
- _Stencil: 0
|
||||
- _StencilComp: 8
|
||||
- _StencilOp: 0
|
||||
- _StencilReadMask: 255
|
||||
- _StencilWriteMask: 255
|
||||
- _UVSec: 0
|
||||
- _UseUIAlphaClip: 0
|
||||
- _ZWrite: 1
|
||||
m_Colors:
|
||||
- _Color: {r: 0, g: 0, b: 0, a: 1}
|
||||
- _EmissionColor: {r: 0, g: 0, b: 0, a: 1}
|
93
Assets/UI/HSLSlider.mat
Normal file
93
Assets/UI/HSLSlider.mat
Normal file
@ -0,0 +1,93 @@
|
||||
%YAML 1.1
|
||||
%TAG !u! tag:unity3d.com,2011:
|
||||
--- !u!21 &2100000
|
||||
Material:
|
||||
serializedVersion: 6
|
||||
m_ObjectHideFlags: 0
|
||||
m_CorrespondingSourceObject: {fileID: 0}
|
||||
m_PrefabInstance: {fileID: 0}
|
||||
m_PrefabAsset: {fileID: 0}
|
||||
m_Name: HSLSlider
|
||||
m_Shader: {fileID: 4800000, guid: a6b2542ba8ea844e7b0526fab69d88ed, type: 3}
|
||||
m_ShaderKeywords: HUE
|
||||
m_LightmapFlags: 4
|
||||
m_EnableInstancingVariants: 0
|
||||
m_DoubleSidedGI: 0
|
||||
m_CustomRenderQueue: -1
|
||||
stringTagMap: {}
|
||||
disabledShaderPasses: []
|
||||
m_SavedProperties:
|
||||
serializedVersion: 3
|
||||
m_TexEnvs:
|
||||
- _BumpMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailAlbedoMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailMask:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailNormalMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _EmissionMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _MainTex:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _MetallicGlossMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _OcclusionMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _ParallaxMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
m_Floats:
|
||||
- _Blue: 0
|
||||
- _BumpScale: 1
|
||||
- _ColorMask: 15
|
||||
- _Cutoff: 0.5
|
||||
- _DetailNormalMapScale: 1
|
||||
- _DstBlend: 0
|
||||
- _GlossMapScale: 1
|
||||
- _Glossiness: 0.5
|
||||
- _GlossyReflections: 1
|
||||
- _GradientStep: 0.2
|
||||
- _Green: 0
|
||||
- _Hue: 1
|
||||
- _Metallic: 0
|
||||
- _Mode: 0
|
||||
- _OcclusionStrength: 1
|
||||
- _OutlineGradient: 0.55
|
||||
- _OutlineOpacity: 0.15
|
||||
- _OutlineWidth: 2.5
|
||||
- _Parallax: 0.02
|
||||
- _Radius: 4
|
||||
- _Red: 0
|
||||
- _SmoothnessTextureChannel: 0
|
||||
- _SpecularHighlights: 1
|
||||
- _SrcBlend: 1
|
||||
- _Stencil: 0
|
||||
- _StencilComp: 8
|
||||
- _StencilOp: 0
|
||||
- _StencilReadMask: 255
|
||||
- _StencilWriteMask: 255
|
||||
- _UVSec: 0
|
||||
- _UseUIAlphaClip: 0
|
||||
- _ZWrite: 1
|
||||
m_Colors:
|
||||
- _Color: {r: 0, g: 1, b: 1, a: 1}
|
||||
- _EmissionColor: {r: 0, g: 0, b: 0, a: 1}
|
89
Assets/UI/HSLSquare.mat
Normal file
89
Assets/UI/HSLSquare.mat
Normal file
@ -0,0 +1,89 @@
|
||||
%YAML 1.1
|
||||
%TAG !u! tag:unity3d.com,2011:
|
||||
--- !u!21 &2100000
|
||||
Material:
|
||||
serializedVersion: 6
|
||||
m_ObjectHideFlags: 0
|
||||
m_CorrespondingSourceObject: {fileID: 0}
|
||||
m_PrefabInstance: {fileID: 0}
|
||||
m_PrefabAsset: {fileID: 0}
|
||||
m_Name: HSLSquare
|
||||
m_Shader: {fileID: 4800000, guid: 41b82117f67243a4851d2ce6bbed0d6a, type: 3}
|
||||
m_ShaderKeywords:
|
||||
m_LightmapFlags: 4
|
||||
m_EnableInstancingVariants: 0
|
||||
m_DoubleSidedGI: 0
|
||||
m_CustomRenderQueue: -1
|
||||
stringTagMap: {}
|
||||
disabledShaderPasses: []
|
||||
m_SavedProperties:
|
||||
serializedVersion: 3
|
||||
m_TexEnvs:
|
||||
- _BumpMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailAlbedoMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailMask:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailNormalMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _EmissionMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _MainTex:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _MetallicGlossMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _OcclusionMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _ParallaxMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
m_Floats:
|
||||
- _BumpScale: 1
|
||||
- _ColorMask: 15
|
||||
- _Cutoff: 0.5
|
||||
- _DetailNormalMapScale: 1
|
||||
- _DstBlend: 0
|
||||
- _GlossMapScale: 1
|
||||
- _Glossiness: 0.5
|
||||
- _GlossyReflections: 1
|
||||
- _Hue: 0.566
|
||||
- _Metallic: 0
|
||||
- _Mode: 0
|
||||
- _OcclusionStrength: 1
|
||||
- _OutlineGradient: 0.55
|
||||
- _OutlineOpacity: 0.15
|
||||
- _OutlineWidth: 2.5
|
||||
- _Parallax: 0.02
|
||||
- _Radius: 4
|
||||
- _SmoothnessTextureChannel: 0
|
||||
- _SpecularHighlights: 1
|
||||
- _SrcBlend: 1
|
||||
- _Stencil: 0
|
||||
- _StencilComp: 8
|
||||
- _StencilOp: 0
|
||||
- _StencilReadMask: 255
|
||||
- _StencilWriteMask: 255
|
||||
- _UVSec: 0
|
||||
- _UseUIAlphaClip: 0
|
||||
- _ZWrite: 1
|
||||
m_Colors:
|
||||
- _Color: {r: 1, g: 1, b: 1, a: 1}
|
||||
- _EmissionColor: {r: 0, g: 0, b: 0, a: 1}
|
93
Assets/UI/RSlider.mat
Normal file
93
Assets/UI/RSlider.mat
Normal file
@ -0,0 +1,93 @@
|
||||
%YAML 1.1
|
||||
%TAG !u! tag:unity3d.com,2011:
|
||||
--- !u!21 &2100000
|
||||
Material:
|
||||
serializedVersion: 6
|
||||
m_ObjectHideFlags: 0
|
||||
m_CorrespondingSourceObject: {fileID: 0}
|
||||
m_PrefabInstance: {fileID: 0}
|
||||
m_PrefabAsset: {fileID: 0}
|
||||
m_Name: RSlider
|
||||
m_Shader: {fileID: 4800000, guid: a6b2542ba8ea844e7b0526fab69d88ed, type: 3}
|
||||
m_ShaderKeywords: RED
|
||||
m_LightmapFlags: 4
|
||||
m_EnableInstancingVariants: 0
|
||||
m_DoubleSidedGI: 0
|
||||
m_CustomRenderQueue: -1
|
||||
stringTagMap: {}
|
||||
disabledShaderPasses: []
|
||||
m_SavedProperties:
|
||||
serializedVersion: 3
|
||||
m_TexEnvs:
|
||||
- _BumpMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailAlbedoMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailMask:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailNormalMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _EmissionMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _MainTex:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _MetallicGlossMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _OcclusionMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _ParallaxMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
m_Floats:
|
||||
- _Blue: 0
|
||||
- _BumpScale: 1
|
||||
- _ColorMask: 15
|
||||
- _Cutoff: 0.5
|
||||
- _DetailNormalMapScale: 1
|
||||
- _DstBlend: 0
|
||||
- _GlossMapScale: 1
|
||||
- _Glossiness: 0.5
|
||||
- _GlossyReflections: 1
|
||||
- _GradientStep: 0.2
|
||||
- _Green: 0
|
||||
- _Hue: 0
|
||||
- _Metallic: 0
|
||||
- _Mode: 0
|
||||
- _OcclusionStrength: 1
|
||||
- _OutlineGradient: 0.7
|
||||
- _OutlineOpacity: 0.15
|
||||
- _OutlineWidth: 2.5
|
||||
- _Parallax: 0.02
|
||||
- _Radius: 4
|
||||
- _Red: 1
|
||||
- _SmoothnessTextureChannel: 0
|
||||
- _SpecularHighlights: 1
|
||||
- _SrcBlend: 1
|
||||
- _Stencil: 0
|
||||
- _StencilComp: 8
|
||||
- _StencilOp: 0
|
||||
- _StencilReadMask: 255
|
||||
- _StencilWriteMask: 255
|
||||
- _UVSec: 0
|
||||
- _UseUIAlphaClip: 0
|
||||
- _ZWrite: 1
|
||||
m_Colors:
|
||||
- _Color: {r: 0, g: 0, b: 0, a: 1}
|
||||
- _EmissionColor: {r: 0, g: 0, b: 0, a: 1}
|
92
Assets/UI/Swatch.mat
Normal file
92
Assets/UI/Swatch.mat
Normal file
@ -0,0 +1,92 @@
|
||||
%YAML 1.1
|
||||
%TAG !u! tag:unity3d.com,2011:
|
||||
--- !u!21 &2100000
|
||||
Material:
|
||||
serializedVersion: 6
|
||||
m_ObjectHideFlags: 0
|
||||
m_CorrespondingSourceObject: {fileID: 0}
|
||||
m_PrefabInstance: {fileID: 0}
|
||||
m_PrefabAsset: {fileID: 0}
|
||||
m_Name: Swatch
|
||||
m_Shader: {fileID: 4800000, guid: a6e04e87fe864ed6a3f6a3ce52f57024, type: 3}
|
||||
m_ShaderKeywords: RED
|
||||
m_LightmapFlags: 4
|
||||
m_EnableInstancingVariants: 0
|
||||
m_DoubleSidedGI: 0
|
||||
m_CustomRenderQueue: -1
|
||||
stringTagMap: {}
|
||||
disabledShaderPasses: []
|
||||
m_SavedProperties:
|
||||
serializedVersion: 3
|
||||
m_TexEnvs:
|
||||
- _BumpMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailAlbedoMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailMask:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _DetailNormalMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _EmissionMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _MainTex:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _MetallicGlossMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _OcclusionMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
- _ParallaxMap:
|
||||
m_Texture: {fileID: 0}
|
||||
m_Scale: {x: 1, y: 1}
|
||||
m_Offset: {x: 0, y: 0}
|
||||
m_Floats:
|
||||
- _Blue: 0
|
||||
- _BumpScale: 1
|
||||
- _ColorMask: 15
|
||||
- _Cutoff: 0.5
|
||||
- _DetailNormalMapScale: 1
|
||||
- _DstBlend: 0
|
||||
- _GlossMapScale: 1
|
||||
- _Glossiness: 0.5
|
||||
- _GlossyReflections: 1
|
||||
- _Green: 0
|
||||
- _Hue: 0
|
||||
- _Metallic: 0
|
||||
- _Mode: 0
|
||||
- _OcclusionStrength: 1
|
||||
- _OutlineGradient: 0.3
|
||||
- _OutlineOpacity: 0.15
|
||||
- _OutlineWidth: 2.5
|
||||
- _Parallax: 0.02
|
||||
- _Radius: 4
|
||||
- _Red: 1
|
||||
- _SmoothnessTextureChannel: 0
|
||||
- _SpecularHighlights: 1
|
||||
- _SrcBlend: 1
|
||||
- _Stencil: 0
|
||||
- _StencilComp: 8
|
||||
- _StencilOp: 0
|
||||
- _StencilReadMask: 255
|
||||
- _StencilWriteMask: 255
|
||||
- _UVSec: 0
|
||||
- _UseUIAlphaClip: 0
|
||||
- _ZWrite: 1
|
||||
m_Colors:
|
||||
- _Color: {r: 0.5176471, g: 0.5019608, b: 0.5019608, a: 1}
|
||||
- _EmissionColor: {r: 0, g: 0, b: 0, a: 1}
|
5193
Assets/UI/TextEntryPanel.prefab
Normal file
5193
Assets/UI/TextEntryPanel.prefab
Normal file
File diff suppressed because it is too large
Load Diff
@ -18,6 +18,14 @@ Localization
|
||||
#LOC_ConformalDecals_gui-aspectratio = Aspect Ratio
|
||||
#LOC_ConformalDecals_gui-select-flag = Select Flag
|
||||
#LOC_ConformalDecals_gui-reset-flag = Reset Flag
|
||||
#LOC_ConformalDecals_gui-set-text = Set Text
|
||||
#LOC_ConformalDecals_gui-group-fill = Fill
|
||||
#LOC_ConformalDecals_gui-group-outline = Outline
|
||||
#LOC_ConformalDecals_gui-fill = Fill
|
||||
#LOC_ConformalDecals_gui-set-fill-color = Set Fill Color
|
||||
#LOC_ConformalDecals_gui-outline = Outline
|
||||
#LOC_ConformalDecals_gui-outline-width = Outline Width
|
||||
#LOC_ConformalDecals_gui-set-outline-color = Set Outline Color
|
||||
|
||||
// PARTS
|
||||
|
||||
@ -26,6 +34,11 @@ Localization
|
||||
#LOC_ConformalDecals_flag-description = A simple switchable flag. Can either use the mission flag or select a specific flag to use.
|
||||
#LOC_ConformalDecals_flag-tags = conformal decal sticker flag
|
||||
|
||||
// Text
|
||||
#LOC_ConformalDecals_text-title = CDL-T Text Decal
|
||||
#LOC_ConformalDecals_text-description = A decal that can display custom text in a variety of fonts
|
||||
#LOC_ConformalDecals_text-tags = conformal decal sticker text font
|
||||
|
||||
// Generic Decals
|
||||
#LOC_ConformalDecals_generic-title = CDL-1 Generic Decal
|
||||
#LOC_ConformalDecals_generic-description = A set of generic warning decals and signs to add to your vehicles.
|
||||
@ -64,7 +77,7 @@ Localization
|
||||
|
||||
// Semiotic Decals
|
||||
#LOC_ConformalDecals_semiotic-title = CDL-2 Semiotic Standard Decal
|
||||
#LOC_ConformalDecals_semiotic-description = After several unfortunate mishaps with confusing signage on spacecraft, The Peel-N-Stik™ Corporation teamed up with Kerland-Mutani to unveil the Semiotic Standard for Kerbal Vessels, a set of standardized icons for use on ships and stations.
|
||||
#LOC_ConformalDecals_semiotic-description = After several unfortunate mishaps with confusing signage on spacecraft, The Peel-N-Stik™ Corporation teamed up with Kerland-Mutani to unveil the Semiotic Standard for Kerbal Vessels, a set of standardized icons for use on ships and stations. (Based on the work of Ron Cobb)
|
||||
#LOC_ConformalDecals_semiotic-tags = conformal decal sticker semiotic standard for kerbal vessels Ron Cobb Alien
|
||||
#LOC_ConformalDecals_semiotic-variant-00 = Hazard
|
||||
#LOC_ConformalDecals_semiotic-variant-01 = Blank
|
||||
@ -98,5 +111,47 @@ Localization
|
||||
#LOC_ConformalDecals_semiotic-variant-29 = Radiation Hazard
|
||||
#LOC_ConformalDecals_semiotic-variant-30 = Radiation Bunker
|
||||
#LOC_ConformalDecals_semiotic-variant-31 = Exhaust
|
||||
|
||||
// Munar Decals
|
||||
#LOC_ConformalDecals_munar-title = CDL-3 Surface Base Decal
|
||||
#LOC_ConformalDecals_munar-description = Munar Industries Ltd. saw the wild success of the CDL-2 decal, and wanted to develop a decal set for their own line of Heluim mining bases. These decals are more explicit than the Semiotic Standard and aimed at the hazards that come with more advanced techonologies. (Based on the work of Gavin Rothery)
|
||||
#LOC_ConformalDecals_munar-tag = conformal decal sticker Moon munar lunar industries Gavin Rothery Sarang
|
||||
#LOC_ConformalDecals_munar-variant-severe-danger = Severe Danger
|
||||
#LOC_ConformalDecals_munar-variant-danger = Danger
|
||||
#LOC_ConformalDecals_munar-variant-hazard = Hazard
|
||||
#LOC_ConformalDecals_munar-variant-warning = Warning
|
||||
#LOC_ConformalDecals_munar-variant-bulkhead = Bulkhead
|
||||
#LOC_ConformalDecals_munar-variant-hatch = Hatch
|
||||
#LOC_ConformalDecals_munar-variant-pressure-hatch = Hatch (Pressurized)
|
||||
#LOC_ConformalDecals_munar-variant-door = Doorway
|
||||
#LOC_ConformalDecals_munar-variant-door-danger = Do Not Obstruct
|
||||
#LOC_ConformalDecals_munar-variant-airlock-interior = Airlock (Interior)
|
||||
#LOC_ConformalDecals_munar-variant-airlock-exterior = Airlock (Exterior)
|
||||
#LOC_ConformalDecals_munar-variant-check-seals = Check All Seals
|
||||
#LOC_ConformalDecals_munar-variant-pressure-seal = Pressure Seal
|
||||
#LOC_ConformalDecals_munar-variant-vacuum = Danger Vacuum
|
||||
#LOC_ConformalDecals_munar-variant-gas-mask = Breathing Apparatus Required
|
||||
#LOC_ConformalDecals_munar-variant-oxygen-rich = Oxygen Rich Environment
|
||||
#LOC_ConformalDecals_munar-variant-robotic-work = Heavy Robotic Work Ahead
|
||||
#LOC_ConformalDecals_munar-variant-explosion = Explosion Hazard
|
||||
#LOC_ConformalDecals_munar-variant-radiation = Radiation Hazard
|
||||
#LOC_ConformalDecals_munar-variant-antimatter = Annihilation Hazard
|
||||
#LOC_ConformalDecals_munar-variant-high-voltage = High Voltage
|
||||
#LOC_ConformalDecals_munar-variant-extreme-voltage = Extreme Voltage
|
||||
#LOC_ConformalDecals_munar-variant-explosive-bolts = Explosive Bolts
|
||||
#LOC_ConformalDecals_munar-variant-autonomous-device = Autonomous Device
|
||||
#LOC_ConformalDecals_munar-variant-gravity-adjust = Gravity Adjust
|
||||
#LOC_ConformalDecals_munar-variant-electromagnetic = Strong EM Field
|
||||
#LOC_ConformalDecals_munar-variant-mind-step = Mind Step
|
||||
#LOC_ConformalDecals_munar-variant-class-1 = Class 1 Hazard: Flammable Liquid
|
||||
#LOC_ConformalDecals_munar-variant-class-2 = Class 2 Hazard: Flammable Solid
|
||||
#LOC_ConformalDecals_munar-variant-class-3 = Class 3 Hazard: Magnetic Field
|
||||
#LOC_ConformalDecals_munar-variant-class-4 = Class 4 Hazard: Electric Field
|
||||
#LOC_ConformalDecals_munar-variant-class-5 = Class 5 Hazard: Pressurized Fuel
|
||||
#LOC_ConformalDecals_munar-variant-class-6 = Class 6 Hazard: Pressurized Coolant
|
||||
#LOC_ConformalDecals_munar-variant-class-7 = Class 7 Hazard: Cryogenic Liquid
|
||||
#LOC_ConformalDecals_munar-variant-class-8 = Class 8 Hazard: Radioactive
|
||||
#LOC_ConformalDecals_munar-variant-class-9 = Class 9 Hazard: Ionized Gas
|
||||
#LOC_ConformalDecals_munar-variant-class-10 = Class 10 Hazard: Antimatter
|
||||
}
|
||||
}
|
@ -65,7 +65,12 @@ PART
|
||||
scaleRange = 0.1, 4
|
||||
scaleMode = AVERAGE
|
||||
|
||||
shader = ConformalDecals/Paint/Specular
|
||||
shader = ConformalDecals/Decal/Standard
|
||||
|
||||
KEYWORD {
|
||||
name = DECAL_SDF_ALPHA
|
||||
value = false
|
||||
}
|
||||
|
||||
TEXTURE {
|
||||
name = _Decal
|
||||
@ -108,7 +113,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 128, 2, 128, 112
|
||||
}
|
||||
}
|
||||
@ -123,7 +128,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 256, 2, 128, 112
|
||||
}
|
||||
}
|
||||
@ -138,7 +143,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 384, 2, 128, 112
|
||||
}
|
||||
}
|
||||
@ -153,7 +158,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 690, 4, 330, 118
|
||||
}
|
||||
}
|
||||
@ -168,7 +173,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 898, 4, 122, 118
|
||||
}
|
||||
}
|
||||
@ -183,7 +188,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 6, 126, 196, 132
|
||||
}
|
||||
}
|
||||
@ -198,7 +203,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 210, 122, 94, 94
|
||||
}
|
||||
}
|
||||
@ -213,7 +218,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 8, 262, 192, 70
|
||||
}
|
||||
}
|
||||
@ -242,7 +247,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 508, 332, 262, 112
|
||||
}
|
||||
}
|
||||
@ -257,7 +262,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 202, 218, 164, 114
|
||||
}
|
||||
}
|
||||
@ -272,7 +277,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 366, 218, 164, 114
|
||||
}
|
||||
}
|
||||
@ -347,7 +352,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 326, 426, 164, 20
|
||||
}
|
||||
}
|
||||
@ -363,7 +368,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 776, 388, 48, 48
|
||||
}
|
||||
}
|
||||
@ -378,7 +383,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 328, 456, 48, 48
|
||||
}
|
||||
}
|
||||
@ -393,7 +398,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 376, 456, 48, 48
|
||||
}
|
||||
}
|
||||
@ -408,7 +413,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 424, 456, 48, 48
|
||||
}
|
||||
}
|
||||
@ -423,7 +428,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 472, 456, 48, 48
|
||||
}
|
||||
}
|
||||
@ -438,7 +443,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 520, 456, 48, 48
|
||||
}
|
||||
}
|
||||
@ -453,7 +458,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 568, 456, 48, 48
|
||||
}
|
||||
}
|
||||
@ -468,7 +473,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 614, 456, 48, 48
|
||||
}
|
||||
}
|
||||
@ -483,7 +488,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 662, 456, 48, 48
|
||||
}
|
||||
}
|
||||
@ -498,7 +503,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 710, 456, 48, 48
|
||||
}
|
||||
}
|
||||
@ -513,7 +518,7 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/SpecularSDF
|
||||
KEYWORD { name = DECAL_SDF_ALPHA }
|
||||
tile = 758, 456, 48, 48
|
||||
}
|
||||
}
|
||||
@ -528,7 +533,10 @@ PART
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
shader = ConformalDecals/Paint/Diffuse
|
||||
TEXTURE {
|
||||
name = _SpecMap
|
||||
remove = true
|
||||
}
|
||||
|
||||
tile = 826, 360, 196, 150
|
||||
opacity = 1
|
||||
|
BIN
GameData/ConformalDecals/Parts/Munar/Munar-Atlas.dds
Normal file
BIN
GameData/ConformalDecals/Parts/Munar/Munar-Atlas.dds
Normal file
Binary file not shown.
537
GameData/ConformalDecals/Parts/Munar/decal-munar.cfg
Normal file
537
GameData/ConformalDecals/Parts/Munar/decal-munar.cfg
Normal file
@ -0,0 +1,537 @@
|
||||
PART
|
||||
{
|
||||
name = conformaldecals-munar
|
||||
module = Part
|
||||
author = Andrew Cassidy
|
||||
MODEL
|
||||
{
|
||||
model = ConformalDecals/Assets/decal-blank
|
||||
scale = 1.0, 1.0, 1.0
|
||||
}
|
||||
rescaleFactor = 1
|
||||
|
||||
// Attachment
|
||||
attachRules = 1,1,0,0,1
|
||||
node_attach = 0.0, 0.0, 0.05, 0.0, 0.0, -1.0
|
||||
|
||||
// Tech
|
||||
TechRequired = start
|
||||
|
||||
// Info
|
||||
cost = 75
|
||||
category = Structural
|
||||
|
||||
// CDL-3 Surface Base Decal
|
||||
title = #LOC_ConformalDecals_munar-title
|
||||
|
||||
// Peel-N-Stik Adhesive Decals
|
||||
manufacturer = #LOC_ConformalDecals_agent-peel-n-stick_title
|
||||
|
||||
// Munar Industries Ltd. saw the wild success of the CDL-2 decal, and wanted to develop a decal set for their own line of Heluim mining bases. These decals are more explicit than the Semiotic Standard and aimed at the hazards that come with more advanced techonologies. (Based on the work of Gavin Rothery)
|
||||
description = #LOC_ConformalDecals_munar-description
|
||||
|
||||
// conformal decal sticker Moon munar lunar industries Gavin Rothery Sarang
|
||||
tags = #LOC_ConformalDecals_munar-tags
|
||||
|
||||
bulkheadProfiles = srf
|
||||
|
||||
// Parameters
|
||||
mass = 0.0005
|
||||
dragModel = NONE
|
||||
angularDrag = 0.0
|
||||
crashTolerance = 10
|
||||
maxTemp = 2000
|
||||
breakingForce = 350
|
||||
breakingTorque = 150
|
||||
physicalSignificance = NONE
|
||||
|
||||
MODULE
|
||||
{
|
||||
name = ModuleConformalDecal
|
||||
|
||||
useBaseNormal = true
|
||||
|
||||
tile = -1, -1, 0, 0
|
||||
tileSize = 96, 96
|
||||
tileIndex = 0
|
||||
|
||||
defaultScale = 0.1
|
||||
defaultDepth = 0.1
|
||||
defaultOpacity = 0.8
|
||||
defaultCutoff = 0
|
||||
|
||||
scaleRange = 0.05, 0.5
|
||||
scaleMode = MINIMUM
|
||||
cutoffAdjustable = false
|
||||
|
||||
shader = ConformalDecals/Decal/Standard
|
||||
|
||||
TEXTURE {
|
||||
name = _Decal
|
||||
textureUrl = ConformalDecals/Parts/Munar/Munar-Atlas
|
||||
isMain = true
|
||||
autoTile = true
|
||||
}
|
||||
|
||||
TEXTURE {
|
||||
name = _SpecMap
|
||||
textureUrl = ConformalDecals/Assets/Decal-Spec
|
||||
autoScale = true
|
||||
}
|
||||
}
|
||||
|
||||
MODULE {
|
||||
name = ModuleB9PartSwitch
|
||||
|
||||
SUBTYPE {
|
||||
name = severe-danger
|
||||
title = #LOC_ConformalDecals_munar-variant-severe-danger
|
||||
primaryColor = #2B250D
|
||||
secondaryColor = #F78000
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 0 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = danger
|
||||
title = #LOC_ConformalDecals_munar-variant-danger
|
||||
primaryColor = #93927E
|
||||
secondaryColor = #2B250D
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 2 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = hazard
|
||||
title = #LOC_ConformalDecals_munar-variant-hazard
|
||||
primaryColor = #2B250D
|
||||
secondaryColor = #CC1F01
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 6 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = warning
|
||||
title = #LOC_ConformalDecals_munar-variant-warning
|
||||
primaryColor = #2B250D
|
||||
secondaryColor = #93927E
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 5 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = bulkhead
|
||||
title = #LOC_ConformalDecals_munar-variant-bulkhead
|
||||
primaryColor = #93927E
|
||||
secondaryColor = White
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 7 }
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
SUBTYPE {
|
||||
name = hatch
|
||||
title = #LOC_ConformalDecals_munar-variant-hatch
|
||||
primaryColor = #93927E
|
||||
secondaryColor = White
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 3 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = pressure-hatch
|
||||
title = #LOC_ConformalDecals_munar-variant-pressure-hatch
|
||||
primaryColor = #93927E
|
||||
secondaryColor = White
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 4 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = door
|
||||
title = #LOC_ConformalDecals_munar-variant-door
|
||||
primaryColor = #93927E
|
||||
secondaryColor = White
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 5 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = door-danger
|
||||
title = #LOC_ConformalDecals_munar-variant-door-danger
|
||||
primaryColor = #2B250D
|
||||
secondaryColor = #CC1F01
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
tile = 960, 0, 64, 96
|
||||
scaleMode = HEIGHT
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = airlock-interior
|
||||
title = #LOC_ConformalDecals_munar-variant-airlock-interior
|
||||
primaryColor = #93927E
|
||||
secondaryColor = White
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 866, 96, 142, 96 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = airlock-exterior
|
||||
title = #LOC_ConformalDecals_munar-variant-airlock-exterior
|
||||
primaryColor = #CC1F01
|
||||
secondaryColor = #2B250D
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 768, 96, 142, 96 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = check-seals
|
||||
title = #LOC_ConformalDecals_munar-variant-check-seals
|
||||
primaryColor = White
|
||||
secondaryColor = #93927E
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 8 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = pressure-seal
|
||||
title = #LOC_ConformalDecals_munar-variant-pressure-seal
|
||||
primaryColor = White
|
||||
secondaryColor = #93927E
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 9 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = vacuum
|
||||
title = #LOC_ConformalDecals_munar-variant-vacuum
|
||||
primaryColor = #93927E
|
||||
secondaryColor = #2B250D
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 17 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = gas-mask
|
||||
title = #LOC_ConformalDecals_munar-variant-gas-mask
|
||||
primaryColor = #93927E
|
||||
secondaryColor = White
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 96, 320, 96, 116 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = oxygen-rich
|
||||
title = #LOC_ConformalDecals_munar-variant-oxygen-rich
|
||||
primaryColor = #CC1F01
|
||||
secondaryColor = #2B250D
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 288, 416, 96, 96 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = robotic-work
|
||||
title = #LOC_ConformalDecals_munar-variant-robotic-work
|
||||
primaryColor = #F78000
|
||||
secondaryColor = #2B250D
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 10 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = explosion
|
||||
title = #LOC_ConformalDecals_munar-variant-explosion
|
||||
primaryColor = #F78000
|
||||
secondaryColor = #2B250D
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 0, 320, 96, 116 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = radiation
|
||||
title = #LOC_ConformalDecals_munar-variant-radiation
|
||||
primaryColor = #F78000
|
||||
secondaryColor = #2B250D
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 1 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = antimatter
|
||||
title = #LOC_ConformalDecals_munar-variant-antimatter
|
||||
primaryColor = #F78000
|
||||
secondaryColor = #2B250D
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 11 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = high-voltage
|
||||
title = #LOC_ConformalDecals_munar-variant-high-voltage
|
||||
primaryColor = #93927E
|
||||
secondaryColor = White
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 13 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = extreme-voltage
|
||||
title = #LOC_ConformalDecals_munar-variant-extreme-voltage
|
||||
primaryColor = #2B250D
|
||||
secondaryColor = White
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 14 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = explosive-bolts
|
||||
title = #LOC_ConformalDecals_munar-variant-explosive-bolts
|
||||
primaryColor = #93927E
|
||||
secondaryColor = #CC1F01
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 15 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = autonomous-device
|
||||
title = #LOC_ConformalDecals_munar-variant-autonomous-device
|
||||
primaryColor = #2B250D
|
||||
secondaryColor = White
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tileIndex = 16 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = gravity-adjust
|
||||
title = #LOC_ConformalDecals_munar-variant-gravity-adjust
|
||||
primaryColor = White
|
||||
secondaryColor = #CC1F01
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 288, 320, 96, 96 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = electromagnetic
|
||||
title = #LOC_ConformalDecals_munar-variant-electromagnetic
|
||||
primaryColor = #93927E
|
||||
secondaryColor = White
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA {
|
||||
tile = 967, 195, 50, 90
|
||||
scaleMode = HEIGHT
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = mind-step
|
||||
title = #LOC_ConformalDecals_munar-variant-mind-step
|
||||
primaryColor = #93927E
|
||||
secondaryColor = White
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 1, 447, 230, 64 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = class-1
|
||||
title = #LOC_ConformalDecals_munar-variant-class-1
|
||||
primaryColor = #CC1F01
|
||||
secondaryColor = #93927E
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 0, 192, 96, 128 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = class-2
|
||||
title = #LOC_ConformalDecals_munar-variant-class-2
|
||||
primaryColor = #93927E
|
||||
secondaryColor = #CC1F01
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 96, 192, 96, 128 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = class-3
|
||||
title = #LOC_ConformalDecals_munar-variant-class-3
|
||||
primaryColor = #93927E
|
||||
secondaryColor = #93927E
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 192, 192, 96, 128 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = class-4
|
||||
title = #LOC_ConformalDecals_munar-variant-class-4
|
||||
primaryColor = #93927E
|
||||
secondaryColor = #93927E
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 288, 192, 96, 128 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = class-5
|
||||
title = #LOC_ConformalDecals_munar-variant-class-5
|
||||
primaryColor = #93927E
|
||||
secondaryColor = White
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 384, 192, 96, 128 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = class-6
|
||||
title = #LOC_ConformalDecals_munar-variant-class-6
|
||||
primaryColor = #93927E
|
||||
secondaryColor = White
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 480, 192, 96, 128 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = class-7
|
||||
title = #LOC_ConformalDecals_munar-variant-class-7
|
||||
primaryColor = #F78000
|
||||
secondaryColor = #93927E
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 576, 192, 96, 128 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = class-8
|
||||
title = #LOC_ConformalDecals_munar-variant-class-8
|
||||
primaryColor = #93927E
|
||||
secondaryColor = #F78000
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 672, 192, 96, 128 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = class-9
|
||||
title = #LOC_ConformalDecals_munar-variant-class-9
|
||||
primaryColor = #93927E
|
||||
secondaryColor = #F78000
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 768, 192, 96, 128 }
|
||||
}
|
||||
}
|
||||
|
||||
SUBTYPE {
|
||||
name = class-10
|
||||
title = #LOC_ConformalDecals_munar-variant-class-10
|
||||
primaryColor = #F78000
|
||||
secondaryColor = White
|
||||
|
||||
MODULE {
|
||||
IDENTIFIER { name = ModuleConformalDecal }
|
||||
DATA { tile = 864, 192, 96, 128 }
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
@ -21,13 +21,13 @@ PART
|
||||
cost = 75
|
||||
category = Structural
|
||||
|
||||
// CDL-1 Semiotic Standard Decal
|
||||
// CDL-2 Semiotic Standard Decal
|
||||
title = #LOC_ConformalDecals_semiotic-title
|
||||
|
||||
// Peel-N-Stik Adhesive Decals
|
||||
manufacturer = #LOC_ConformalDecals_agent-peel-n-stick_title
|
||||
|
||||
// After several unfortunate mishaps with confusing signage on spacecraft, The Peel-N-Stik™ Corporation teamed up with Kerland-Mutani to unveil the Semiotic Standard for Kerbal Vessels, a set of standardized icons for use on ships and stations.
|
||||
// After several unfortunate mishaps with confusing signage on spacecraft, The Peel-N-Stik™ Corporation teamed up with Kerland-Mutani to unveil the Semiotic Standard for Kerbal Vessels, a set of standardized icons for use on ships and stations. (Based on the work of Ron Cobb)
|
||||
description = #LOC_ConformalDecals_semiotic-description
|
||||
|
||||
// conformal decal sticker semiotic standard for kerbal vessels Ron Cobb Alien
|
||||
@ -62,7 +62,7 @@ PART
|
||||
scaleRange = 0.05, 0.5
|
||||
cutoffAdjustable = false
|
||||
|
||||
shader = ConformalDecals/Paint/Specular
|
||||
shader = ConformalDecals/Decal/Standard
|
||||
|
||||
TEXTURE {
|
||||
name = _Decal
|
||||
|
@ -53,5 +53,13 @@ PART
|
||||
|
||||
defaultDepth = 0.2
|
||||
defaultCutoff = 0
|
||||
|
||||
shader = ConformalDecals/Decal/Standard
|
||||
|
||||
TEXTURE {
|
||||
name = _SpecMap
|
||||
textureUrl = ConformalDecals/Assets/Decal-Spec
|
||||
autoScale = true
|
||||
}
|
||||
}
|
||||
}
|
||||
|
63
GameData/ConformalDecals/Parts/decal-text.cfg
Normal file
63
GameData/ConformalDecals/Parts/decal-text.cfg
Normal file
@ -0,0 +1,63 @@
|
||||
PART
|
||||
{
|
||||
name = conformaldecals-text
|
||||
module = Part
|
||||
author = Andrew Cassidy
|
||||
MODEL
|
||||
{
|
||||
model = ConformalDecals/Assets/decal-blank
|
||||
scale = 1.0, 1.0, 1.0
|
||||
}
|
||||
rescaleFactor = 1
|
||||
|
||||
// Attachment
|
||||
attachRules = 1,1,0,0,1
|
||||
node_attach = 0.0, 0.0, 0.1, 0.0, 0.0, -1.0
|
||||
|
||||
// Tech
|
||||
TechRequired = start
|
||||
|
||||
// Info
|
||||
cost = 75
|
||||
category = Structural
|
||||
|
||||
// CDL-T Text Decal
|
||||
title = #LOC_ConformalDecals_text-title
|
||||
|
||||
|
||||
// Peel-N-Stik Adhesive Decals
|
||||
manufacturer = #LOC_ConformalDecals_agent-peel-n-stick_title
|
||||
|
||||
// A decal that can display custom text in a variety of fonts
|
||||
description = #LOC_ConformalDecals_text-description
|
||||
|
||||
|
||||
// conformal decal sticker text font
|
||||
tags = #LOC_ConformalDecals_text-tags
|
||||
|
||||
bulkheadProfiles = srf
|
||||
|
||||
// Parameters
|
||||
mass = 0.0005
|
||||
dragModel = NONE
|
||||
angularDrag = 0.0
|
||||
crashTolerance = 10
|
||||
maxTemp = 2000
|
||||
breakingForce = 350
|
||||
breakingTorque = 150
|
||||
physicalSignificance = NONE
|
||||
|
||||
MODULE
|
||||
{
|
||||
name = ModuleConformalText
|
||||
|
||||
text = Text
|
||||
|
||||
shader = ConformalDecals/Decal/Text
|
||||
useBaseNormal = true
|
||||
scaleMode = MINIMUM
|
||||
|
||||
defaultDepth = 0.2
|
||||
defaultCutoff = 0.5
|
||||
}
|
||||
}
|
Binary file not shown.
@ -1,6 +1,7 @@
|
||||
// Custom category, requires WildBlueTools to work
|
||||
MODCAT
|
||||
{
|
||||
name = conformaldecals
|
||||
title = #LOC_ConformalDecals_category-decals_title // Decals
|
||||
|
||||
folderName = ConformalDecals
|
||||
|
@ -20,4 +20,41 @@ CONFORMALDECALS {
|
||||
shader = KSP/Specular (Transparent)
|
||||
shader = Solid Color (Alpha)
|
||||
}
|
||||
|
||||
FONT {
|
||||
name = LiberationSans SDF
|
||||
title = Liberation Sans
|
||||
}
|
||||
|
||||
FONT {
|
||||
name = Calibri SDF
|
||||
title = Calibri
|
||||
}
|
||||
|
||||
FONT {
|
||||
name = NotoSans-Regular SDF
|
||||
title = Noto Sans
|
||||
}
|
||||
|
||||
FONT {
|
||||
name = Waukegan LDO Extended SDF
|
||||
title = Waukegan Extended
|
||||
}
|
||||
|
||||
FONT {
|
||||
name = Nasalization SDF
|
||||
title = Nasalization
|
||||
}
|
||||
|
||||
FONT {
|
||||
name = Helvetica SDF
|
||||
title = Helvetica
|
||||
}
|
||||
|
||||
FONT {
|
||||
name = amarurgt SDF
|
||||
title = Amarillo USAF
|
||||
style = 32
|
||||
styleMask = 4
|
||||
}
|
||||
}
|
@ -0,0 +1,3 @@
|
||||
version https://git-lfs.github.com/spec/v1
|
||||
oid sha256:8580f3c17793051fdc08447401fb7920665ad681ff352588508044b662a3240b
|
||||
size 364900
|
Binary file not shown.
BIN
GameData/ConformalDecals/Resources/ui.conformaldecals
Normal file
BIN
GameData/ConformalDecals/Resources/ui.conformaldecals
Normal file
Binary file not shown.
@ -5,8 +5,8 @@
|
||||
"VERSION":
|
||||
{
|
||||
"MAJOR":0,
|
||||
"MINOR":1,
|
||||
"PATCH":4,
|
||||
"MINOR":2,
|
||||
"PATCH":0,
|
||||
"BUILD":0
|
||||
},
|
||||
"KSP_VERSION":
|
||||
|
@ -1,4 +1,4 @@
|
||||
# Conformal Decals v0.1.4
|
||||
# Conformal Decals v0.2.0
|
||||
[](https://travis-ci.org/drewcassidy/KSP-Conformal-Decals) [](https://creativecommons.org/licenses/by-sa/4.0/) [](https://www.gnu.org/licenses/gpl-3.0)
|
||||
|
||||
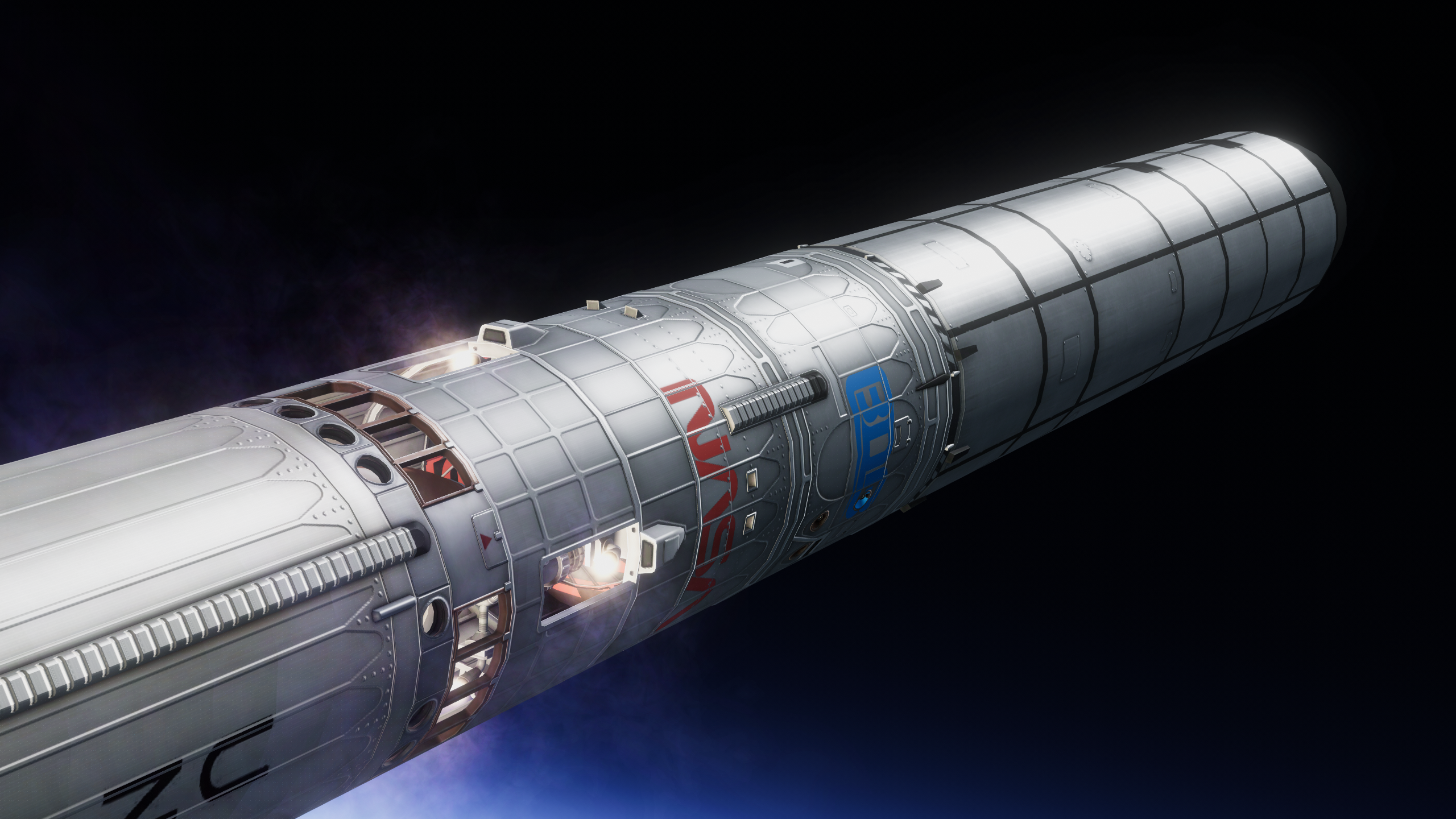
|
||||
@ -20,6 +20,7 @@ Optional:
|
||||
|
||||
- Art and Plugin code: Andrew Cassidy (Cineboxandrew)
|
||||
- Semiotic decal pack based on the work of Ron Cobb
|
||||
- Munar decal pack based on the work of Gavin Rothery
|
||||
|
||||
## Installation
|
||||
|
||||
|
@ -1,6 +1,6 @@
|
||||
<?xml version="1.0" encoding="utf-8"?>
|
||||
<Project ToolsVersion="15.0" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
|
||||
<Import Project="$(MSBuildExtensionsPath)\$(MSBuildToolsVersion)\Microsoft.Common.props" Condition="Exists('$(MSBuildExtensionsPath)\$(MSBuildToolsVersion)\Microsoft.Common.props')" />
|
||||
<Import Project="$(MSBuildExtensionsPath)/$(MSBuildToolsVersion)/Microsoft.Common.props" Condition="Exists('$(MSBuildExtensionsPath)/$(MSBuildToolsVersion)/Microsoft.Common.props')" />
|
||||
<PropertyGroup>
|
||||
<Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
|
||||
<Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform>
|
||||
@ -17,7 +17,7 @@
|
||||
<DebugSymbols>true</DebugSymbols>
|
||||
<DebugType>full</DebugType>
|
||||
<Optimize>false</Optimize>
|
||||
<OutputPath>bin\Debug\</OutputPath>
|
||||
<OutputPath>bin/Debug/</OutputPath>
|
||||
<DefineConstants>DEBUG;TRACE</DefineConstants>
|
||||
<ErrorReport>prompt</ErrorReport>
|
||||
<WarningLevel>4</WarningLevel>
|
||||
@ -25,12 +25,12 @@
|
||||
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Release|AnyCPU' ">
|
||||
<DebugType>pdbonly</DebugType>
|
||||
<Optimize>true</Optimize>
|
||||
<OutputPath>bin\Release\</OutputPath>
|
||||
<OutputPath>bin/Release/</OutputPath>
|
||||
<DefineConstants>TRACE</DefineConstants>
|
||||
<ErrorReport>prompt</ErrorReport>
|
||||
<WarningLevel>4</WarningLevel>
|
||||
<DocumentationFile>bin\Release\ConformalDecals.xml</DocumentationFile>
|
||||
<NoWarn>CS1591</NoWarn>
|
||||
<DocumentationFile>bin/Release/ConformalDecals.xml</DocumentationFile>
|
||||
<NoWarn>CS1591,CS0649</NoWarn>
|
||||
</PropertyGroup>
|
||||
<ItemGroup>
|
||||
<Reference Include="Assembly-CSharp, Version=0.0.0.0, Culture=neutral, PublicKeyToken=null">
|
||||
@ -41,34 +41,72 @@
|
||||
</Reference>
|
||||
<Reference Include="System" />
|
||||
<Reference Include="UnityEngine, Version=0.0.0.0, Culture=neutral, PublicKeyToken=null">
|
||||
<HintPath>dlls\UnityEngine.dll</HintPath>
|
||||
<HintPath>dlls/UnityEngine.dll</HintPath>
|
||||
</Reference>
|
||||
<Reference Include="UnityEngine.AssetBundleModule, Version=0.0.0.0, Culture=neutral, PublicKeyToken=null">
|
||||
<HintPath>dlls/UnityEngine.AssetBundleModule.dll</HintPath>
|
||||
</Reference>
|
||||
<Reference Include="UnityEngine.CoreModule, Version=0.0.0.0, Culture=neutral, PublicKeyToken=null">
|
||||
<HintPath>dlls\UnityEngine.CoreModule.dll</HintPath>
|
||||
<HintPath>dlls/UnityEngine.CoreModule.dll</HintPath>
|
||||
</Reference>
|
||||
<Reference Include="UnityEngine.PhysicsModule, Version=0.0.0.0, Culture=neutral, PublicKeyToken=null">
|
||||
<HintPath>dlls\UnityEngine.PhysicsModule.dll</HintPath>
|
||||
<HintPath>dlls/UnityEngine.PhysicsModule.dll</HintPath>
|
||||
</Reference>
|
||||
<Reference Include="UnityEngine.TextCoreModule, Version=0.0.0.0, Culture=neutral, PublicKeyToken=null">
|
||||
<HintPath>dlls/UnityEngine.TextCoreModule.dll</HintPath>
|
||||
</Reference>
|
||||
<Reference Include="UnityEngine.TextRenderingModule, Version=0.0.0.0, Culture=neutral, PublicKeyToken=null">
|
||||
<HintPath>dlls/UnityEngine.TextRenderingModule.dll</HintPath>
|
||||
</Reference>
|
||||
<Reference Include="UnityEngine.UI, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null">
|
||||
<HintPath>dlls/UnityEngine.UI.dll</HintPath>
|
||||
</Reference>
|
||||
<Reference Include="UnityEngine.UIElementsModule, Version=0.0.0.0, Culture=neutral, PublicKeyToken=null">
|
||||
<HintPath>dlls/UnityEngine.UIElementsModule.dll</HintPath>
|
||||
</Reference>
|
||||
<Reference Include="UnityEngine.UIModule, Version=0.0.0.0, Culture=neutral, PublicKeyToken=null">
|
||||
<HintPath>dlls/UnityEngine.UIModule.dll</HintPath>
|
||||
</Reference>
|
||||
</ItemGroup>
|
||||
<ItemGroup>
|
||||
<Compile Include="DecalConfig.cs" />
|
||||
<Compile Include="DecalIconFixer.cs" />
|
||||
<Compile Include="DecalPropertyIDs.cs" />
|
||||
<Compile Include="MaterialProperties\MaterialColorProperty.cs" />
|
||||
<Compile Include="MaterialProperties\MaterialFloatProperty.cs" />
|
||||
<Compile Include="MaterialProperties\MaterialProperty.cs" />
|
||||
<Compile Include="MaterialProperties\MaterialPropertyCollection.cs" />
|
||||
<Compile Include="MaterialProperties\MaterialTextureProperty.cs" />
|
||||
<Compile Include="MaterialProperties/MaterialColorProperty.cs" />
|
||||
<Compile Include="MaterialProperties/MaterialFloatProperty.cs" />
|
||||
<Compile Include="MaterialProperties/MaterialKeywordProperty.cs" />
|
||||
<Compile Include="MaterialProperties/MaterialProperty.cs" />
|
||||
<Compile Include="MaterialProperties/MaterialPropertyCollection.cs" />
|
||||
<Compile Include="MaterialProperties/MaterialTextureProperty.cs" />
|
||||
<Compile Include="ModuleConformalFlag.cs" />
|
||||
<Compile Include="ModuleConformalText.cs" />
|
||||
<Compile Include="ProjectionTarget.cs" />
|
||||
<Compile Include="ModuleConformalDecal.cs" />
|
||||
<Compile Include="Properties\AssemblyInfo.cs" />
|
||||
<Compile Include="Test\TestLayers.cs" />
|
||||
<Compile Include="Util\Logging.cs" />
|
||||
<Compile Include="Util\OrientedBounds.cs" />
|
||||
<Compile Include="Util\ParseUtil.cs" />
|
||||
<Compile Include="Properties/AssemblyInfo.cs" />
|
||||
<Compile Include="Text/DecalFont.cs" />
|
||||
<Compile Include="Text/FontLoader.cs" />
|
||||
<Compile Include="Text/TextRenderer.cs" />
|
||||
<Compile Include="Text/DecalText.cs" />
|
||||
<Compile Include="Text\DecalTextStyle.cs" />
|
||||
<Compile Include="Text\TextRenderOutput.cs" />
|
||||
<Compile Include="Text\TextRenderJob.cs" />
|
||||
<Compile Include="UI/ColorPickerController.cs" />
|
||||
<Compile Include="UI/FontMenuController.cs" />
|
||||
<Compile Include="UI/FontMenuItem.cs" />
|
||||
<Compile Include="UI/TextEntryController.cs" />
|
||||
<Compile Include="UI/UILoader.cs" />
|
||||
<Compile Include="UI/UITag.cs" />
|
||||
<Compile Include="UI\ColorBoxSlider.cs" />
|
||||
<Compile Include="UI\ColorChannelSlider.cs" />
|
||||
<Compile Include="Util/Logging.cs" />
|
||||
<Compile Include="Util/OrientedBounds.cs" />
|
||||
<Compile Include="Util/ParseUtil.cs" />
|
||||
<Compile Include="UI/BoxSlider.cs" />
|
||||
<Compile Include="Util\ColorHSL.cs" />
|
||||
<Compile Include="Util\ColorHSV.cs" />
|
||||
<Compile Include="Util\ColorUtil.cs" />
|
||||
</ItemGroup>
|
||||
<Import Project="$(MSBuildToolsPath)\Microsoft.CSharp.targets" />
|
||||
<Import Project="$(MSBuildToolsPath)/Microsoft.CSharp.targets" />
|
||||
<PropertyGroup>
|
||||
<PostBuildEvent>sh -e -c "cp -v '$(TargetPath)' '$(SolutionDir)/../GameData/ConformalDecals/Plugins'"</PostBuildEvent>
|
||||
</PropertyGroup>
|
||||
|
@ -1,13 +1,47 @@
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using ConformalDecals.Text;
|
||||
using ConformalDecals.Util;
|
||||
using TMPro;
|
||||
using UniLinq;
|
||||
using UnityEngine;
|
||||
|
||||
namespace ConformalDecals {
|
||||
public static class DecalConfig {
|
||||
private static Texture2D _blankNormal;
|
||||
private static List<string> _shaderBlacklist;
|
||||
private static int _decalLayer = 31;
|
||||
private static bool _selectableInFlight = false;
|
||||
private static Texture2D _blankNormal;
|
||||
private static List<string> _shaderBlacklist;
|
||||
private static Dictionary<string, DecalFont> _fontList;
|
||||
private static int _decalLayer = 31;
|
||||
private static bool _selectableInFlight;
|
||||
|
||||
private struct LegacyShaderEntry {
|
||||
public string name;
|
||||
public string[] keywords;
|
||||
}
|
||||
|
||||
private static readonly Dictionary<string, LegacyShaderEntry> LegacyShaderPairs = new Dictionary<string, LegacyShaderEntry>() {
|
||||
["ConformalDecals/Feature/Bumped"] = new LegacyShaderEntry() {
|
||||
name = "ConformalDecals/Decal/Standard",
|
||||
keywords = new[] {"DECAL_BUMPMAP"}
|
||||
},
|
||||
["ConformalDecals/Paint/Diffuse"] = new LegacyShaderEntry() {
|
||||
name = "ConformalDecals/Decal/Standard",
|
||||
keywords = new string[] { }
|
||||
},
|
||||
["ConformalDecals/Paint/Specular"] = new LegacyShaderEntry() {
|
||||
name = "ConformalDecals/Decal/Standard",
|
||||
keywords = new[] {"DECAL_SPECMAP"}
|
||||
},
|
||||
["ConformalDecals/Paint/DiffuseSDF"] = new LegacyShaderEntry() {
|
||||
name = "ConformalDecals/Decal/Standard",
|
||||
keywords = new[] {"DECAL_SDF_ALPHA"}
|
||||
},
|
||||
["ConformalDecals/Paint/SpecularSDF"] = new LegacyShaderEntry() {
|
||||
name = "ConformalDecals/Decal/Standard",
|
||||
keywords = new[] {"DECAL_SDF_ALPHA", "DECAL_SPECMAP"}
|
||||
},
|
||||
};
|
||||
|
||||
|
||||
public static Texture2D BlankNormal => _blankNormal;
|
||||
|
||||
@ -15,6 +49,10 @@ namespace ConformalDecals {
|
||||
|
||||
public static bool SelectableInFlight => _selectableInFlight;
|
||||
|
||||
public static IEnumerable<DecalFont> Fonts => _fontList.Values;
|
||||
|
||||
public static DecalFont FallbackFont { get; private set; }
|
||||
|
||||
public static bool IsBlacklisted(Shader shader) {
|
||||
return IsBlacklisted(shader.name);
|
||||
}
|
||||
@ -23,19 +61,53 @@ namespace ConformalDecals {
|
||||
return _shaderBlacklist.Contains(shaderName);
|
||||
}
|
||||
|
||||
public static bool IsLegacy(string shaderName, out string newShader, out string[] keywords) {
|
||||
if (LegacyShaderPairs.TryGetValue(shaderName, out var entry)) {
|
||||
newShader = entry.name;
|
||||
keywords = entry.keywords;
|
||||
return true;
|
||||
}
|
||||
|
||||
newShader = null;
|
||||
keywords = null;
|
||||
return false;
|
||||
}
|
||||
|
||||
public static DecalFont GetFont(string name) {
|
||||
if (_fontList.TryGetValue(name, out var font)) {
|
||||
return font;
|
||||
}
|
||||
else {
|
||||
throw new KeyNotFoundException($"Font {name} not found");
|
||||
}
|
||||
}
|
||||
|
||||
private static void ParseConfig(ConfigNode node) {
|
||||
|
||||
ParseUtil.ParseIntIndirect(ref _decalLayer, node, "decalLayer");
|
||||
ParseUtil.ParseBoolIndirect(ref _selectableInFlight, node, "selectableInFlight");
|
||||
|
||||
foreach (var blacklist in node.GetNodes("SHADERBLACKLIST")) {
|
||||
foreach (var shaderName in blacklist.GetValuesList("shader")) {
|
||||
_shaderBlacklist.Add(shaderName);
|
||||
}
|
||||
|
||||
ParseUtil.ParseIntIndirect(ref _decalLayer, node, "decalLayer");
|
||||
ParseUtil.ParseBoolIndirect(ref _selectableInFlight, node, "selectableInFlight");
|
||||
}
|
||||
|
||||
var allFonts = Resources.FindObjectsOfTypeAll<TMP_FontAsset>();
|
||||
|
||||
foreach (var fontNode in node.GetNodes("FONT")) {
|
||||
try {
|
||||
var font = new DecalFont(fontNode, allFonts);
|
||||
_fontList.Add(font.Name, font);
|
||||
}
|
||||
catch (Exception e) {
|
||||
Debug.LogException(e);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
private static Texture2D MakeBlankNormal() {
|
||||
Debug.Log("ConformalDecals: Generating neutral normal map texture");
|
||||
Logging.Log("Generating neutral normal map texture");
|
||||
var width = 2;
|
||||
var height = 2;
|
||||
var color = new Color32(255, 128, 128, 128);
|
||||
@ -56,11 +128,12 @@ namespace ConformalDecals {
|
||||
// ReSharper disable once UnusedMember.Global
|
||||
public static void ModuleManagerPostLoad() {
|
||||
_shaderBlacklist = new List<string>();
|
||||
_fontList = new Dictionary<string, DecalFont>();
|
||||
|
||||
var configs = GameDatabase.Instance.GetConfigs("CONFORMALDECALS");
|
||||
|
||||
if (configs.Length > 0) {
|
||||
Debug.Log("ConformalDecals: loading config");
|
||||
Logging.Log("loading config");
|
||||
foreach (var config in configs) {
|
||||
ParseConfig(config.config);
|
||||
}
|
||||
|
@ -1,4 +1,5 @@
|
||||
using System.Collections.Generic;
|
||||
using ConformalDecals.Util;
|
||||
using UnityEngine;
|
||||
|
||||
namespace ConformalDecals {
|
||||
@ -12,11 +13,11 @@ namespace ConformalDecals {
|
||||
|
||||
public void Start() {
|
||||
foreach (var partName in PartNames) {
|
||||
Debug.Log($"Unf*&king decal preview on {partName}");
|
||||
Logging.Log($"Unf*&king decal preview on '{partName}'");
|
||||
var partInfo = PartLoader.getPartInfoByName(partName);
|
||||
|
||||
if (partInfo == null) {
|
||||
Debug.Log($"Part {partName} not found!");
|
||||
Logging.LogError($"Part {partName} not found!");
|
||||
continue;
|
||||
}
|
||||
|
||||
@ -28,12 +29,12 @@ namespace ConformalDecals {
|
||||
var backTransform = Part.FindHeirarchyTransform(icon.transform, decalModule.decalBack);
|
||||
|
||||
if (frontTransform == null) {
|
||||
Debug.Log($"Part {partName} has no frontTransform");
|
||||
Logging.Log($"Part '{partName}' has no frontTransform");
|
||||
continue;
|
||||
}
|
||||
|
||||
if (backTransform == null) {
|
||||
Debug.Log($"Part {partName} has no backTransform");
|
||||
Logging.Log($"Part '{partName}' has no backTransform");
|
||||
continue;
|
||||
}
|
||||
|
||||
|
@ -1,4 +1,5 @@
|
||||
using UnityEngine;
|
||||
|
||||
// ReSharper disable InconsistentNaming
|
||||
|
||||
namespace ConformalDecals {
|
||||
@ -12,5 +13,6 @@ namespace ConformalDecals {
|
||||
public static readonly int _DecalTangent = Shader.PropertyToID("_DecalTangent");
|
||||
public static readonly int _EdgeWearStrength = Shader.PropertyToID("_EdgeWearStrength");
|
||||
public static readonly int _ProjectionMatrix = Shader.PropertyToID("_ProjectionMatrix");
|
||||
public static readonly int _ZWrite = Shader.PropertyToID("_ZWrite");
|
||||
}
|
||||
}
|
@ -0,0 +1,19 @@
|
||||
using ConformalDecals.Util;
|
||||
using UnityEngine;
|
||||
|
||||
namespace ConformalDecals.MaterialProperties {
|
||||
public class MaterialKeywordProperty : MaterialProperty {
|
||||
[SerializeField] public bool value = true;
|
||||
|
||||
public override void ParseNode(ConfigNode node) {
|
||||
base.ParseNode(node);
|
||||
|
||||
ParseUtil.ParseBoolIndirect(ref value, node, "value");
|
||||
}
|
||||
|
||||
public override void Modify(Material material) {
|
||||
if (value) material.EnableKeyword(_propertyName);
|
||||
else material.DisableKeyword(_propertyName);
|
||||
}
|
||||
}
|
||||
}
|
@ -14,13 +14,14 @@ namespace ConformalDecals.MaterialProperties {
|
||||
[SerializeField] protected int _propertyID;
|
||||
[SerializeField] protected string _propertyName;
|
||||
|
||||
public abstract void Modify(Material material);
|
||||
|
||||
public virtual void ParseNode(ConfigNode node) {
|
||||
if (node == null) throw new ArgumentNullException(nameof(node));
|
||||
|
||||
PropertyName = node.GetValue("name");
|
||||
Debug.Log($"Parsing material property {_propertyName}");
|
||||
}
|
||||
|
||||
public abstract void Modify(Material material);
|
||||
public virtual void Remove(Material material) { }
|
||||
}
|
||||
}
|
@ -30,12 +30,20 @@ namespace ConformalDecals.MaterialProperties {
|
||||
|
||||
public Shader DecalShader => _shader;
|
||||
|
||||
public IEnumerable<Material> Materials {
|
||||
get {
|
||||
yield return PreviewMaterial;
|
||||
yield return DecalMaterial;
|
||||
}
|
||||
}
|
||||
|
||||
public Material DecalMaterial {
|
||||
get {
|
||||
if (_decalMaterial == null) {
|
||||
_decalMaterial = new Material(_shader);
|
||||
|
||||
_decalMaterial.SetInt(DecalPropertyIDs._Cull, (int) CullMode.Off);
|
||||
_decalMaterial.SetInt(DecalPropertyIDs._ZWrite, 0);
|
||||
_decalMaterial.renderQueue = RenderQueue;
|
||||
}
|
||||
|
||||
@ -50,6 +58,7 @@ namespace ConformalDecals.MaterialProperties {
|
||||
|
||||
_previewMaterial.EnableKeyword("DECAL_PREVIEW");
|
||||
_previewMaterial.SetInt(DecalPropertyIDs._Cull, (int) CullMode.Back);
|
||||
_previewMaterial.SetInt(DecalPropertyIDs._ZWrite, 1);
|
||||
}
|
||||
|
||||
return _previewMaterial;
|
||||
@ -69,7 +78,6 @@ namespace ConformalDecals.MaterialProperties {
|
||||
public float AspectRatio => MainTexture == null ? 1 : MainTexture.AspectRatio;
|
||||
|
||||
public void OnBeforeSerialize() {
|
||||
Debug.Log($"Serializing MaterialPropertyCollection {this.GetInstanceID()}");
|
||||
if (_materialProperties == null) throw new SerializationException("Tried to serialize an uninitialized MaterialPropertyCollection");
|
||||
|
||||
_serializedNames = _materialProperties.Keys.ToArray();
|
||||
@ -77,7 +85,6 @@ namespace ConformalDecals.MaterialProperties {
|
||||
}
|
||||
|
||||
public void OnAfterDeserialize() {
|
||||
Debug.Log($"Deserializing MaterialPropertyCollection {this.GetInstanceID()}");
|
||||
if (_serializedNames == null) throw new SerializationException("ID array is null");
|
||||
if (_serializedProperties == null) throw new SerializationException("Property array is null");
|
||||
if (_serializedProperties.Length != _serializedNames.Length) throw new SerializationException("Material property arrays are different lengths.");
|
||||
@ -95,7 +102,6 @@ namespace ConformalDecals.MaterialProperties {
|
||||
}
|
||||
|
||||
public void Awake() {
|
||||
Debug.Log($"MaterialPropertyCollection {this.GetInstanceID()} onAwake");
|
||||
_materialProperties ??= new Dictionary<string, MaterialProperty>();
|
||||
}
|
||||
|
||||
@ -145,6 +151,20 @@ namespace ConformalDecals.MaterialProperties {
|
||||
}
|
||||
}
|
||||
|
||||
public bool RemoveProperty(string propertyName) {
|
||||
if (_materialProperties.TryGetValue(propertyName, out var property)) {
|
||||
foreach (var material in Materials) {
|
||||
property.Remove(material);
|
||||
}
|
||||
_materialProperties.Remove(propertyName);
|
||||
Destroy(property);
|
||||
|
||||
return true;
|
||||
}
|
||||
|
||||
return false;
|
||||
}
|
||||
|
||||
public MaterialTextureProperty AddTextureProperty(string propertyName, bool isMain = false) {
|
||||
var newProperty = AddProperty<MaterialTextureProperty>(propertyName);
|
||||
if (isMain) _mainTexture = newProperty;
|
||||
@ -167,6 +187,8 @@ namespace ConformalDecals.MaterialProperties {
|
||||
string propertyName = "";
|
||||
if (!ParseUtil.ParseStringIndirect(ref propertyName, node, "name")) throw new ArgumentException("node has no name");
|
||||
|
||||
if (ParseUtil.ParseBool(node, "remove", true)) RemoveProperty(propertyName);
|
||||
|
||||
var newProperty = AddOrGetProperty<T>(propertyName);
|
||||
newProperty.ParseNode(node);
|
||||
|
||||
@ -180,14 +202,23 @@ namespace ConformalDecals.MaterialProperties {
|
||||
public void SetShader(string shaderName) {
|
||||
if (string.IsNullOrEmpty(shaderName)) {
|
||||
if (_shader == null) {
|
||||
Debug.Log("Using default decal shader");
|
||||
shaderName = "ConformalDecals/Paint/Diffuse";
|
||||
Logging.Log("Using default decal shader");
|
||||
shaderName = "ConformalDecals/Decal/Standard";
|
||||
}
|
||||
else {
|
||||
return;
|
||||
}
|
||||
}
|
||||
|
||||
if (DecalConfig.IsLegacy(shaderName, out var newShader, out var keywords)) {
|
||||
Logging.LogWarning($"Part is using shader {shaderName}, which has been replaced by {newShader}.");
|
||||
shaderName = newShader;
|
||||
foreach (var keyword in keywords) {
|
||||
var newProperty = AddOrGetProperty<MaterialKeywordProperty>(keyword);
|
||||
newProperty.value = true;
|
||||
}
|
||||
}
|
||||
|
||||
var shader = Shabby.Shabby.FindShader(shaderName);
|
||||
|
||||
if (shader == null) throw new FormatException($"Unable to find specified shader '{shaderName}'");
|
||||
@ -209,8 +240,6 @@ namespace ConformalDecals.MaterialProperties {
|
||||
if (_mainTexture == null) throw new InvalidOperationException("UpdateTile called but no main texture is specified!");
|
||||
var mainTexSize = _mainTexture.Dimensions;
|
||||
|
||||
Debug.Log($"Main texture is {_mainTexture.PropertyName} and its size is {mainTexSize}");
|
||||
|
||||
foreach (var entry in _materialProperties) {
|
||||
if (entry.Value is MaterialTextureProperty textureProperty && textureProperty.autoTile) {
|
||||
textureProperty.SetTile(tile, mainTexSize);
|
||||
@ -244,8 +273,9 @@ namespace ConformalDecals.MaterialProperties {
|
||||
}
|
||||
|
||||
public void UpdateMaterials() {
|
||||
UpdateMaterial(DecalMaterial);
|
||||
UpdateMaterial(PreviewMaterial);
|
||||
foreach (var material in Materials) {
|
||||
UpdateMaterial(material);
|
||||
}
|
||||
}
|
||||
|
||||
public void UpdateMaterial(Material material) {
|
||||
|
@ -10,7 +10,7 @@ namespace ConformalDecals.MaterialProperties {
|
||||
[SerializeField] public bool autoTile;
|
||||
|
||||
[SerializeField] private string _textureUrl;
|
||||
[SerializeField] private Texture2D _texture = Texture2D.whiteTexture;
|
||||
[SerializeField] private Texture2D _texture;
|
||||
|
||||
[SerializeField] private bool _hasTile;
|
||||
[SerializeField] private Rect _tileRect;
|
||||
@ -19,7 +19,10 @@ namespace ConformalDecals.MaterialProperties {
|
||||
[SerializeField] private Vector2 _textureOffset;
|
||||
[SerializeField] private Vector2 _textureScale = Vector2.one;
|
||||
|
||||
public Texture2D Texture => _texture;
|
||||
public Texture2D Texture {
|
||||
get => _texture;
|
||||
set => _texture = value;
|
||||
}
|
||||
|
||||
public string TextureUrl {
|
||||
get => _textureUrl;
|
||||
@ -55,18 +58,29 @@ namespace ConformalDecals.MaterialProperties {
|
||||
if (ParseUtil.ParseStringIndirect(ref _textureUrl, node, "textureUrl")) {
|
||||
_texture = LoadTexture(_textureUrl, isNormal);
|
||||
}
|
||||
|
||||
if (_texture == null) {
|
||||
_texture = isNormal ? DecalConfig.BlankNormal : Texture2D.whiteTexture;
|
||||
}
|
||||
}
|
||||
|
||||
public override void Modify(Material material) {
|
||||
if (material == null) throw new ArgumentNullException(nameof(material));
|
||||
if (_texture == null) {
|
||||
_texture = Texture2D.whiteTexture;
|
||||
throw new NullReferenceException("texture is null, but should not be");
|
||||
_texture = isNormal ? DecalConfig.BlankNormal : Texture2D.whiteTexture;
|
||||
}
|
||||
|
||||
material.SetTexture(_propertyID, _texture);
|
||||
material.SetTextureOffset(_propertyID, _textureOffset);
|
||||
material.SetTextureScale(_propertyID, _textureScale * _scale);
|
||||
if (_propertyName != "_Decal") material.EnableKeyword("DECAL" + _propertyName.ToUpper());
|
||||
}
|
||||
|
||||
public override void Remove(Material material) {
|
||||
if (material == null) throw new ArgumentNullException(nameof(material));
|
||||
base.Remove(material);
|
||||
|
||||
if (_propertyName != "_Decal") material.DisableKeyword("DECAL" + _propertyName.ToUpper());
|
||||
}
|
||||
|
||||
public void SetScale(Vector2 scale) {
|
||||
@ -94,7 +108,7 @@ namespace ConformalDecals.MaterialProperties {
|
||||
}
|
||||
|
||||
private static Texture2D LoadTexture(string textureUrl, bool isNormal) {
|
||||
Debug.Log($"loading texture '{textureUrl}', isNormalMap = {isNormal}");
|
||||
//Logging.Log($"loading texture '{textureUrl}', isNormalMap = {isNormal}");
|
||||
if ((string.IsNullOrEmpty(textureUrl) && isNormal) || textureUrl == "Bump") {
|
||||
return Texture2D.normalTexture;
|
||||
}
|
||||
|
@ -19,7 +19,7 @@ namespace ConformalDecals {
|
||||
|
||||
// CONFIGURABLE VALUES
|
||||
|
||||
[KSPField] public string shader = "ConformalDecals/Paint/Diffuse";
|
||||
[KSPField] public string shader = "ConformalDecals/Decal/Standard";
|
||||
|
||||
[KSPField] public string decalFront = "Decal-Front";
|
||||
[KSPField] public string decalBack = "Decal-Back";
|
||||
@ -130,7 +130,6 @@ namespace ConformalDecals {
|
||||
|
||||
/// <inheritdoc />
|
||||
public override void OnLoad(ConfigNode node) {
|
||||
this.Log("Loading module");
|
||||
try {
|
||||
// SETUP TRANSFORMS
|
||||
decalFrontTransform = part.FindModelTransform(decalFront);
|
||||
@ -150,7 +149,6 @@ namespace ConformalDecals {
|
||||
|
||||
// SETUP BACK MATERIAL
|
||||
if (updateBackScale) {
|
||||
this.Log("Getting material and base scale for back material");
|
||||
var backRenderer = decalBackTransform.GetComponent<MeshRenderer>();
|
||||
if (backRenderer == null) {
|
||||
this.LogError($"Specified decalBack transform {decalBack} has no renderer attached! Setting updateBackScale to false.");
|
||||
@ -172,6 +170,12 @@ namespace ConformalDecals {
|
||||
|
||||
// set shader
|
||||
materialProperties.SetShader(shader);
|
||||
materialProperties.AddOrGetProperty<MaterialKeywordProperty>("DECAL_BASE_NORMAL").value = useBaseNormal;
|
||||
|
||||
// add keyword nodes
|
||||
foreach (var keywordNode in node.GetNodes("KEYWORD")) {
|
||||
materialProperties.ParseProperty<MaterialKeywordProperty>(keywordNode);
|
||||
}
|
||||
|
||||
// add texture nodes
|
||||
foreach (var textureNode in node.GetNodes("TEXTURE")) {
|
||||
@ -190,7 +194,6 @@ namespace ConformalDecals {
|
||||
|
||||
// handle texture tiling parameters
|
||||
var tileString = node.GetValue("tile");
|
||||
this.Log(tileString);
|
||||
if (!string.IsNullOrEmpty(tileString)) {
|
||||
var tileValid = ParseExtensions.TryParseRect(tileString, out tileRect);
|
||||
if (!tileValid) throw new FormatException($"Invalid rect value for tile '{tileString}'");
|
||||
@ -202,9 +205,6 @@ namespace ConformalDecals {
|
||||
else if (tileIndex >= 0) {
|
||||
materialProperties.UpdateTile(tileIndex, tileSize);
|
||||
}
|
||||
|
||||
// QUEUE PART FOR ICON FIXING IN VAB
|
||||
DecalIconFixer.QueuePart(part.name);
|
||||
}
|
||||
catch (Exception e) {
|
||||
this.LogException("Exception parsing partmodule", e);
|
||||
@ -212,6 +212,10 @@ namespace ConformalDecals {
|
||||
|
||||
UpdateMaterials();
|
||||
|
||||
foreach (var keyword in _decalMaterial.shaderKeywords) {
|
||||
this.Log($"keyword: {keyword}");
|
||||
}
|
||||
|
||||
if (HighLogic.LoadedSceneIsEditor) {
|
||||
UpdateTweakables();
|
||||
}
|
||||
@ -225,6 +229,9 @@ namespace ConformalDecals {
|
||||
opacity = defaultOpacity;
|
||||
cutoff = defaultCutoff;
|
||||
wear = defaultWear;
|
||||
|
||||
// QUEUE PART FOR ICON FIXING IN VAB
|
||||
DecalIconFixer.QueuePart(part.name);
|
||||
}
|
||||
}
|
||||
|
||||
@ -235,14 +242,10 @@ namespace ConformalDecals {
|
||||
|
||||
/// <inheritdoc />
|
||||
public override void OnStart(StartState state) {
|
||||
this.Log("Starting module");
|
||||
|
||||
materialProperties.RenderQueue = DecalQueue;
|
||||
|
||||
_boundsRenderer = decalProjectorTransform.GetComponent<MeshRenderer>();
|
||||
|
||||
UpdateMaterials();
|
||||
|
||||
// handle tweakables
|
||||
if (HighLogic.LoadedSceneIsEditor) {
|
||||
GameEvents.onEditorPartEvent.Add(OnEditorEvent);
|
||||
@ -263,13 +266,13 @@ namespace ConformalDecals {
|
||||
OnAttach();
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
// handle flight events
|
||||
if (HighLogic.LoadedSceneIsFlight) {
|
||||
GameEvents.onPartWillDie.Add(OnPartWillDie);
|
||||
|
||||
|
||||
if (part.parent == null) part.explode();
|
||||
|
||||
|
||||
Part.layerMask |= 1 << DecalConfig.DecalLayer;
|
||||
decalColliderTransform.gameObject.layer = DecalConfig.DecalLayer;
|
||||
|
||||
@ -354,8 +357,8 @@ namespace ConformalDecals {
|
||||
part.Die();
|
||||
}
|
||||
}
|
||||
|
||||
protected void OnAttach() {
|
||||
|
||||
protected virtual void OnAttach() {
|
||||
if (part.parent == null) {
|
||||
this.LogError("Attach function called but part has no parent!");
|
||||
_isAttached = false;
|
||||
@ -364,8 +367,6 @@ namespace ConformalDecals {
|
||||
|
||||
_isAttached = true;
|
||||
|
||||
this.Log($"Decal attached to {part.parent.partName}");
|
||||
|
||||
// hide model
|
||||
decalModelTransform.gameObject.SetActive(false);
|
||||
|
||||
@ -380,7 +381,7 @@ namespace ConformalDecals {
|
||||
UpdateScale();
|
||||
}
|
||||
|
||||
protected void OnDetach() {
|
||||
protected virtual void OnDetach() {
|
||||
_isAttached = false;
|
||||
|
||||
// unhide model
|
||||
@ -405,10 +406,10 @@ namespace ConformalDecals {
|
||||
switch (scaleMode) {
|
||||
default:
|
||||
case DecalScaleMode.HEIGHT:
|
||||
size = new Vector2(scale, scale * aspectRatio);
|
||||
size = new Vector2(scale / aspectRatio, scale);
|
||||
break;
|
||||
case DecalScaleMode.WIDTH:
|
||||
size = new Vector2(scale / aspectRatio, scale);
|
||||
size = new Vector2(scale, scale * aspectRatio);
|
||||
break;
|
||||
case DecalScaleMode.AVERAGE:
|
||||
var width1 = 2 * scale / (1 + aspectRatio);
|
||||
@ -453,7 +454,7 @@ namespace ConformalDecals {
|
||||
}
|
||||
}
|
||||
|
||||
protected void UpdateMaterials() {
|
||||
protected virtual void UpdateMaterials() {
|
||||
materialProperties.UpdateMaterials();
|
||||
materialProperties.SetOpacity(opacity);
|
||||
materialProperties.SetCutoff(cutoff);
|
||||
@ -497,7 +498,7 @@ namespace ConformalDecals {
|
||||
}
|
||||
}
|
||||
|
||||
protected void UpdateTweakables() {
|
||||
protected virtual void UpdateTweakables() {
|
||||
// setup tweakable fields
|
||||
var scaleField = Fields[nameof(scale)];
|
||||
var depthField = Fields[nameof(depth)];
|
||||
@ -511,7 +512,7 @@ namespace ConformalDecals {
|
||||
cutoffField.guiActiveEditor = cutoffAdjustable;
|
||||
wearField.guiActiveEditor = useBaseNormal;
|
||||
|
||||
var steps = 20;
|
||||
var steps = 40;
|
||||
|
||||
if (scaleAdjustable) {
|
||||
var minValue = Mathf.Max(Mathf.Epsilon, scaleRange.x);
|
||||
|
307
Source/ConformalDecals/ModuleConformalText.cs
Normal file
307
Source/ConformalDecals/ModuleConformalText.cs
Normal file
@ -0,0 +1,307 @@
|
||||
using ConformalDecals.MaterialProperties;
|
||||
using ConformalDecals.Text;
|
||||
using ConformalDecals.UI;
|
||||
using ConformalDecals.Util;
|
||||
using TMPro;
|
||||
using UnityEngine;
|
||||
|
||||
namespace ConformalDecals {
|
||||
public class ModuleConformalText : ModuleConformalDecal, ISerializationCallbackReceiver {
|
||||
[KSPField(isPersistant = true)] public string text = "Text";
|
||||
|
||||
[KSPField] public Vector2 lineSpacingRange = new Vector2(-50, 50);
|
||||
[KSPField] public Vector2 charSpacingRange = new Vector2(-50, 50);
|
||||
|
||||
// serialization-only fields. do not use except in serialization functions
|
||||
[KSPField(isPersistant = true)] public string fontName = "Calibri SDF";
|
||||
[KSPField(isPersistant = true)] public int style;
|
||||
[KSPField(isPersistant = true)] public bool vertical;
|
||||
[KSPField(isPersistant = true)] public float lineSpacing;
|
||||
[KSPField(isPersistant = true)] public float charSpacing;
|
||||
[KSPField(isPersistant = true)] public string fillColor = "000000FF";
|
||||
[KSPField(isPersistant = true)] public string outlineColor = "FFFFFFFF";
|
||||
|
||||
// KSP TWEAKABLES
|
||||
|
||||
[KSPEvent(guiName = "#LOC_ConformalDecals_gui-set-text", guiActive = false, guiActiveEditor = true)]
|
||||
public void SetText() {
|
||||
if (_textEntryController == null) {
|
||||
_textEntryController = TextEntryController.Create(text, _font, _style, lineSpacingRange, charSpacingRange, OnTextUpdate);
|
||||
}
|
||||
else {
|
||||
_textEntryController.Close();
|
||||
}
|
||||
}
|
||||
|
||||
// FILL
|
||||
|
||||
[KSPField(guiName = "#LOC_ConformalDecals_gui-fill", groupName = "decal-fill", groupDisplayName = "#LOC_ConformalDecals_gui-group-fill",
|
||||
guiActive = false, guiActiveEditor = true, isPersistant = true),
|
||||
UI_Toggle()]
|
||||
public bool fillEnabled = true;
|
||||
|
||||
[KSPEvent(guiName = "#LOC_ConformalDecals_gui-set-fill-color", groupName = "decal-fill", groupDisplayName = "#LOC_ConformalDecals_gui-group-fill",
|
||||
guiActive = false, guiActiveEditor = true)]
|
||||
public void SetFillColor() {
|
||||
if (_fillColorPickerController == null) {
|
||||
_fillColorPickerController = ColorPickerController.Create(_fillColor, OnFillColorUpdate);
|
||||
}
|
||||
else {
|
||||
_fillColorPickerController.Close();
|
||||
}
|
||||
}
|
||||
|
||||
// OUTLINE
|
||||
|
||||
[KSPField(guiName = "#LOC_ConformalDecals_gui-outline", groupName = "decal-outline", groupDisplayName = "#LOC_ConformalDecals_gui-group-outline",
|
||||
guiActive = false, guiActiveEditor = true, isPersistant = true),
|
||||
UI_Toggle()]
|
||||
public bool outlineEnabled;
|
||||
|
||||
[KSPField(guiName = "#LOC_ConformalDecals_gui-outline-width", groupName = "decal-outline", groupDisplayName = "#LOC_ConformalDecals_gui-group-outline",
|
||||
guiActive = false, guiActiveEditor = true, isPersistant = true, guiFormat = "F2"),
|
||||
UI_FloatRange(stepIncrement = 0.05f)]
|
||||
public float outlineWidth = 0.1f;
|
||||
|
||||
[KSPEvent(guiName = "#LOC_ConformalDecals_gui-set-outline-color", groupName = "decal-outline", groupDisplayName = "#LOC_ConformalDecals_gui-group-outline",
|
||||
guiActive = false, guiActiveEditor = true)]
|
||||
public void SetOutlineColor() {
|
||||
if (_outlineColorPickerController == null) {
|
||||
_outlineColorPickerController = ColorPickerController.Create(_outlineColor, OnOutlineColorUpdate);
|
||||
}
|
||||
else {
|
||||
_outlineColorPickerController.Close();
|
||||
}
|
||||
}
|
||||
|
||||
private DecalTextStyle _style;
|
||||
private DecalFont _font;
|
||||
private Color32 _fillColor;
|
||||
private Color32 _outlineColor;
|
||||
|
||||
private TextEntryController _textEntryController;
|
||||
private ColorPickerController _fillColorPickerController;
|
||||
private ColorPickerController _outlineColorPickerController;
|
||||
|
||||
private MaterialTextureProperty _decalTextureProperty;
|
||||
|
||||
private MaterialKeywordProperty _fillEnabledProperty;
|
||||
private MaterialColorProperty _fillColorProperty;
|
||||
|
||||
private MaterialKeywordProperty _outlineEnabledProperty;
|
||||
private MaterialColorProperty _outlineColorProperty;
|
||||
private MaterialFloatProperty _outlineWidthProperty;
|
||||
|
||||
private TextRenderJob _currentJob;
|
||||
private DecalText _currentText;
|
||||
|
||||
public override void OnLoad(ConfigNode node) {
|
||||
base.OnLoad(node);
|
||||
OnAfterDeserialize();
|
||||
|
||||
UpdateTextRecursive();
|
||||
}
|
||||
|
||||
public override void OnSave(ConfigNode node) {
|
||||
OnBeforeSerialize();
|
||||
base.OnSave(node);
|
||||
}
|
||||
|
||||
public override void OnStart(StartState state) {
|
||||
base.OnStart(state);
|
||||
|
||||
UpdateTextRecursive();
|
||||
}
|
||||
|
||||
public override void OnAwake() {
|
||||
base.OnAwake();
|
||||
|
||||
_decalTextureProperty = materialProperties.AddOrGetTextureProperty("_Decal", true);
|
||||
|
||||
_fillEnabledProperty = materialProperties.AddOrGetProperty<MaterialKeywordProperty>("DECAL_FILL");
|
||||
_fillColorProperty = materialProperties.AddOrGetProperty<MaterialColorProperty>("_DecalColor");
|
||||
|
||||
_outlineEnabledProperty = materialProperties.AddOrGetProperty<MaterialKeywordProperty>("DECAL_OUTLINE");
|
||||
_outlineColorProperty = materialProperties.AddOrGetProperty<MaterialColorProperty>("_OutlineColor");
|
||||
_outlineWidthProperty = materialProperties.AddOrGetProperty<MaterialFloatProperty>("_OutlineWidth");
|
||||
}
|
||||
|
||||
public void OnTextUpdate(string newText, DecalFont newFont, DecalTextStyle newStyle) {
|
||||
text = newText;
|
||||
_font = newFont;
|
||||
_style = newStyle;
|
||||
UpdateTextRecursive();
|
||||
}
|
||||
|
||||
public void OnFillColorUpdate(Color rgb, Util.ColorHSV hsv) {
|
||||
_fillColor = rgb;
|
||||
UpdateMaterials();
|
||||
|
||||
foreach (var counterpart in part.symmetryCounterparts) {
|
||||
var decal = counterpart.GetComponent<ModuleConformalText>();
|
||||
decal._fillColor = _fillColor;
|
||||
decal.UpdateMaterials();
|
||||
}
|
||||
}
|
||||
|
||||
public void OnOutlineColorUpdate(Color rgb, Util.ColorHSV hsv) {
|
||||
_outlineColor = rgb;
|
||||
UpdateMaterials();
|
||||
|
||||
foreach (var counterpart in part.symmetryCounterparts) {
|
||||
var decal = counterpart.GetComponent<ModuleConformalText>();
|
||||
decal._outlineColor = _outlineColor;
|
||||
decal.UpdateMaterials();
|
||||
}
|
||||
}
|
||||
|
||||
public void OnFillToggle(BaseField field, object obj) {
|
||||
// fill and outline cant both be disabled
|
||||
outlineEnabled = outlineEnabled || (!outlineEnabled && !fillEnabled);
|
||||
|
||||
UpdateTweakables();
|
||||
UpdateMaterials();
|
||||
|
||||
foreach (var counterpart in part.symmetryCounterparts) {
|
||||
var decal = counterpart.GetComponent<ModuleConformalText>();
|
||||
decal.fillEnabled = fillEnabled;
|
||||
decal.outlineEnabled = outlineEnabled;
|
||||
decal.UpdateTweakables();
|
||||
decal.UpdateMaterials();
|
||||
}
|
||||
}
|
||||
|
||||
public void OnOutlineToggle(BaseField field, object obj) {
|
||||
// fill and outline cant both be disabled
|
||||
fillEnabled = fillEnabled || (!fillEnabled && !outlineEnabled);
|
||||
|
||||
UpdateTweakables();
|
||||
UpdateMaterials();
|
||||
|
||||
foreach (var counterpart in part.symmetryCounterparts) {
|
||||
var decal = counterpart.GetComponent<ModuleConformalText>();
|
||||
decal.fillEnabled = fillEnabled;
|
||||
decal.outlineEnabled = outlineEnabled;
|
||||
decal.UpdateTweakables();
|
||||
decal.UpdateMaterials();
|
||||
}
|
||||
}
|
||||
|
||||
public void OnOutlineWidthUpdate(BaseField field, object obj) {
|
||||
UpdateMaterials();
|
||||
|
||||
foreach (var counterpart in part.symmetryCounterparts) {
|
||||
var decal = counterpart.GetComponent<ModuleConformalText>();
|
||||
decal.outlineWidth = outlineWidth;
|
||||
decal.UpdateMaterials();
|
||||
}
|
||||
}
|
||||
|
||||
public void OnBeforeSerialize() {
|
||||
fontName = _font.Name;
|
||||
style = (int) _style.FontStyle;
|
||||
vertical = _style.Vertical;
|
||||
lineSpacing = _style.LineSpacing;
|
||||
charSpacing = _style.CharSpacing;
|
||||
fillColor = _fillColor.ToHexString();
|
||||
outlineColor = _outlineColor.ToHexString();
|
||||
}
|
||||
|
||||
public void OnAfterDeserialize() {
|
||||
_font = DecalConfig.GetFont(fontName);
|
||||
_style = new DecalTextStyle((FontStyles) style, vertical, lineSpacing, charSpacing);
|
||||
|
||||
if (!ParseUtil.TryParseColor32(fillColor, out _fillColor)) {
|
||||
Logging.LogWarning($"Improperly formatted color value for fill: '{fillColor}'");
|
||||
_fillColor = Color.magenta;
|
||||
}
|
||||
|
||||
if (!ParseUtil.TryParseColor32(outlineColor, out _outlineColor)) {
|
||||
Logging.LogWarning($"Improperly formatted color value for outline: '{outlineColor}'");
|
||||
_outlineColor = Color.magenta;
|
||||
}
|
||||
}
|
||||
|
||||
public override void OnDestroy() {
|
||||
if (HighLogic.LoadedSceneIsGame && _currentText != null) TextRenderer.UnregisterText(_currentText);
|
||||
|
||||
base.OnDestroy();
|
||||
}
|
||||
|
||||
protected override void OnDetach() {
|
||||
// close all UIs
|
||||
if (_textEntryController != null) _textEntryController.Close();
|
||||
if (_fillColorPickerController != null) _fillColorPickerController.Close();
|
||||
if (_outlineColorPickerController != null) _outlineColorPickerController.Close();
|
||||
|
||||
base.OnDetach();
|
||||
}
|
||||
|
||||
private void UpdateTextRecursive() {
|
||||
UpdateText();
|
||||
|
||||
foreach (var counterpart in part.symmetryCounterparts) {
|
||||
var decal = counterpart.GetComponent<ModuleConformalText>();
|
||||
decal.text = text;
|
||||
decal._font = _font;
|
||||
decal._style = _style;
|
||||
|
||||
decal._currentJob = _currentJob;
|
||||
decal._currentText = _currentText;
|
||||
decal.UpdateText();
|
||||
}
|
||||
}
|
||||
|
||||
private void UpdateText() {
|
||||
// Render text
|
||||
var newText = new DecalText(text, _font, _style);
|
||||
var output = TextRenderer.UpdateTextNow(_currentText, newText);
|
||||
_currentText = newText;
|
||||
|
||||
UpdateTexture(output);
|
||||
|
||||
// TODO: ASYNC RENDERING
|
||||
// var newText = new DecalText(text, _font, _style);
|
||||
// _currentJob = TextRenderer.UpdateText(_currentText, newText, UpdateTexture);
|
||||
// _currentText = newText;
|
||||
}
|
||||
|
||||
public void UpdateTexture(TextRenderOutput output) {
|
||||
_decalTextureProperty.Texture = output.Texture;
|
||||
_decalTextureProperty.SetTile(output.Window);
|
||||
|
||||
UpdateMaterials();
|
||||
UpdateScale();
|
||||
}
|
||||
|
||||
protected override void UpdateMaterials() {
|
||||
_fillEnabledProperty.value = fillEnabled;
|
||||
_fillColorProperty.color = _fillColor;
|
||||
|
||||
_outlineEnabledProperty.value = outlineEnabled;
|
||||
_outlineColorProperty.color = _outlineColor;
|
||||
_outlineWidthProperty.value = outlineWidth;
|
||||
|
||||
base.UpdateMaterials();
|
||||
}
|
||||
|
||||
protected override void UpdateTweakables() {
|
||||
var fillEnabledField = Fields[nameof(fillEnabled)];
|
||||
var fillColorEvent = Events["SetFillColor"];
|
||||
|
||||
var outlineEnabledField = Fields[nameof(outlineEnabled)];
|
||||
var outlineWidthField = Fields[nameof(outlineWidth)];
|
||||
var outlineColorEvent = Events["SetOutlineColor"];
|
||||
|
||||
fillColorEvent.guiActiveEditor = fillEnabled;
|
||||
outlineWidthField.guiActiveEditor = outlineEnabled;
|
||||
outlineColorEvent.guiActiveEditor = outlineEnabled;
|
||||
|
||||
((UI_Toggle) fillEnabledField.uiControlEditor).onFieldChanged = OnFillToggle;
|
||||
((UI_Toggle) outlineEnabledField.uiControlEditor).onFieldChanged = OnOutlineToggle;
|
||||
((UI_FloatRange) outlineWidthField.uiControlEditor).onFieldChanged = OnOutlineWidthUpdate;
|
||||
|
||||
base.UpdateTweakables();
|
||||
}
|
||||
}
|
||||
}
|
@ -5,7 +5,7 @@ using System.Runtime.InteropServices;
|
||||
// General Information about an assembly is controlled through the following
|
||||
// set of attributes. Change these attribute values to modify the information
|
||||
// associated with an assembly.
|
||||
[assembly: AssemblyTitle("Conformal Decals")]
|
||||
[assembly: AssemblyTitle("ConformalDecals")]
|
||||
[assembly: AssemblyDescription("")]
|
||||
[assembly: AssemblyConfiguration("")]
|
||||
[assembly: AssemblyCompany("Cineboxandrew")]
|
||||
|
@ -1,34 +0,0 @@
|
||||
using System;
|
||||
using UnityEngine;
|
||||
|
||||
namespace ConformalDecals.Test {
|
||||
public class TestLayers : PartModule {
|
||||
|
||||
[KSPField(guiActive = true)]
|
||||
public int layer = 2;
|
||||
|
||||
public override void OnStart(StartState state) {
|
||||
base.OnStart(state);
|
||||
|
||||
|
||||
Part.layerMask.value |= (1 << 3);
|
||||
}
|
||||
|
||||
public void Update() {
|
||||
foreach (var collider in GameObject.FindObjectsOfType<Collider>()) {
|
||||
if (collider.gameObject.layer == 3) {
|
||||
Debug.Log($"Has layer 3: {collider.gameObject.name}");
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
[KSPEvent(guiActive = true, guiActiveEditor = true, guiName = "switch layers")]
|
||||
public void Switch() {
|
||||
Debug.Log(Part.layerMask.value);
|
||||
|
||||
var cube = part.FindModelTransform("test");
|
||||
layer = (layer + 1) % 32;
|
||||
cube.gameObject.layer = layer;
|
||||
}
|
||||
}
|
||||
}
|
99
Source/ConformalDecals/Text/DecalFont.cs
Normal file
99
Source/ConformalDecals/Text/DecalFont.cs
Normal file
@ -0,0 +1,99 @@
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using ConformalDecals.Util;
|
||||
using TMPro;
|
||||
using UniLinq;
|
||||
|
||||
namespace ConformalDecals.Text {
|
||||
public class DecalFont : IEquatable<DecalFont> {
|
||||
/// Human-readable name for the font
|
||||
public string Title { get; }
|
||||
|
||||
/// Internal name for the font
|
||||
public string Name => FontAsset.name;
|
||||
|
||||
/// The font asset itself
|
||||
public TMP_FontAsset FontAsset { get; }
|
||||
|
||||
/// Styles that are forced on for this font,
|
||||
/// e.g. smallcaps for a font without lower case characters
|
||||
public FontStyles FontStyle { get; }
|
||||
|
||||
public bool Bold => (FontStyle & FontStyles.Bold) != 0;
|
||||
|
||||
public bool Italic => (FontStyle & FontStyles.Italic) != 0;
|
||||
|
||||
public bool Underline => (FontStyle & FontStyles.Underline) != 0;
|
||||
|
||||
public bool SmallCaps => (FontStyle & FontStyles.SmallCaps) != 0;
|
||||
|
||||
/// Styles that are forced off for this font,
|
||||
/// e.g. underline for a font with no underscore character
|
||||
public FontStyles FontStyleMask { get; }
|
||||
|
||||
public bool BoldMask => (FontStyleMask & FontStyles.Bold) != 0;
|
||||
|
||||
public bool ItalicMask => (FontStyleMask & FontStyles.Italic) != 0;
|
||||
|
||||
public bool UnderlineMask => (FontStyleMask & FontStyles.Underline) != 0;
|
||||
|
||||
public bool SmallCapsMask => (FontStyleMask & FontStyles.SmallCaps) != 0;
|
||||
|
||||
|
||||
public DecalFont(ConfigNode node, IEnumerable<TMP_FontAsset> fontAssets) {
|
||||
if (node == null) throw new ArgumentNullException(nameof(node));
|
||||
if (fontAssets == null) throw new ArgumentNullException(nameof(fontAssets));
|
||||
|
||||
var name = ParseUtil.ParseString(node, "name");
|
||||
FontAsset = fontAssets.First(o => o.name == name);
|
||||
if (FontAsset == null) {
|
||||
throw new FormatException($"Could not find font asset named {name}");
|
||||
}
|
||||
|
||||
Title = ParseUtil.ParseString(node, "title", true, name);
|
||||
FontStyle = (FontStyles) ParseUtil.ParseInt(node, "style", true);
|
||||
FontStyleMask = (FontStyles) ParseUtil.ParseInt(node, "styleMask", true);
|
||||
}
|
||||
|
||||
|
||||
public void SetupSample(TMP_Text tmp) {
|
||||
if (tmp == null) throw new ArgumentNullException(nameof(tmp));
|
||||
if (FontAsset == null) throw new InvalidOperationException("DecalFont has not been initialized and Font is null.");
|
||||
|
||||
tmp.text = Title;
|
||||
tmp.font = FontAsset;
|
||||
tmp.fontStyle = FontStyle;
|
||||
}
|
||||
|
||||
public bool Equals(DecalFont other) {
|
||||
if (ReferenceEquals(null, other)) return false;
|
||||
if (ReferenceEquals(this, other)) return true;
|
||||
return Title == other.Title && Equals(FontAsset, other.FontAsset) && FontStyle == other.FontStyle && FontStyleMask == other.FontStyleMask;
|
||||
}
|
||||
|
||||
public override bool Equals(object obj) {
|
||||
if (ReferenceEquals(null, obj)) return false;
|
||||
if (ReferenceEquals(this, obj)) return true;
|
||||
if (obj.GetType() != this.GetType()) return false;
|
||||
return Equals((DecalFont) obj);
|
||||
}
|
||||
|
||||
public override int GetHashCode() {
|
||||
unchecked {
|
||||
var hashCode = (Title != null ? Title.GetHashCode() : 0);
|
||||
hashCode = (hashCode * 397) ^ (FontAsset != null ? FontAsset.GetHashCode() : 0);
|
||||
hashCode = (hashCode * 397) ^ (int) FontStyle;
|
||||
hashCode = (hashCode * 397) ^ (int) FontStyleMask;
|
||||
return hashCode;
|
||||
}
|
||||
}
|
||||
|
||||
public static bool operator ==(DecalFont left, DecalFont right) {
|
||||
return Equals(left, right);
|
||||
}
|
||||
|
||||
public static bool operator !=(DecalFont left, DecalFont right) {
|
||||
return !Equals(left, right);
|
||||
}
|
||||
}
|
||||
}
|
64
Source/ConformalDecals/Text/DecalText.cs
Normal file
64
Source/ConformalDecals/Text/DecalText.cs
Normal file
@ -0,0 +1,64 @@
|
||||
using System;
|
||||
using System.Text.RegularExpressions;
|
||||
|
||||
namespace ConformalDecals.Text {
|
||||
public class DecalText : IEquatable<DecalText> {
|
||||
/// Raw text contents
|
||||
public string Text { get; }
|
||||
|
||||
/// Font asset used by this text snippet
|
||||
public DecalFont Font { get; }
|
||||
|
||||
/// Style used by this text snippet
|
||||
public DecalTextStyle Style { get; }
|
||||
|
||||
/// The text formatted with newlines for vertical text
|
||||
public string FormattedText {
|
||||
get {
|
||||
if (Style.Vertical) {
|
||||
return Regex.Replace(Text, @"(.)", "$1\n");
|
||||
}
|
||||
else {
|
||||
return Text;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
public DecalText(string text, DecalFont font, DecalTextStyle style) {
|
||||
if (font == null) throw new ArgumentNullException(nameof(font));
|
||||
Text = text;
|
||||
Font = font;
|
||||
Style = style;
|
||||
}
|
||||
|
||||
public bool Equals(DecalText other) {
|
||||
if (ReferenceEquals(null, other)) return false;
|
||||
if (ReferenceEquals(this, other)) return true;
|
||||
return Text == other.Text && Equals(Font, other.Font) && Style.Equals(other.Style);
|
||||
}
|
||||
|
||||
public override bool Equals(object obj) {
|
||||
if (ReferenceEquals(null, obj)) return false;
|
||||
if (ReferenceEquals(this, obj)) return true;
|
||||
if (obj.GetType() != this.GetType()) return false;
|
||||
return Equals((DecalText) obj);
|
||||
}
|
||||
|
||||
public override int GetHashCode() {
|
||||
unchecked {
|
||||
var hashCode = (Text != null ? Text.GetHashCode() : 0);
|
||||
hashCode = (hashCode * 397) ^ (Font != null ? Font.GetHashCode() : 0);
|
||||
hashCode = (hashCode * 397) ^ Style.GetHashCode();
|
||||
return hashCode;
|
||||
}
|
||||
}
|
||||
|
||||
public static bool operator ==(DecalText left, DecalText right) {
|
||||
return Equals(left, right);
|
||||
}
|
||||
|
||||
public static bool operator !=(DecalText left, DecalText right) {
|
||||
return !Equals(left, right);
|
||||
}
|
||||
}
|
||||
}
|
101
Source/ConformalDecals/Text/DecalTextStyle.cs
Normal file
101
Source/ConformalDecals/Text/DecalTextStyle.cs
Normal file
@ -0,0 +1,101 @@
|
||||
using System;
|
||||
using TMPro;
|
||||
using UnityEngine;
|
||||
|
||||
// ReSharper disable NonReadonlyMemberInGetHashCode
|
||||
|
||||
namespace ConformalDecals.Text {
|
||||
public struct DecalTextStyle : IEquatable<DecalTextStyle> {
|
||||
private FontStyles _fontStyle;
|
||||
private bool _vertical;
|
||||
private float _lineSpacing;
|
||||
private float _charSpacing;
|
||||
|
||||
public FontStyles FontStyle {
|
||||
get => _fontStyle;
|
||||
set => _fontStyle = value;
|
||||
}
|
||||
|
||||
public bool Bold {
|
||||
get => (FontStyle & FontStyles.Bold) != 0;
|
||||
set {
|
||||
if (value) FontStyle |= FontStyles.Bold;
|
||||
else FontStyle &= ~FontStyles.Bold;
|
||||
}
|
||||
}
|
||||
|
||||
public bool Italic {
|
||||
get => (FontStyle & FontStyles.Italic) != 0;
|
||||
set {
|
||||
if (value) FontStyle |= FontStyles.Italic;
|
||||
else FontStyle &= ~FontStyles.Italic;
|
||||
}
|
||||
}
|
||||
|
||||
public bool Underline {
|
||||
get => (FontStyle & FontStyles.Underline) != 0;
|
||||
set {
|
||||
if (value) FontStyle |= FontStyles.Underline;
|
||||
else FontStyle &= ~FontStyles.Underline;
|
||||
}
|
||||
}
|
||||
|
||||
public bool SmallCaps {
|
||||
get => (FontStyle & FontStyles.SmallCaps) != 0;
|
||||
set {
|
||||
if (value) FontStyle |= FontStyles.SmallCaps;
|
||||
else FontStyle &= ~FontStyles.SmallCaps;
|
||||
}
|
||||
}
|
||||
|
||||
public bool Vertical {
|
||||
get => _vertical;
|
||||
set => _vertical = value;
|
||||
}
|
||||
|
||||
public float LineSpacing {
|
||||
get => _lineSpacing;
|
||||
set => _lineSpacing = value;
|
||||
}
|
||||
|
||||
public float CharSpacing {
|
||||
get => _charSpacing;
|
||||
set => _charSpacing = value;
|
||||
}
|
||||
|
||||
public DecalTextStyle(FontStyles fontStyle, bool vertical, float lineSpacing, float charSpacing) {
|
||||
_fontStyle = fontStyle;
|
||||
_vertical = vertical;
|
||||
_lineSpacing = lineSpacing;
|
||||
_charSpacing = charSpacing;
|
||||
}
|
||||
|
||||
public bool Equals(DecalTextStyle other) {
|
||||
return FontStyle == other.FontStyle && Vertical == other.Vertical &&
|
||||
Mathf.Approximately(LineSpacing, other.LineSpacing) &&
|
||||
Mathf.Approximately(CharSpacing, other.CharSpacing);
|
||||
}
|
||||
|
||||
public override bool Equals(object obj) {
|
||||
return obj is DecalTextStyle other && Equals(other);
|
||||
}
|
||||
|
||||
public override int GetHashCode() {
|
||||
unchecked {
|
||||
var hashCode = (int) FontStyle;
|
||||
hashCode = (hashCode * 397) ^ Vertical.GetHashCode();
|
||||
hashCode = (hashCode * 397) ^ LineSpacing.GetHashCode();
|
||||
hashCode = (hashCode * 397) ^ CharSpacing.GetHashCode();
|
||||
return hashCode;
|
||||
}
|
||||
}
|
||||
|
||||
public static bool operator ==(DecalTextStyle left, DecalTextStyle right) {
|
||||
return left.Equals(right);
|
||||
}
|
||||
|
||||
public static bool operator !=(DecalTextStyle left, DecalTextStyle right) {
|
||||
return !left.Equals(right);
|
||||
}
|
||||
}
|
||||
}
|
38
Source/ConformalDecals/Text/FontLoader.cs
Normal file
38
Source/ConformalDecals/Text/FontLoader.cs
Normal file
@ -0,0 +1,38 @@
|
||||
using System.IO;
|
||||
using System.Collections;
|
||||
using System.Collections.Generic;
|
||||
using ConformalDecals.Util;
|
||||
using TMPro;
|
||||
using UniLinq;
|
||||
using UnityEngine;
|
||||
|
||||
namespace ConformalDecals.Text {
|
||||
/// KSP database loader for KSPFont files which contain TextMeshPro font assets
|
||||
[DatabaseLoaderAttrib(new[] {"kspfont"})]
|
||||
public class FontLoader : DatabaseLoader<GameDatabase.TextureInfo> {
|
||||
private const string FallbackName = "NotoSans-Regular SDF";
|
||||
private static TMP_FontAsset _fallbackFont;
|
||||
|
||||
public override IEnumerator Load(UrlDir.UrlFile urlFile, FileInfo fileInfo) {
|
||||
if (_fallbackFont == null) {
|
||||
_fallbackFont = Resources.FindObjectsOfTypeAll<TMP_FontAsset>().First(o => o.name == FallbackName);
|
||||
if (_fallbackFont == null) Logging.LogError($"Could not find fallback font '{FallbackName}'");
|
||||
}
|
||||
|
||||
Logging.Log($"Loading font file '{urlFile.fullPath}'");
|
||||
var bundle = AssetBundle.LoadFromFile(urlFile.fullPath);
|
||||
if (!bundle) {
|
||||
Logging.Log($"Could not load font asset {urlFile.fullPath}");
|
||||
}
|
||||
else {
|
||||
var loadedFonts = bundle.LoadAllAssets<TMP_FontAsset>();
|
||||
foreach (var font in loadedFonts) {
|
||||
Logging.Log($"Adding font {font.name}");
|
||||
font.fallbackFontAssets.Add(_fallbackFont);
|
||||
}
|
||||
}
|
||||
|
||||
yield break;
|
||||
}
|
||||
}
|
||||
}
|
35
Source/ConformalDecals/Text/TextRenderJob.cs
Normal file
35
Source/ConformalDecals/Text/TextRenderJob.cs
Normal file
@ -0,0 +1,35 @@
|
||||
using System;
|
||||
using UnityEngine.Events;
|
||||
|
||||
namespace ConformalDecals.Text {
|
||||
public class TextRenderJob {
|
||||
public DecalText OldText { get; }
|
||||
public DecalText NewText { get; }
|
||||
public bool Needed { get; private set; }
|
||||
public bool IsStarted { get; private set; }
|
||||
public bool IsDone { get; private set; }
|
||||
|
||||
public readonly TextRenderer.TextRenderEvent onRenderFinished = new TextRenderer.TextRenderEvent();
|
||||
|
||||
public TextRenderJob(DecalText oldText, DecalText newText, UnityAction<TextRenderOutput> renderFinishedCallback) {
|
||||
OldText = oldText;
|
||||
NewText = newText ?? throw new ArgumentNullException(nameof(newText));
|
||||
Needed = true;
|
||||
|
||||
if (renderFinishedCallback != null) onRenderFinished.AddListener(renderFinishedCallback);
|
||||
}
|
||||
|
||||
public void Cancel() {
|
||||
Needed = false;
|
||||
}
|
||||
|
||||
public void Start() {
|
||||
IsStarted = true;
|
||||
}
|
||||
|
||||
public void Finish(TextRenderOutput output) {
|
||||
IsDone = true;
|
||||
onRenderFinished.Invoke(output);
|
||||
}
|
||||
}
|
||||
}
|
20
Source/ConformalDecals/Text/TextRenderOutput.cs
Normal file
20
Source/ConformalDecals/Text/TextRenderOutput.cs
Normal file
@ -0,0 +1,20 @@
|
||||
using UnityEngine;
|
||||
|
||||
namespace ConformalDecals.Text {
|
||||
/// Texture render output, used for cacheing and is the datastructure returned to the ModuleConformalText class
|
||||
public class TextRenderOutput {
|
||||
/// Texture with the rendered text
|
||||
public Texture2D Texture { get; private set; }
|
||||
|
||||
/// The rectangle that the rendered text takes up within the texture
|
||||
public Rect Window { get; private set; }
|
||||
|
||||
/// The number of users for this render output. If 0, it can be discarded from the cache and the texture reused
|
||||
public int UserCount { get; set; }
|
||||
|
||||
public TextRenderOutput(Texture2D texture, Rect window) {
|
||||
Texture = texture;
|
||||
Window = window;
|
||||
}
|
||||
}
|
||||
}
|
276
Source/ConformalDecals/Text/TextRenderer.cs
Normal file
276
Source/ConformalDecals/Text/TextRenderer.cs
Normal file
@ -0,0 +1,276 @@
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using ConformalDecals.Util;
|
||||
using TMPro;
|
||||
using UnityEngine;
|
||||
using UnityEngine.Events;
|
||||
|
||||
namespace ConformalDecals.Text {
|
||||
// TODO: Testing shows the job system is unnecessary, so remove job system code.
|
||||
|
||||
/// Class handing text rendering.
|
||||
/// Is a singleton referencing a single gameobject in the scene which contains the TextMeshPro component
|
||||
[KSPAddon(KSPAddon.Startup.Instantly, true)]
|
||||
public class TextRenderer : MonoBehaviour {
|
||||
/// Texture format used for returned textures.
|
||||
/// Unfortunately due to how Unity textures work, this cannot be R8 or Alpha8,
|
||||
/// so theres always a superfluous green channel using memory
|
||||
public const TextureFormat TextTextureFormat = TextureFormat.RG16;
|
||||
|
||||
/// Render Texture format used when rendering
|
||||
public const RenderTextureFormat TextRenderTextureFormat = RenderTextureFormat.R8;
|
||||
|
||||
/// The text renderer object within the scene which contains the TextMeshPro component used for rendering.
|
||||
public static TextRenderer Instance {
|
||||
get {
|
||||
if (!_instance._isSetup) {
|
||||
_instance.Setup();
|
||||
}
|
||||
|
||||
return _instance;
|
||||
}
|
||||
}
|
||||
|
||||
/// Text Render unityevent, used with the job system to signal render completion
|
||||
[Serializable]
|
||||
public class TextRenderEvent : UnityEvent<TextRenderOutput> { }
|
||||
|
||||
private const string ShaderName = "ConformalDecals/Text Blit";
|
||||
private const int MaxTextureSize = 4096;
|
||||
private const float FontSize = 100;
|
||||
private const float PixelDensity = 5;
|
||||
|
||||
private static TextRenderer _instance;
|
||||
|
||||
private bool _isSetup;
|
||||
private TextMeshPro _tmp;
|
||||
private Shader _blitShader;
|
||||
|
||||
private static readonly Dictionary<DecalText, TextRenderOutput> RenderCache = new Dictionary<DecalText, TextRenderOutput>();
|
||||
private static readonly Queue<TextRenderJob> RenderJobs = new Queue<TextRenderJob>();
|
||||
|
||||
/// Update text using the job queue
|
||||
public static TextRenderJob UpdateText(DecalText oldText, DecalText newText, UnityAction<TextRenderOutput> renderFinishedCallback) {
|
||||
if (newText == null) throw new ArgumentNullException(nameof(newText));
|
||||
|
||||
var job = new TextRenderJob(oldText, newText, renderFinishedCallback);
|
||||
RenderJobs.Enqueue(job);
|
||||
return job;
|
||||
}
|
||||
|
||||
/// Update text immediately without using job queue
|
||||
public static TextRenderOutput UpdateTextNow(DecalText oldText, DecalText newText) {
|
||||
if (newText == null) throw new ArgumentNullException(nameof(newText));
|
||||
|
||||
return Instance.RunJob(new TextRenderJob(oldText, newText, null), out _);
|
||||
}
|
||||
|
||||
/// Unregister a user of a piece of text
|
||||
public static void UnregisterText(DecalText text) {
|
||||
if (RenderCache.TryGetValue(text, out var renderedText)) {
|
||||
renderedText.UserCount--;
|
||||
if (renderedText.UserCount <= 0) {
|
||||
RenderCache.Remove(text);
|
||||
Destroy(renderedText.Texture);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
private void Start() {
|
||||
if (_instance != null) {
|
||||
Logging.LogError("Duplicate TextRenderer created???");
|
||||
}
|
||||
|
||||
Logging.Log("Creating TextRenderer Object");
|
||||
_instance = this;
|
||||
DontDestroyOnLoad(gameObject);
|
||||
}
|
||||
|
||||
/// Setup this text renderer instance for rendering
|
||||
private void Setup() {
|
||||
if (_isSetup) return;
|
||||
|
||||
Logging.Log("Setting Up TextRenderer Object");
|
||||
|
||||
_tmp = gameObject.AddComponent<TextMeshPro>();
|
||||
_tmp.renderer.enabled = false; // dont automatically render
|
||||
|
||||
_blitShader = Shabby.Shabby.FindShader(ShaderName);
|
||||
if (_blitShader == null) Logging.LogError($"Could not find text blit shader named '{ShaderName}'");
|
||||
|
||||
_isSetup = true;
|
||||
}
|
||||
|
||||
/// Run a text render job
|
||||
private TextRenderOutput RunJob(TextRenderJob job, out bool renderNeeded) {
|
||||
if (!job.Needed) {
|
||||
renderNeeded = false;
|
||||
return null;
|
||||
}
|
||||
|
||||
job.Start();
|
||||
|
||||
Texture2D texture = null;
|
||||
if (job.OldText != null && RenderCache.TryGetValue(job.OldText, out var oldRender)) {
|
||||
// old output still exists
|
||||
|
||||
oldRender.UserCount--;
|
||||
|
||||
if (oldRender.UserCount <= 0) {
|
||||
// this is the only usage of this output, so we are free to re-render into the texture
|
||||
|
||||
texture = oldRender.Texture;
|
||||
RenderCache.Remove(job.OldText);
|
||||
}
|
||||
}
|
||||
|
||||
// now that all old references are handled, begin rendering the new output
|
||||
|
||||
if (RenderCache.TryGetValue(job.NewText, out var renderOutput)) {
|
||||
renderNeeded = false;
|
||||
}
|
||||
else {
|
||||
renderNeeded = true;
|
||||
|
||||
renderOutput = RenderText(job.NewText, texture);
|
||||
RenderCache.Add(job.NewText, renderOutput);
|
||||
}
|
||||
|
||||
renderOutput.UserCount++;
|
||||
|
||||
job.Finish(renderOutput);
|
||||
return renderOutput;
|
||||
}
|
||||
|
||||
/// Render a piece of text to a given texture
|
||||
public TextRenderOutput RenderText(DecalText text, Texture2D texture) {
|
||||
if (text == null) throw new ArgumentNullException(nameof(text));
|
||||
if (_tmp == null) throw new InvalidOperationException("TextMeshPro object not yet created.");
|
||||
|
||||
// SETUP TMP OBJECT FOR RENDERING
|
||||
_tmp.text = text.FormattedText;
|
||||
_tmp.font = text.Font.FontAsset;
|
||||
_tmp.fontStyle = text.Style.FontStyle | text.Font.FontStyle;
|
||||
_tmp.lineSpacing = text.Style.LineSpacing;
|
||||
_tmp.characterSpacing = text.Style.CharSpacing;
|
||||
|
||||
_tmp.extraPadding = true;
|
||||
_tmp.enableKerning = true;
|
||||
_tmp.enableWordWrapping = false;
|
||||
_tmp.overflowMode = TextOverflowModes.Overflow;
|
||||
_tmp.alignment = TextAlignmentOptions.Center;
|
||||
_tmp.fontSize = FontSize;
|
||||
|
||||
// GENERATE MESH
|
||||
_tmp.ClearMesh(false);
|
||||
_tmp.ForceMeshUpdate();
|
||||
|
||||
var meshFilters = gameObject.GetComponentsInChildren<MeshFilter>();
|
||||
var meshes = new Mesh[meshFilters.Length];
|
||||
var materials = new Material[meshFilters.Length];
|
||||
|
||||
var bounds = new Bounds();
|
||||
|
||||
// SETUP MATERIALS AND BOUNDS
|
||||
for (int i = 0; i < meshFilters.Length; i++) {
|
||||
var renderer = meshFilters[i].gameObject.GetComponent<MeshRenderer>();
|
||||
|
||||
meshes[i] = meshFilters[i].mesh;
|
||||
if (i == 0) meshes[i] = _tmp.mesh;
|
||||
|
||||
materials[i] = Instantiate(renderer.material);
|
||||
materials[i].shader = _blitShader;
|
||||
|
||||
if (renderer == null) throw new FormatException($"Object {meshFilters[i].gameObject.name} has filter but no renderer");
|
||||
if (meshes[i] == null) throw new FormatException($"Object {meshFilters[i].gameObject.name} has a null mesh");
|
||||
|
||||
if (i == 0) {
|
||||
bounds = meshes[i].bounds;
|
||||
}
|
||||
else {
|
||||
bounds.Encapsulate(meshes[i].bounds);
|
||||
}
|
||||
}
|
||||
|
||||
// CALCULATE SIZES
|
||||
var size = bounds.size * PixelDensity;
|
||||
var textureSize = new Vector2Int {
|
||||
x = Mathf.NextPowerOfTwo((int) size.x),
|
||||
y = Mathf.NextPowerOfTwo((int) size.y)
|
||||
};
|
||||
|
||||
if (textureSize.x == 0 || textureSize.y == 0) {
|
||||
Logging.LogWarning("No text present or error in texture size calculation. Aborting.");
|
||||
return new TextRenderOutput(Texture2D.blackTexture, Rect.zero);
|
||||
}
|
||||
|
||||
// make sure texture isnt too big, scale it down if it is
|
||||
// this is just so you dont crash the game by pasting in the entire script of The Bee Movie
|
||||
if (textureSize.x > MaxTextureSize) {
|
||||
textureSize.y /= textureSize.x / MaxTextureSize;
|
||||
textureSize.x = MaxTextureSize;
|
||||
}
|
||||
|
||||
if (textureSize.y > MaxTextureSize) {
|
||||
textureSize.x /= textureSize.y / MaxTextureSize;
|
||||
textureSize.y = MaxTextureSize;
|
||||
}
|
||||
|
||||
// scale up everything to fit the texture for maximum usage
|
||||
float sizeRatio = Mathf.Min(textureSize.x / size.x, textureSize.y / size.y);
|
||||
|
||||
// calculate where in the texture the used area actually is
|
||||
var window = new Rect {
|
||||
size = size * sizeRatio,
|
||||
center = (Vector2) textureSize / 2
|
||||
};
|
||||
|
||||
// SETUP TEXTURE
|
||||
if (texture == null) {
|
||||
texture = new Texture2D(textureSize.x, textureSize.y, TextTextureFormat, true);
|
||||
}
|
||||
else if (texture.width != textureSize.x || texture.height != textureSize.y || texture.format != TextTextureFormat) {
|
||||
texture.Resize(textureSize.x, textureSize.y, TextTextureFormat, true);
|
||||
}
|
||||
|
||||
// GENERATE PROJECTION MATRIX
|
||||
var halfSize = (Vector2) textureSize / PixelDensity / 2 / sizeRatio;
|
||||
var matrix = Matrix4x4.Ortho(bounds.center.x - halfSize.x, bounds.center.x + halfSize.x,
|
||||
bounds.center.y - halfSize.y, bounds.center.y + halfSize.y, -1, 1);
|
||||
|
||||
// GET RENDERTEX
|
||||
var renderTex = RenderTexture.GetTemporary(textureSize.x, textureSize.y, 0, TextRenderTextureFormat, RenderTextureReadWrite.Linear, 1);
|
||||
renderTex.autoGenerateMips = false;
|
||||
|
||||
// RENDER
|
||||
Graphics.SetRenderTarget(renderTex);
|
||||
GL.PushMatrix();
|
||||
GL.LoadProjectionMatrix(matrix);
|
||||
GL.Clear(false, true, Color.black);
|
||||
|
||||
for (var i = 0; i < meshes.Length; i++) {
|
||||
if (meshes[i].vertexCount >= 3) {
|
||||
materials[i].SetPass(0);
|
||||
Graphics.DrawMeshNow(meshes[i], Matrix4x4.identity);
|
||||
}
|
||||
}
|
||||
|
||||
GL.PopMatrix();
|
||||
|
||||
// COPY TEXTURE BACK INTO RAM
|
||||
RenderTexture.active = renderTex;
|
||||
texture.ReadPixels(new Rect(0, 0, textureSize.x, textureSize.y), 0, 0, true);
|
||||
texture.Apply();
|
||||
|
||||
// RELEASE RENDERTEX
|
||||
RenderTexture.ReleaseTemporary(renderTex);
|
||||
|
||||
// CLEAR SUBMESHES
|
||||
for (int i = 0; i < transform.childCount; i++) {
|
||||
Destroy(transform.GetChild(i).gameObject);
|
||||
}
|
||||
|
||||
return new TextRenderOutput(texture, window);
|
||||
}
|
||||
}
|
||||
}
|
176
Source/ConformalDecals/UI/BoxSlider.cs
Normal file
176
Source/ConformalDecals/UI/BoxSlider.cs
Normal file
@ -0,0 +1,176 @@
|
||||
using System;
|
||||
using UnityEngine;
|
||||
using UnityEngine.Events;
|
||||
using UnityEngine.EventSystems;
|
||||
using UnityEngine.UI;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
[AddComponentMenu("UI/BoxSlider", 35)]
|
||||
[RequireComponent(typeof(RectTransform))]
|
||||
public class BoxSlider : Selectable, IDragHandler, IInitializePotentialDragHandler, ICanvasElement {
|
||||
[Serializable]
|
||||
public class BoxSliderEvent : UnityEvent<Vector2> { }
|
||||
|
||||
[SerializeField] private RectTransform _handleRect;
|
||||
[SerializeField] private Vector2 _value = Vector2.zero;
|
||||
|
||||
public RectTransform HandleRect {
|
||||
get => _handleRect;
|
||||
set {
|
||||
if (value == null) throw new ArgumentNullException(nameof(value));
|
||||
if (value != _handleRect) {
|
||||
_handleRect = value;
|
||||
UpdateCachedReferences();
|
||||
UpdateVisuals();
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
public Vector2 Value {
|
||||
get => _value;
|
||||
set {
|
||||
_value = value;
|
||||
_onValueChanged.Invoke(value);
|
||||
UpdateVisuals();
|
||||
}
|
||||
}
|
||||
|
||||
[Space(6)]
|
||||
|
||||
// Allow for delegate-based subscriptions for faster events than 'eventReceiver', and allowing for multiple receivers.
|
||||
[SerializeField]
|
||||
private BoxSliderEvent _onValueChanged = new BoxSliderEvent();
|
||||
|
||||
public BoxSliderEvent OnValueChanged {
|
||||
get => _onValueChanged;
|
||||
set => _onValueChanged = value;
|
||||
}
|
||||
|
||||
// Private fields
|
||||
|
||||
private Transform _handleTransform;
|
||||
private RectTransform _handleContainerRect;
|
||||
|
||||
// The offset from handle position to mouse down position
|
||||
private Vector2 _offset = Vector2.zero;
|
||||
|
||||
#if UNITY_EDITOR
|
||||
protected override void OnValidate() {
|
||||
base.OnValidate();
|
||||
|
||||
//Onvalidate is called before OnEnabled. We need to make sure not to touch any other objects before OnEnable is run.
|
||||
if (IsActive()) {
|
||||
UpdateCachedReferences();
|
||||
// Update rects since other things might affect them even if value didn't change.
|
||||
UpdateVisuals();
|
||||
}
|
||||
|
||||
#if UNITY_2018_3_OR_NEWER
|
||||
|
||||
if (!UnityEditor.PrefabUtility.IsPartOfPrefabAsset(this) && !Application.isPlaying)
|
||||
CanvasUpdateRegistry.RegisterCanvasElementForLayoutRebuild(this);
|
||||
|
||||
#else
|
||||
var prefabType = UnityEditor.PrefabUtility.GetPrefabType(this);
|
||||
if (prefabType != UnityEditor.PrefabType.Prefab && !Application.isPlaying)
|
||||
CanvasUpdateRegistry.RegisterCanvasElementForLayoutRebuild(this);
|
||||
#endif
|
||||
}
|
||||
#endif // if UNITY_EDITOR
|
||||
|
||||
public virtual void Rebuild(CanvasUpdate executing) {
|
||||
#if UNITY_EDITOR
|
||||
if (executing == CanvasUpdate.Prelayout)
|
||||
OnValueChanged.Invoke(Value);
|
||||
#endif
|
||||
}
|
||||
|
||||
public void LayoutComplete() { }
|
||||
|
||||
public void GraphicUpdateComplete() { }
|
||||
|
||||
protected override void OnEnable() {
|
||||
base.OnEnable();
|
||||
UpdateCachedReferences();
|
||||
// Update rects since they need to be initialized correctly.
|
||||
UpdateVisuals();
|
||||
}
|
||||
|
||||
private void UpdateCachedReferences() {
|
||||
if (_handleRect) {
|
||||
_handleTransform = _handleRect.transform;
|
||||
if (_handleTransform.parent != null)
|
||||
_handleContainerRect = _handleTransform.parent.GetComponent<RectTransform>();
|
||||
}
|
||||
else {
|
||||
_handleContainerRect = null;
|
||||
}
|
||||
}
|
||||
|
||||
protected override void OnRectTransformDimensionsChange() {
|
||||
base.OnRectTransformDimensionsChange();
|
||||
UpdateVisuals();
|
||||
}
|
||||
|
||||
// Force-update the slider. Useful if you've changed the properties and want it to update visually.
|
||||
private void UpdateVisuals() {
|
||||
if (_handleContainerRect != null) {
|
||||
_handleRect.anchorMin = _value;
|
||||
_handleRect.anchorMax = _value;
|
||||
}
|
||||
}
|
||||
|
||||
// Update the slider's position based on the mouse.
|
||||
private void UpdateDrag(PointerEventData eventData, Camera cam) {
|
||||
var clickRect = _handleContainerRect;
|
||||
if (clickRect != null && clickRect.rect.size[0] > 0) {
|
||||
if (!RectTransformUtility.ScreenPointToLocalPointInRectangle(clickRect, eventData.position, cam, out var localCursor))
|
||||
return;
|
||||
|
||||
var rect = clickRect.rect;
|
||||
localCursor -= rect.position;
|
||||
|
||||
Vector2 newVal;
|
||||
newVal.x = Mathf.Clamp01((localCursor - _offset).x / rect.size.x);
|
||||
newVal.y = Mathf.Clamp01((localCursor - _offset).y / rect.size.y);
|
||||
|
||||
Value = newVal;
|
||||
}
|
||||
}
|
||||
|
||||
private bool MayDrag(PointerEventData eventData) {
|
||||
return IsActive() && IsInteractable() && eventData.button == PointerEventData.InputButton.Left;
|
||||
}
|
||||
|
||||
public override void OnPointerDown(PointerEventData eventData) {
|
||||
if (!MayDrag(eventData))
|
||||
return;
|
||||
|
||||
base.OnPointerDown(eventData);
|
||||
|
||||
_offset = Vector2.zero;
|
||||
if (_handleContainerRect != null && RectTransformUtility.RectangleContainsScreenPoint(_handleRect, eventData.position, eventData.enterEventCamera)) {
|
||||
Vector2 localMousePos;
|
||||
if (RectTransformUtility.ScreenPointToLocalPointInRectangle(_handleRect, eventData.position, eventData.pressEventCamera, out localMousePos))
|
||||
_offset = localMousePos;
|
||||
|
||||
_offset.y = -_offset.y;
|
||||
}
|
||||
else {
|
||||
// Outside the slider handle - jump to this point instead
|
||||
UpdateDrag(eventData, eventData.pressEventCamera);
|
||||
}
|
||||
}
|
||||
|
||||
public virtual void OnDrag(PointerEventData eventData) {
|
||||
if (!MayDrag(eventData))
|
||||
return;
|
||||
|
||||
UpdateDrag(eventData, eventData.pressEventCamera);
|
||||
}
|
||||
|
||||
public virtual void OnInitializePotentialDrag(PointerEventData eventData) {
|
||||
eventData.useDragThreshold = false;
|
||||
}
|
||||
}
|
||||
}
|
63
Source/ConformalDecals/UI/ColorBoxSlider.cs
Normal file
63
Source/ConformalDecals/UI/ColorBoxSlider.cs
Normal file
@ -0,0 +1,63 @@
|
||||
using UnityEngine;
|
||||
using UnityEngine.UI;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
public class ColorBoxSlider : MonoBehaviour {
|
||||
[SerializeField] public ColorPickerController.SVUpdateEvent onValueChanged = new ColorPickerController.SVUpdateEvent();
|
||||
|
||||
[SerializeField] private Vector2 _value;
|
||||
|
||||
[SerializeField] private BoxSlider _slider;
|
||||
[SerializeField] private Image _image;
|
||||
|
||||
private bool _ignoreUpdates;
|
||||
|
||||
public Vector2 Value {
|
||||
get => _value;
|
||||
set {
|
||||
_value.x = Mathf.Clamp01(value.x);
|
||||
_value.y = Mathf.Clamp01(value.y);
|
||||
UpdateSlider();
|
||||
UpdateChannels();
|
||||
}
|
||||
}
|
||||
|
||||
public void OnColorUpdate(Color rgb, Util.ColorHSV hsv) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
_image.material.SetColor(PropertyIDs._Color, (Vector4) hsv);
|
||||
|
||||
_value.x = hsv.s;
|
||||
_value.y = hsv.v;
|
||||
UpdateSlider();
|
||||
}
|
||||
|
||||
public void OnSliderUpdate(Vector2 value) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
_value = value;
|
||||
UpdateChannels();
|
||||
}
|
||||
|
||||
private void Awake() {
|
||||
var boxSlider = gameObject.GetComponentInChildren<BoxSlider>();
|
||||
boxSlider.OnValueChanged.AddListener(OnSliderUpdate);
|
||||
}
|
||||
|
||||
private void UpdateChannels() {
|
||||
_ignoreUpdates = true;
|
||||
|
||||
onValueChanged.Invoke(_value);
|
||||
|
||||
_ignoreUpdates = false;
|
||||
}
|
||||
|
||||
private void UpdateSlider() {
|
||||
_ignoreUpdates = true;
|
||||
|
||||
_slider.Value = _value;
|
||||
|
||||
_ignoreUpdates = false;
|
||||
}
|
||||
}
|
||||
}
|
79
Source/ConformalDecals/UI/ColorChannelSlider.cs
Normal file
79
Source/ConformalDecals/UI/ColorChannelSlider.cs
Normal file
@ -0,0 +1,79 @@
|
||||
using TMPro;
|
||||
using UnityEngine;
|
||||
using UnityEngine.UI;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
public class ColorChannelSlider : MonoBehaviour {
|
||||
[SerializeField] public ColorPickerController.ChannelUpdateEvent onChannelChanged = new ColorPickerController.ChannelUpdateEvent();
|
||||
|
||||
[SerializeField] private float _value;
|
||||
[SerializeField] private int _channel;
|
||||
[SerializeField] private bool _hsv;
|
||||
|
||||
[SerializeField] private Selectable _textBox;
|
||||
[SerializeField] private Slider _slider;
|
||||
[SerializeField] private Image _image;
|
||||
|
||||
private bool _ignoreUpdates;
|
||||
|
||||
public float Value {
|
||||
get => _value;
|
||||
set {
|
||||
_value = Mathf.Clamp01(value);
|
||||
UpdateSlider();
|
||||
UpdateTextbox();
|
||||
UpdateChannel();
|
||||
}
|
||||
}
|
||||
|
||||
public void OnColorUpdate(Color rgb, Util.ColorHSV hsv) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
_image.material.SetColor(PropertyIDs._Color, _hsv ? (Color) (Vector4) hsv : rgb);
|
||||
|
||||
_value = _hsv ? hsv[_channel] : rgb[_channel];
|
||||
UpdateSlider();
|
||||
UpdateTextbox();
|
||||
}
|
||||
|
||||
public void OnTextBoxUpdate(string text) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
if (byte.TryParse(text, out byte byteValue)) {
|
||||
_value = (float) byteValue / 255;
|
||||
UpdateSlider();
|
||||
UpdateChannel();
|
||||
}
|
||||
else {
|
||||
// value is invalid, reset value
|
||||
UpdateTextbox();
|
||||
}
|
||||
}
|
||||
|
||||
public void OnSliderUpdate(float value) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
_value = value;
|
||||
UpdateTextbox();
|
||||
UpdateChannel();
|
||||
}
|
||||
|
||||
private void UpdateChannel() {
|
||||
onChannelChanged.Invoke(_value, _channel, _hsv);
|
||||
}
|
||||
|
||||
private void UpdateSlider() {
|
||||
_ignoreUpdates = true;
|
||||
_slider.value = _value;
|
||||
_ignoreUpdates = false;
|
||||
}
|
||||
|
||||
private void UpdateTextbox() {
|
||||
if (_textBox == null) return;
|
||||
|
||||
_ignoreUpdates = true;
|
||||
((TMP_InputField) _textBox).text = ((byte) (255 * _value)).ToString();
|
||||
_ignoreUpdates = false;
|
||||
}
|
||||
}
|
||||
}
|
117
Source/ConformalDecals/UI/ColorPickerController.cs
Normal file
117
Source/ConformalDecals/UI/ColorPickerController.cs
Normal file
@ -0,0 +1,117 @@
|
||||
using System;
|
||||
using ConformalDecals.Util;
|
||||
using TMPro;
|
||||
using UnityEngine;
|
||||
using UnityEngine.Events;
|
||||
using UnityEngine.UI;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
public class ColorPickerController : MonoBehaviour {
|
||||
[Serializable]
|
||||
public class ColorUpdateEvent : UnityEvent<Color, Util.ColorHSV> { }
|
||||
|
||||
[Serializable]
|
||||
public class ChannelUpdateEvent : UnityEvent<float, int, bool> { }
|
||||
|
||||
[Serializable]
|
||||
public class SVUpdateEvent : UnityEvent<Vector2> { }
|
||||
|
||||
[SerializeField] public ColorUpdateEvent onColorChanged = new ColorUpdateEvent();
|
||||
|
||||
[SerializeField] private Color _value;
|
||||
[SerializeField] private Image _previewImage;
|
||||
[SerializeField] private Selectable _hexTextBox;
|
||||
|
||||
private bool _ignoreUpdate;
|
||||
|
||||
public Color RGB {
|
||||
get => _value;
|
||||
set {
|
||||
_value = value;
|
||||
OnColorUpdate();
|
||||
}
|
||||
}
|
||||
|
||||
public Util.ColorHSV HSV {
|
||||
get => Util.ColorHSV.RGB2HSV(_value);
|
||||
set {
|
||||
_value = Util.ColorHSV.HSV2RGB(value);
|
||||
OnColorUpdate();
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
public static ColorPickerController Create(Color rgb, UnityAction<Color, Util.ColorHSV> colorUpdateCallback) {
|
||||
var menu = Instantiate(UILoader.ColorPickerPrefab, MainCanvasUtil.MainCanvas.transform, true);
|
||||
menu.AddComponent<DragPanel>();
|
||||
MenuNavigation.SpawnMenuNavigation(menu, Navigation.Mode.Automatic, true);
|
||||
|
||||
var controller = menu.GetComponent<ColorPickerController>();
|
||||
controller.RGB = rgb;
|
||||
controller.onColorChanged.AddListener(colorUpdateCallback);
|
||||
return controller;
|
||||
}
|
||||
|
||||
public void Close() {
|
||||
Destroy(gameObject);
|
||||
}
|
||||
|
||||
public void OnChannelUpdate(float value, int channel, bool hsv) {
|
||||
if (hsv) {
|
||||
var newHSV = HSV;
|
||||
newHSV[channel] = value;
|
||||
HSV = newHSV;
|
||||
}
|
||||
else {
|
||||
var newRGB = RGB;
|
||||
newRGB[channel] = value;
|
||||
RGB = newRGB;
|
||||
}
|
||||
}
|
||||
|
||||
public void OnSVUpdate(Vector2 sv) {
|
||||
var newHSV = HSV;
|
||||
newHSV.s = sv.x;
|
||||
newHSV.v = sv.y;
|
||||
HSV = newHSV;
|
||||
}
|
||||
|
||||
public void OnColorUpdate() {
|
||||
onColorChanged.Invoke(RGB, HSV);
|
||||
_previewImage.material.SetColor(PropertyIDs._Color, RGB);
|
||||
UpdateHexColor();
|
||||
}
|
||||
|
||||
public void OnHexColorUpdate(string text) {
|
||||
if (_ignoreUpdate) return;
|
||||
|
||||
if (ParseUtil.TryParseHexColor(text, out var newRGB)) {
|
||||
RGB = newRGB;
|
||||
OnColorUpdate();
|
||||
}
|
||||
else {
|
||||
UpdateHexColor();
|
||||
}
|
||||
}
|
||||
|
||||
private void Awake() {
|
||||
foreach (var slider in gameObject.GetComponentsInChildren<ColorChannelSlider>()) {
|
||||
slider.onChannelChanged.AddListener(OnChannelUpdate);
|
||||
onColorChanged.AddListener(slider.OnColorUpdate);
|
||||
}
|
||||
|
||||
foreach (var box in gameObject.GetComponentsInChildren<ColorBoxSlider>()) {
|
||||
box.onValueChanged.AddListener(OnSVUpdate);
|
||||
onColorChanged.AddListener(box.OnColorUpdate);
|
||||
}
|
||||
}
|
||||
|
||||
private void UpdateHexColor() {
|
||||
_ignoreUpdate = true;
|
||||
var byteColor = (Color32) RGB;
|
||||
var hexColor = $"{byteColor.r:x2}{byteColor.g:x2}{byteColor.b:x2}";
|
||||
((TMP_InputField) _hexTextBox).text = hexColor;
|
||||
_ignoreUpdate = false;
|
||||
}
|
||||
}
|
||||
}
|
63
Source/ConformalDecals/UI/FontMenuController.cs
Normal file
63
Source/ConformalDecals/UI/FontMenuController.cs
Normal file
@ -0,0 +1,63 @@
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using ConformalDecals.Text;
|
||||
using UniLinq;
|
||||
using UnityEngine;
|
||||
using UnityEngine.Events;
|
||||
using UnityEngine.UI;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
public class FontMenuController : MonoBehaviour {
|
||||
[Serializable]
|
||||
public class FontUpdateEvent : UnityEvent<DecalFont> { }
|
||||
|
||||
[SerializeField] public FontUpdateEvent onFontChanged = new FontUpdateEvent();
|
||||
|
||||
[SerializeField] private GameObject _menuItem;
|
||||
[SerializeField] private GameObject _menuList;
|
||||
|
||||
private DecalFont _currentFont;
|
||||
|
||||
public static FontMenuController Create(IEnumerable<DecalFont> fonts, DecalFont currentFont, UnityAction<DecalFont> fontUpdateCallback) {
|
||||
var menu = Instantiate(UILoader.FontMenuPrefab, MainCanvasUtil.MainCanvas.transform, true);
|
||||
menu.AddComponent<DragPanel>();
|
||||
MenuNavigation.SpawnMenuNavigation(menu, Navigation.Mode.Automatic, true);
|
||||
|
||||
var controller = menu.GetComponent<FontMenuController>();
|
||||
controller._currentFont = currentFont;
|
||||
controller.onFontChanged.AddListener(fontUpdateCallback);
|
||||
|
||||
controller.Populate(fonts);
|
||||
return controller;
|
||||
}
|
||||
|
||||
public void Close() {
|
||||
Destroy(gameObject);
|
||||
}
|
||||
|
||||
public void OnFontSelected(DecalFont font) {
|
||||
_currentFont = font ?? throw new ArgumentNullException(nameof(font));
|
||||
onFontChanged.Invoke(_currentFont);
|
||||
}
|
||||
|
||||
public void Populate(IEnumerable<DecalFont> fonts) {
|
||||
if (fonts == null) throw new ArgumentNullException(nameof(fonts));
|
||||
|
||||
Toggle active = null;
|
||||
|
||||
foreach (var font in fonts.OrderBy(x => x.Title)) {
|
||||
var listItem = GameObject.Instantiate(_menuItem, _menuList.transform);
|
||||
listItem.name = font.Title;
|
||||
listItem.SetActive(true);
|
||||
|
||||
var fontItem = listItem.AddComponent<FontMenuItem>();
|
||||
fontItem.Font = font;
|
||||
fontItem.fontSelectionCallback = OnFontSelected;
|
||||
|
||||
if (font == _currentFont) active = fontItem.toggle;
|
||||
}
|
||||
|
||||
if (active != null) active.isOn = true;
|
||||
}
|
||||
}
|
||||
}
|
36
Source/ConformalDecals/UI/FontMenuItem.cs
Normal file
36
Source/ConformalDecals/UI/FontMenuItem.cs
Normal file
@ -0,0 +1,36 @@
|
||||
using System;
|
||||
using ConformalDecals.Text;
|
||||
using TMPro;
|
||||
using UnityEngine;
|
||||
using UnityEngine.UI;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
public class FontMenuItem : MonoBehaviour {
|
||||
public DecalFont Font {
|
||||
get => _font;
|
||||
set {
|
||||
_font = value;
|
||||
_font.SetupSample(_label);
|
||||
}
|
||||
}
|
||||
|
||||
public delegate void FontSelectionReceiver(DecalFont font);
|
||||
|
||||
public FontSelectionReceiver fontSelectionCallback;
|
||||
public Toggle toggle;
|
||||
|
||||
private DecalFont _font;
|
||||
private TMP_Text _label;
|
||||
|
||||
private void Awake() {
|
||||
_label = gameObject.GetComponentInChildren<TextMeshProUGUI>();
|
||||
toggle = gameObject.GetComponent<Toggle>();
|
||||
toggle.isOn = false;
|
||||
toggle.onValueChanged.AddListener(delegate { OnToggle(toggle); });
|
||||
}
|
||||
|
||||
public void OnToggle(Toggle change) {
|
||||
if (change.isOn) fontSelectionCallback?.Invoke(_font);
|
||||
}
|
||||
}
|
||||
}
|
272
Source/ConformalDecals/UI/TextEntryController.cs
Normal file
272
Source/ConformalDecals/UI/TextEntryController.cs
Normal file
@ -0,0 +1,272 @@
|
||||
using System;
|
||||
using ConformalDecals.Text;
|
||||
using ConformalDecals.Util;
|
||||
using TMPro;
|
||||
using UnityEngine;
|
||||
using UnityEngine.Events;
|
||||
using UnityEngine.UI;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
public class TextEntryController : MonoBehaviour {
|
||||
[Serializable]
|
||||
public class TextUpdateEvent : UnityEvent<string, DecalFont, DecalTextStyle> { }
|
||||
|
||||
[SerializeField] public TextUpdateEvent onValueChanged = new TextUpdateEvent();
|
||||
|
||||
[SerializeField] private Selectable _textBox;
|
||||
[SerializeField] private Button _fontButton;
|
||||
|
||||
[SerializeField] private Slider _lineSpacingSlider;
|
||||
[SerializeField] private Selectable _lineSpacingTextBox;
|
||||
|
||||
[SerializeField] private Slider _charSpacingSlider;
|
||||
[SerializeField] private Selectable _charSpacingTextBox;
|
||||
|
||||
[SerializeField] private Toggle _boldButton;
|
||||
[SerializeField] private Toggle _italicButton;
|
||||
[SerializeField] private Toggle _underlineButton;
|
||||
[SerializeField] private Toggle _smallCapsButton;
|
||||
[SerializeField] private Toggle _verticalButton;
|
||||
|
||||
private string _text;
|
||||
private DecalFont _font;
|
||||
private DecalTextStyle _style;
|
||||
private Vector2 _lineSpacingRange;
|
||||
private Vector2 _charSpacingRange;
|
||||
private TMP_InputField _textBoxTMP;
|
||||
|
||||
private FontMenuController _fontMenu;
|
||||
|
||||
private bool _ignoreUpdates;
|
||||
|
||||
public static TextEntryController Create(
|
||||
string text, DecalFont font, DecalTextStyle style,
|
||||
Vector2 lineSpacingRange, Vector2 charSpacingRange,
|
||||
UnityAction<string, DecalFont, DecalTextStyle> textUpdateCallback) {
|
||||
|
||||
var window = Instantiate(UILoader.TextEntryPrefab, MainCanvasUtil.MainCanvas.transform, true);
|
||||
window.AddComponent<DragPanel>();
|
||||
MenuNavigation.SpawnMenuNavigation(window, Navigation.Mode.Automatic, true);
|
||||
|
||||
var controller = window.GetComponent<TextEntryController>();
|
||||
controller._text = text;
|
||||
controller._font = font;
|
||||
controller._style = style;
|
||||
controller._lineSpacingRange = lineSpacingRange;
|
||||
controller._charSpacingRange = charSpacingRange;
|
||||
controller.onValueChanged.AddListener(textUpdateCallback);
|
||||
|
||||
return controller;
|
||||
}
|
||||
|
||||
public void Close() {
|
||||
if (_fontMenu != null) _fontMenu.Close();
|
||||
Destroy(gameObject);
|
||||
}
|
||||
|
||||
public void OnTextUpdate(string newText) {
|
||||
this._text = newText;
|
||||
|
||||
OnValueChanged();
|
||||
}
|
||||
|
||||
public void OnFontMenu() {
|
||||
if (_fontMenu == null) _fontMenu = FontMenuController.Create(DecalConfig.Fonts, _font, OnFontUpdate);
|
||||
}
|
||||
|
||||
public void OnFontUpdate(DecalFont font) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
_font = font;
|
||||
font.SetupSample(_fontButton.GetComponentInChildren<TextMeshProUGUI>());
|
||||
|
||||
_textBoxTMP.text = _text;
|
||||
_textBoxTMP.textComponent.fontStyle = _style.FontStyle | _font.FontStyle & ~_font.FontStyleMask;
|
||||
_textBoxTMP.fontAsset = _font.FontAsset;
|
||||
|
||||
UpdateStyleButtons();
|
||||
OnValueChanged();
|
||||
}
|
||||
|
||||
public void OnLineSpacingUpdate(float value) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
_style.LineSpacing = Mathf.Lerp(_lineSpacingRange.x, _lineSpacingRange.y, value);
|
||||
|
||||
UpdateLineSpacing();
|
||||
OnValueChanged();
|
||||
}
|
||||
|
||||
public void OnLineSpacingUpdate(string text) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
if (float.TryParse(text, out var value)) {
|
||||
_style.LineSpacing = Mathf.Clamp(value, _lineSpacingRange.x, _lineSpacingRange.y);
|
||||
}
|
||||
else {
|
||||
Logging.LogWarning("Line spacing value '{text}' could not be parsed.");
|
||||
}
|
||||
|
||||
UpdateLineSpacing();
|
||||
OnValueChanged();
|
||||
}
|
||||
|
||||
public void OnCharSpacingUpdate(float value) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
_style.CharSpacing = Mathf.Lerp(_charSpacingRange.x, _charSpacingRange.y, value);
|
||||
|
||||
UpdateCharSpacing();
|
||||
OnValueChanged();
|
||||
}
|
||||
|
||||
public void OnCharSpacingUpdate(string text) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
if (float.TryParse(text, out var value)) {
|
||||
_style.CharSpacing = Mathf.Clamp(value, _charSpacingRange.x, _charSpacingRange.y);
|
||||
}
|
||||
else {
|
||||
Logging.LogWarning("Char spacing value '{text}' could not be parsed.");
|
||||
}
|
||||
|
||||
UpdateCharSpacing();
|
||||
OnValueChanged();
|
||||
}
|
||||
|
||||
public void OnBoldUpdate(bool state) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
_style.Bold = state;
|
||||
_textBoxTMP.textComponent.fontStyle = _style.FontStyle | _font.FontStyle & ~_font.FontStyleMask;
|
||||
OnValueChanged();
|
||||
}
|
||||
|
||||
public void OnItalicUpdate(bool state) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
_style.Italic = state;
|
||||
_textBoxTMP.textComponent.fontStyle = _style.FontStyle | _font.FontStyle & ~_font.FontStyleMask;
|
||||
OnValueChanged();
|
||||
}
|
||||
|
||||
public void OnUnderlineUpdate(bool state) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
_style.Underline = state;
|
||||
_textBoxTMP.textComponent.fontStyle = _style.FontStyle | _font.FontStyle & ~_font.FontStyleMask;
|
||||
OnValueChanged();
|
||||
}
|
||||
|
||||
public void OnSmallCapsUpdate(bool state) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
_style.SmallCaps = state;
|
||||
_textBoxTMP.textComponent.fontStyle = _style.FontStyle | _font.FontStyle & ~_font.FontStyleMask;
|
||||
OnValueChanged();
|
||||
}
|
||||
|
||||
public void OnVerticalUpdate(bool state) {
|
||||
if (_ignoreUpdates) return;
|
||||
|
||||
_style.Vertical = state;
|
||||
OnValueChanged();
|
||||
}
|
||||
|
||||
|
||||
private void Start() {
|
||||
_textBoxTMP = ((TMP_InputField) _textBox);
|
||||
_textBoxTMP.text = _text;
|
||||
_textBoxTMP.textComponent.fontStyle = _style.FontStyle | _font.FontStyle & ~_font.FontStyleMask;
|
||||
_textBoxTMP.fontAsset = _font.FontAsset;
|
||||
|
||||
_font.SetupSample(_fontButton.GetComponentInChildren<TextMeshProUGUI>());
|
||||
|
||||
UpdateStyleButtons();
|
||||
UpdateLineSpacing();
|
||||
UpdateCharSpacing();
|
||||
}
|
||||
|
||||
private void OnValueChanged() {
|
||||
onValueChanged.Invoke(_text, _font, _style);
|
||||
}
|
||||
|
||||
private void UpdateStyleButtons() {
|
||||
_ignoreUpdates = true;
|
||||
|
||||
if (_font.Bold) {
|
||||
_boldButton.interactable = false;
|
||||
_boldButton.isOn = true;
|
||||
}
|
||||
else if (_font.BoldMask) {
|
||||
_boldButton.interactable = false;
|
||||
_boldButton.isOn = false;
|
||||
}
|
||||
else {
|
||||
_boldButton.interactable = true;
|
||||
_boldButton.isOn = _style.Bold;
|
||||
}
|
||||
|
||||
if (_font.Italic) {
|
||||
_italicButton.interactable = false;
|
||||
_italicButton.isOn = true;
|
||||
}
|
||||
else if (_font.ItalicMask) {
|
||||
_italicButton.interactable = false;
|
||||
_italicButton.isOn = false;
|
||||
}
|
||||
else {
|
||||
_italicButton.interactable = true;
|
||||
_italicButton.isOn = _style.Italic;
|
||||
}
|
||||
|
||||
if (_font.Underline) {
|
||||
_underlineButton.interactable = false;
|
||||
_underlineButton.isOn = true;
|
||||
}
|
||||
else if (_font.UnderlineMask) {
|
||||
_underlineButton.interactable = false;
|
||||
_underlineButton.isOn = false;
|
||||
}
|
||||
else {
|
||||
_underlineButton.interactable = true;
|
||||
_underlineButton.isOn = _style.Underline;
|
||||
}
|
||||
|
||||
if (_font.SmallCaps) {
|
||||
_smallCapsButton.interactable = false;
|
||||
_smallCapsButton.isOn = true;
|
||||
}
|
||||
else if (_font.SmallCapsMask) {
|
||||
_smallCapsButton.interactable = false;
|
||||
_smallCapsButton.isOn = false;
|
||||
}
|
||||
else {
|
||||
_smallCapsButton.interactable = true;
|
||||
_smallCapsButton.isOn = _style.SmallCaps;
|
||||
}
|
||||
|
||||
_verticalButton.isOn = _style.Vertical;
|
||||
|
||||
_ignoreUpdates = false;
|
||||
}
|
||||
|
||||
private void UpdateLineSpacing() {
|
||||
_ignoreUpdates = true;
|
||||
|
||||
_lineSpacingSlider.value = Mathf.InverseLerp(_lineSpacingRange.x, _lineSpacingRange.y, _style.LineSpacing);
|
||||
((TMP_InputField) _lineSpacingTextBox).text = $"{_style.LineSpacing:F1}";
|
||||
|
||||
_ignoreUpdates = false;
|
||||
}
|
||||
|
||||
private void UpdateCharSpacing() {
|
||||
_ignoreUpdates = true;
|
||||
|
||||
_charSpacingSlider.value = Mathf.InverseLerp(_charSpacingRange.x, _charSpacingRange.y, _style.CharSpacing);
|
||||
((TMP_InputField) _charSpacingTextBox).text = $"{_style.CharSpacing:F1}";
|
||||
|
||||
_ignoreUpdates = false;
|
||||
}
|
||||
}
|
||||
}
|
139
Source/ConformalDecals/UI/UILoader.cs
Normal file
139
Source/ConformalDecals/UI/UILoader.cs
Normal file
@ -0,0 +1,139 @@
|
||||
using TMPro;
|
||||
using UnityEngine;
|
||||
using UnityEngine.UI;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
[KSPAddon(KSPAddon.Startup.Instantly, true)]
|
||||
public class UILoader : MonoBehaviour {
|
||||
private static readonly string Path = KSPUtil.ApplicationRootPath + "GameData/ConformalDecals/Resources/";
|
||||
|
||||
private static GameObject _textEntryPrefab;
|
||||
private static GameObject _fontMenuPrefab;
|
||||
private static GameObject _colorPickerPrefab;
|
||||
|
||||
public static GameObject FontMenuPrefab => _fontMenuPrefab;
|
||||
public static GameObject TextEntryPrefab => _textEntryPrefab;
|
||||
public static GameObject ColorPickerPrefab => _colorPickerPrefab;
|
||||
|
||||
private void Awake() {
|
||||
var prefabs = AssetBundle.LoadFromFile(Path + "ui.conformaldecals");
|
||||
|
||||
_textEntryPrefab = prefabs.LoadAsset("TextEntryPanel") as GameObject;
|
||||
_fontMenuPrefab = prefabs.LoadAsset("FontMenuPanel") as GameObject;
|
||||
_colorPickerPrefab = prefabs.LoadAsset("ColorPickerPanel") as GameObject;
|
||||
|
||||
ProcessWindow(_textEntryPrefab);
|
||||
ProcessWindow(_fontMenuPrefab);
|
||||
ProcessWindow(_colorPickerPrefab);
|
||||
}
|
||||
|
||||
private static void ProcessWindow(GameObject window) {
|
||||
var skin = UISkinManager.defaultSkin;
|
||||
var font = UISkinManager.TMPFont;
|
||||
|
||||
var background = window.GetComponent<Image>();
|
||||
background.sprite = skin.window.normal.background;
|
||||
background.type = Image.Type.Sliced;
|
||||
|
||||
var texts = window.GetComponentsInChildren<TextMeshProUGUI>(true);
|
||||
|
||||
foreach (var text in texts) {
|
||||
ProcessText(text, font, Color.white);
|
||||
}
|
||||
|
||||
var tags = window.GetComponentsInChildren<UITag>(true);
|
||||
|
||||
foreach (var tag in tags) {
|
||||
switch (tag.type) {
|
||||
case UITag.UIType.Window:
|
||||
ProcessImage(tag.gameObject, skin.window);
|
||||
break;
|
||||
case UITag.UIType.Button:
|
||||
ProcessSelectable(tag.gameObject, skin.button);
|
||||
break;
|
||||
case UITag.UIType.ButtonToggle:
|
||||
ProcessToggleButton(tag.gameObject, skin.button);
|
||||
break;
|
||||
case UITag.UIType.RadioToggle:
|
||||
ProcessSelectable(tag.gameObject, skin.toggle);
|
||||
break;
|
||||
case UITag.UIType.BoxSlider:
|
||||
case UITag.UIType.Slider:
|
||||
ProcessSlider(tag.gameObject, skin.horizontalSlider, skin.horizontalSliderThumb);
|
||||
break;
|
||||
case UITag.UIType.Box:
|
||||
ProcessSelectable(tag.gameObject, skin.box);
|
||||
break;
|
||||
case UITag.UIType.Dropdown:
|
||||
ProcessDropdown(tag.gameObject, skin.button, skin.window);
|
||||
break;
|
||||
case UITag.UIType.Label:
|
||||
ProcessText(tag.GetComponent<TextMeshProUGUI>(), font, new Color(0.718f, 0.996f, 0.000f, 1.000f), 14);
|
||||
break;
|
||||
case UITag.UIType.Header:
|
||||
ProcessText(tag.GetComponent<TextMeshProUGUI>(), font, new Color(0.718f, 0.996f, 0.000f, 1.000f), 16);
|
||||
break;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
private static void ProcessImage(GameObject gameObject, UIStyle style) {
|
||||
var image = gameObject.GetComponent<Image>();
|
||||
if (image != null) {
|
||||
ProcessImage(image, style.normal);
|
||||
}
|
||||
}
|
||||
|
||||
private static void ProcessImage(Image image, UIStyleState state) {
|
||||
image.sprite = state.background;
|
||||
image.color = Color.white;
|
||||
image.type = Image.Type.Sliced;
|
||||
}
|
||||
|
||||
private static void ProcessSelectable(GameObject gameObject, UIStyle style) {
|
||||
var selectable = gameObject.GetComponent<Selectable>();
|
||||
if (selectable == null) {
|
||||
ProcessImage(gameObject, style);
|
||||
}
|
||||
else {
|
||||
ProcessImage(selectable.image, style.normal);
|
||||
|
||||
selectable.transition = Selectable.Transition.SpriteSwap;
|
||||
|
||||
var state = selectable.spriteState;
|
||||
state.highlightedSprite = style.highlight.background;
|
||||
state.selectedSprite = style.highlight.background;
|
||||
state.pressedSprite = style.active.background;
|
||||
state.disabledSprite = style.disabled.background;
|
||||
selectable.spriteState = state;
|
||||
}
|
||||
}
|
||||
|
||||
private static void ProcessToggleButton(GameObject gameObject, UIStyle style) {
|
||||
ProcessSelectable(gameObject, style);
|
||||
|
||||
var toggle = gameObject.GetComponent<Toggle>();
|
||||
if (toggle != null) ProcessImage(toggle.graphic as Image, style.active);
|
||||
}
|
||||
|
||||
private static void ProcessSlider(GameObject gameObject, UIStyle backgroundStyle, UIStyle thumbStyle) {
|
||||
ProcessSelectable(gameObject, thumbStyle);
|
||||
|
||||
var background = gameObject.transform.Find("Background");
|
||||
if (background != null) ProcessImage(background.gameObject, backgroundStyle);
|
||||
}
|
||||
|
||||
private static void ProcessDropdown(GameObject gameObject, UIStyle buttonStyle, UIStyle windowStyle) {
|
||||
ProcessSelectable(gameObject, buttonStyle);
|
||||
|
||||
var template = gameObject.transform.Find("Template").gameObject;
|
||||
if (template != null) ProcessImage(template, windowStyle);
|
||||
}
|
||||
|
||||
private static void ProcessText(TextMeshProUGUI text, TMP_FontAsset font, Color color, int size = -1) {
|
||||
text.font = font;
|
||||
text.color = color;
|
||||
if (size > 0) text.fontSize = size;
|
||||
}
|
||||
}
|
||||
}
|
21
Source/ConformalDecals/UI/UITag.cs
Normal file
21
Source/ConformalDecals/UI/UITag.cs
Normal file
@ -0,0 +1,21 @@
|
||||
using UnityEngine;
|
||||
|
||||
namespace ConformalDecals.UI {
|
||||
public class UITag : MonoBehaviour {
|
||||
public enum UIType {
|
||||
None,
|
||||
Window,
|
||||
Box,
|
||||
Button,
|
||||
ButtonToggle,
|
||||
RadioToggle,
|
||||
Slider,
|
||||
Dropdown,
|
||||
Label,
|
||||
Header,
|
||||
BoxSlider
|
||||
}
|
||||
|
||||
[SerializeField] public UIType type = UIType.None;
|
||||
}
|
||||
}
|
161
Source/ConformalDecals/Util/ColorHSL.cs
Normal file
161
Source/ConformalDecals/Util/ColorHSL.cs
Normal file
@ -0,0 +1,161 @@
|
||||
using System;
|
||||
using System.Globalization;
|
||||
using UnityEngine;
|
||||
|
||||
namespace ConformalDecals.Util {
|
||||
public struct ColorHSL : IEquatable<Color> {
|
||||
public float h;
|
||||
public float s;
|
||||
public float l;
|
||||
public float a;
|
||||
|
||||
public ColorHSL(float h, float s = 1, float l = 0.5f, float a = 1) {
|
||||
this.h = h;
|
||||
this.s = s;
|
||||
this.l = l;
|
||||
this.a = a;
|
||||
}
|
||||
|
||||
public override string ToString() {
|
||||
return $"HSLA({this.h:F3}, {this.s:F3}, {this.l:F3}, {this.a:F3})";
|
||||
}
|
||||
|
||||
public string ToString(string format) {
|
||||
return
|
||||
"HSLA(" +
|
||||
$"{this.h.ToString(format, CultureInfo.InvariantCulture.NumberFormat)}, " +
|
||||
$"{this.s.ToString(format, CultureInfo.InvariantCulture.NumberFormat)}, " +
|
||||
$"{this.l.ToString(format, CultureInfo.InvariantCulture.NumberFormat)}, " +
|
||||
$"{this.a.ToString(format, CultureInfo.InvariantCulture.NumberFormat)})";
|
||||
}
|
||||
|
||||
public bool Equals(ColorHSL other) {
|
||||
return (this.h.Equals(other.h) && this.s.Equals(other.s) && this.l.Equals(other.l) && this.a.Equals(other.a));
|
||||
}
|
||||
|
||||
public bool Equals(Color other) {
|
||||
var rgb = HSL2RGB(this);
|
||||
return rgb.Equals(other);
|
||||
}
|
||||
|
||||
public override bool Equals(object obj) {
|
||||
if (obj is ColorHSL otherHSL) return Equals(otherHSL);
|
||||
if (obj is Color otherRGB) return Equals(otherRGB);
|
||||
|
||||
return false;
|
||||
}
|
||||
|
||||
public override int GetHashCode() {
|
||||
return ((Vector4) this).GetHashCode();
|
||||
}
|
||||
|
||||
public float this[int index] {
|
||||
get {
|
||||
switch (index) {
|
||||
case 0:
|
||||
return this.h;
|
||||
case 1:
|
||||
return this.s;
|
||||
case 2:
|
||||
return this.l;
|
||||
case 3:
|
||||
return this.a;
|
||||
default:
|
||||
throw new IndexOutOfRangeException("Invalid Vector3 index!");
|
||||
}
|
||||
}
|
||||
set {
|
||||
switch (index) {
|
||||
case 0:
|
||||
this.h = value;
|
||||
break;
|
||||
case 1:
|
||||
this.s = value;
|
||||
break;
|
||||
case 2:
|
||||
this.l = value;
|
||||
break;
|
||||
case 3:
|
||||
this.a = value;
|
||||
break;
|
||||
default:
|
||||
throw new IndexOutOfRangeException("Invalid Vector3 index!");
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
public static bool operator ==(ColorHSL lhs, ColorHSL rhs) {
|
||||
return lhs.Equals(rhs);
|
||||
}
|
||||
|
||||
public static bool operator !=(ColorHSL lhs, ColorHSL rhs) {
|
||||
return !(lhs == rhs);
|
||||
}
|
||||
|
||||
public static implicit operator Vector4(ColorHSL c) {
|
||||
return new Vector4(c.h, c.s, c.l, c.a);
|
||||
}
|
||||
|
||||
public static implicit operator ColorHSL(Vector4 v) {
|
||||
return new ColorHSL(v.x, v.y, v.z, v.w);
|
||||
}
|
||||
|
||||
public static implicit operator ColorHSL(Color rgb) {
|
||||
return RGB2HSL(rgb);
|
||||
}
|
||||
|
||||
public static implicit operator Color(ColorHSL hsl) {
|
||||
return HSL2RGB(hsl);
|
||||
}
|
||||
|
||||
public static Color HSL2RGB(ColorHSL hsl) {
|
||||
float a = hsl.s * Mathf.Min(hsl.l, 1 - hsl.l);
|
||||
|
||||
float Component(int n) {
|
||||
float k = (n + hsl.h * 12) % 12;
|
||||
return hsl.l - a * Mathf.Max(-1, Mathf.Min(k - 3, Mathf.Min(9 - k, 1)));
|
||||
}
|
||||
|
||||
return new Color(Component(0), Component(8), Component(4), hsl.a);
|
||||
}
|
||||
|
||||
public static ColorHSL RGB2HSL(Color rgb) {
|
||||
float h = 0;
|
||||
float s = 0;
|
||||
float l = 0;
|
||||
|
||||
if (rgb.r >= rgb.g && rgb.r >= rgb.b) {
|
||||
float xMin = Mathf.Min(rgb.g, rgb.b);
|
||||
|
||||
l = (rgb.r + xMin) / 2;
|
||||
s = (rgb.r - l) / Mathf.Min(l, 1 - l);
|
||||
|
||||
float c = rgb.r - xMin;
|
||||
if (c > Mathf.Epsilon) h = (rgb.g - rgb.b) / (6 * c);
|
||||
|
||||
}
|
||||
else if (rgb.g >= rgb.r && rgb.g >= rgb.b) {
|
||||
float xMin = Mathf.Min(rgb.r, rgb.b);
|
||||
|
||||
l = (rgb.g + xMin) / 2;
|
||||
s = (rgb.g - l) / Mathf.Min(l, 1 - l);
|
||||
|
||||
float c = rgb.g - xMin;
|
||||
if (c > Mathf.Epsilon) h = (2 + ((rgb.b - rgb.r) / c)) / 6;
|
||||
|
||||
}
|
||||
else if (rgb.b >= rgb.r && rgb.b >= rgb.g) {
|
||||
float xMin = Mathf.Min(rgb.r, rgb.g);
|
||||
|
||||
l = (rgb.b + xMin) / 2;
|
||||
s = (rgb.b - l) / Mathf.Min(l, 1 - l);
|
||||
|
||||
float c = rgb.g - xMin;
|
||||
if (c > Mathf.Epsilon) h = (4 + ((rgb.r - rgb.g) / c)) / 6;
|
||||
|
||||
}
|
||||
|
||||
return new ColorHSL(h, s, l, rgb.a);
|
||||
}
|
||||
}
|
||||
}
|
123
Source/ConformalDecals/Util/ColorHSV.cs
Normal file
123
Source/ConformalDecals/Util/ColorHSV.cs
Normal file
@ -0,0 +1,123 @@
|
||||
using System;
|
||||
using System.Globalization;
|
||||
using UnityEngine;
|
||||
|
||||
namespace ConformalDecals.Util {
|
||||
public struct ColorHSV : IEquatable<Color> {
|
||||
public float h;
|
||||
public float s;
|
||||
public float v;
|
||||
public float a;
|
||||
|
||||
public ColorHSV(float h, float s = 1, float v = 1, float a = 1) {
|
||||
this.h = h;
|
||||
this.s = s;
|
||||
this.v = v;
|
||||
this.a = a;
|
||||
}
|
||||
|
||||
public override string ToString() {
|
||||
return $"HSVA({this.h:F3}, {this.s:F3}, {this.v:F3}, {this.a:F3})";
|
||||
}
|
||||
|
||||
public string ToString(string format) {
|
||||
return
|
||||
"HSVA(" +
|
||||
$"{this.h.ToString(format, CultureInfo.InvariantCulture.NumberFormat)}, " +
|
||||
$"{this.s.ToString(format, CultureInfo.InvariantCulture.NumberFormat)}, " +
|
||||
$"{this.v.ToString(format, CultureInfo.InvariantCulture.NumberFormat)}, " +
|
||||
$"{this.a.ToString(format, CultureInfo.InvariantCulture.NumberFormat)})";
|
||||
}
|
||||
|
||||
public bool Equals(ColorHSL other) {
|
||||
return (this.h.Equals(other.h) && this.s.Equals(other.s) && this.v.Equals(other.l) && this.a.Equals(other.a));
|
||||
}
|
||||
|
||||
public bool Equals(Color other) {
|
||||
var rgb = HSV2RGB(this);
|
||||
return rgb.Equals(other);
|
||||
}
|
||||
|
||||
public override bool Equals(object obj) {
|
||||
if (obj is ColorHSL otherHSL) return Equals(otherHSL);
|
||||
if (obj is Color otherRGB) return Equals(otherRGB);
|
||||
|
||||
return false;
|
||||
}
|
||||
|
||||
public override int GetHashCode() {
|
||||
return ((Vector4) this).GetHashCode();
|
||||
}
|
||||
|
||||
public float this[int index] {
|
||||
get {
|
||||
switch (index) {
|
||||
case 0:
|
||||
return this.h;
|
||||
case 1:
|
||||
return this.s;
|
||||
case 2:
|
||||
return this.v;
|
||||
case 3:
|
||||
return this.a;
|
||||
default:
|
||||
throw new IndexOutOfRangeException("Invalid Vector3 index!");
|
||||
}
|
||||
}
|
||||
set {
|
||||
switch (index) {
|
||||
case 0:
|
||||
this.h = value;
|
||||
break;
|
||||
case 1:
|
||||
this.s = value;
|
||||
break;
|
||||
case 2:
|
||||
this.v = value;
|
||||
break;
|
||||
case 3:
|
||||
this.a = value;
|
||||
break;
|
||||
default:
|
||||
throw new IndexOutOfRangeException("Invalid Vector3 index!");
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
public static bool operator ==(ColorHSV lhs, ColorHSV rhs) {
|
||||
return lhs.Equals(rhs);
|
||||
}
|
||||
|
||||
public static bool operator !=(ColorHSV lhs, ColorHSV rhs) {
|
||||
return !(lhs == rhs);
|
||||
}
|
||||
|
||||
public static implicit operator Vector4(ColorHSV c) {
|
||||
return new Vector4(c.h, c.s, c.v, c.a);
|
||||
}
|
||||
|
||||
public static implicit operator ColorHSV(Vector4 v) {
|
||||
return new ColorHSV(v.x, v.y, v.z, v.w);
|
||||
}
|
||||
|
||||
public static implicit operator ColorHSV(Color rgb) {
|
||||
return RGB2HSV(rgb);
|
||||
}
|
||||
|
||||
public static implicit operator Color(ColorHSV hsv) {
|
||||
return HSV2RGB(hsv);
|
||||
}
|
||||
|
||||
public static Color HSV2RGB(ColorHSV hsv) {
|
||||
var rgb = Color.HSVToRGB(hsv.h, hsv.s, hsv.v, false);
|
||||
rgb.a = hsv.a;
|
||||
return rgb;
|
||||
}
|
||||
|
||||
public static ColorHSV RGB2HSV(Color rgb) {
|
||||
var hsv = new ColorHSV {a = rgb.a};
|
||||
Color.RGBToHSV(rgb, out hsv.h, out hsv.s, out hsv.v);
|
||||
return hsv;
|
||||
}
|
||||
}
|
||||
}
|
22
Source/ConformalDecals/Util/ColorUtil.cs
Normal file
22
Source/ConformalDecals/Util/ColorUtil.cs
Normal file
@ -0,0 +1,22 @@
|
||||
using UnityEngine;
|
||||
|
||||
namespace ConformalDecals.Util {
|
||||
public static class ColorUtil {
|
||||
/// Returns an RGBA 32-bit hex string
|
||||
public static string ToHexString(this Color32 color) {
|
||||
return $"#{color.r:x2}{color.g:x2}{color.b:x2}{color.a:x2}";
|
||||
}
|
||||
|
||||
// Returns an RGBA 32-bit unsigned integer representation of the color
|
||||
public static uint ToUint(this Color32 color) {
|
||||
uint rgba = color.r;
|
||||
rgba <<= 8;
|
||||
rgba |= color.g;
|
||||
rgba <<= 8;
|
||||
rgba |= color.b;
|
||||
rgba <<= 8;
|
||||
rgba |= color.a;
|
||||
return rgba;
|
||||
}
|
||||
}
|
||||
}
|
@ -3,18 +3,23 @@ using UnityEngine;
|
||||
|
||||
namespace ConformalDecals.Util {
|
||||
public static class Logging {
|
||||
public static void Log(string message) => Debug.Log("[ConformalDecals] " + message);
|
||||
|
||||
public static void Log(this PartModule module, string message) => Debug.Log(FormatMessage(module, message));
|
||||
|
||||
public static void LogWarning(this PartModule module, string message) =>
|
||||
Debug.LogWarning(FormatMessage(module, message));
|
||||
public static void LogWarning(string message) => Debug.LogWarning("[ConformalDecals] " + message);
|
||||
|
||||
public static void LogError(this PartModule module, string message) =>
|
||||
Debug.LogError(FormatMessage(module, message));
|
||||
public static void LogWarning(this PartModule module, string message) => Debug.LogWarning(FormatMessage(module, message));
|
||||
|
||||
public static void LogError(string message) => Debug.LogError("[ConformalDecals] " + message);
|
||||
|
||||
public static void LogError(this PartModule module, string message) => Debug.LogError(FormatMessage(module, message));
|
||||
|
||||
public static void LogException(string message, Exception exception) => Debug.LogException(new Exception("[ConformalDecals] " + message, exception));
|
||||
|
||||
public static void LogException(this PartModule module, string message, Exception exception) =>
|
||||
Debug.LogException(new Exception(FormatMessage(module, message), exception));
|
||||
|
||||
|
||||
private static string FormatMessage(PartModule module, string message) =>
|
||||
$"[{GetPartName(module.part)} {module.GetType()}] {message}";
|
||||
|
||||
|
@ -139,44 +139,53 @@ namespace ConformalDecals.Util {
|
||||
throw new FormatException($"Improperly formatted {typeof(T)} value for {valueName} : '{valueString}");
|
||||
}
|
||||
|
||||
public static bool TryParseHexColor(string valueString, out Color32 value) {
|
||||
value = new Color32(0, 0, 0, byte.MaxValue);
|
||||
|
||||
if (!uint.TryParse(valueString, System.Globalization.NumberStyles.HexNumber, null, out var hexColor)) return false;
|
||||
|
||||
switch (valueString.Length) {
|
||||
case 8: // RRGGBBAA
|
||||
value.a = (byte) (hexColor & 0xFF);
|
||||
hexColor >>= 8;
|
||||
goto case 6;
|
||||
|
||||
case 6: // RRGGBB
|
||||
value.b = (byte) (hexColor & 0xFF);
|
||||
hexColor >>= 8;
|
||||
value.g = (byte) (hexColor & 0xFF);
|
||||
hexColor >>= 8;
|
||||
value.r = (byte) (hexColor & 0xFF);
|
||||
return true;
|
||||
|
||||
case 4: // RGBA
|
||||
value.a = (byte) ((hexColor & 0xF) << 4);
|
||||
hexColor >>= 4;
|
||||
goto case 3;
|
||||
|
||||
case 3: // RGB
|
||||
value.b = (byte) (hexColor & 0xF << 4);
|
||||
hexColor >>= 4;
|
||||
value.g = (byte) (hexColor & 0xF << 4);
|
||||
hexColor >>= 4;
|
||||
value.r = (byte) (hexColor & 0xF << 4);
|
||||
return true;
|
||||
|
||||
default:
|
||||
return false;
|
||||
}
|
||||
}
|
||||
|
||||
public static bool TryParseColor32(string valueString, out Color32 value) {
|
||||
value = new Color32(0, 0, 0, byte.MaxValue);
|
||||
|
||||
// HTML-style hex color
|
||||
// hex color
|
||||
if (valueString[0] == '#') {
|
||||
var hexColorString = valueString.Substring(1);
|
||||
|
||||
if (!int.TryParse(hexColorString, System.Globalization.NumberStyles.HexNumber, null, out var hexColor)) return false;
|
||||
|
||||
switch (hexColorString.Length) {
|
||||
case 8: // RRGGBBAA
|
||||
value.a = (byte) (hexColor & 0xFF);
|
||||
hexColor >>= 8;
|
||||
goto case 6;
|
||||
|
||||
case 6: // RRGGBB
|
||||
value.b = (byte) (hexColor & 0xFF);
|
||||
hexColor >>= 8;
|
||||
value.g = (byte) (hexColor & 0xFF);
|
||||
hexColor >>= 8;
|
||||
value.r = (byte) (hexColor & 0xFF);
|
||||
return true;
|
||||
|
||||
case 4: // RGBA
|
||||
value.a = (byte) ((hexColor & 0xF) << 4);
|
||||
hexColor >>= 4;
|
||||
goto case 3;
|
||||
|
||||
case 3: // RGB
|
||||
value.b = (byte) (hexColor & 0xF << 4);
|
||||
hexColor >>= 4;
|
||||
value.g = (byte) (hexColor & 0xF << 4);
|
||||
hexColor >>= 4;
|
||||
value.r = (byte) (hexColor & 0xF << 4);
|
||||
return true;
|
||||
|
||||
default:
|
||||
return false;
|
||||
if (TryParseHexColor(hexColorString, out var hexColor)) {
|
||||
value = hexColor;
|
||||
return true;
|
||||
}
|
||||
}
|
||||
|
||||
@ -207,7 +216,14 @@ namespace ConformalDecals.Util {
|
||||
value.g = (byte) (green * 0xFF);
|
||||
value.b = (byte) (blue * 0xFF);
|
||||
return true;
|
||||
|
||||
case 1: // try again for hex color
|
||||
if (TryParseHexColor(split[0], out var hexcolor)) {
|
||||
value = hexcolor;
|
||||
return true;
|
||||
}
|
||||
else {
|
||||
return false;
|
||||
}
|
||||
default:
|
||||
return false;
|
||||
}
|
||||
|
@ -1,3 +1,18 @@
|
||||
v0.2.0
|
||||
------
|
||||
- New Parts:
|
||||
- CDL-3 Surface Base Decal: A set of conformal decals based on the symbols from the movie Moon designed by Gavin Rothery
|
||||
- CDL-T Custom Text Decal: A customizable text decal with a variety of fonts
|
||||
- Changes:
|
||||
- New "KEYWORD" material modifier, allowing for shader features to be enabled and disabled.
|
||||
- material modifiers can now be removed in variants by setting `remove = true` inside them.
|
||||
- Unified all decal shaders into a single "Standard" shader with variants supporting any combination of bump, specular and emissive maps, plus SDF alphas.
|
||||
- Old shaders are remapped to Standard shader plus keywords automatically.
|
||||
- New SDF-based antialiasing for when decals extend to their borders, i.e. on opaque flags.
|
||||
- Fixes:
|
||||
- Fixed WIDTH and HEIGHT scale modes being flipped
|
||||
- Removed some debug log statements
|
||||
|
||||
v0.1.4
|
||||
------
|
||||
- Supported KSP versions: 1.8.x to 1.10.x
|
||||
|
Loading…
Reference in New Issue
Block a user